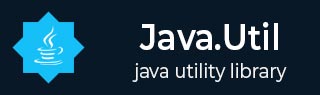
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包额外内容
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java TreeMap higherEntry() 方法
描述
Java TreeMap higherEntry(K key) 方法用于返回与严格大于给定键的最小键关联的键值映射,如果不存在这样的键,则返回 null。
声明
以下是 java.util.TreeMap.higherEntry() 方法的声明。
public Map.Entry<K,V> higherEntry(K key)
参数
key − 这是要匹配的键。
返回值
方法调用返回具有大于 key 的最小键的条目,如果不存在这样的键,则返回 null。
异常
ClassCastException − 如果指定的键无法与当前映射中的键进行比较,则抛出此异常。
NullPointerException − 如果指定的键为 null,并且此映射使用自然排序,或者其比较器不允许 null 键,则抛出此异常。
基于给定键从 Integer, Integer 对的 TreeMap 获取更高条目示例
以下示例演示了 Java TreeMap higherEntry() 方法的使用,以获取与严格大于给定键的最小键关联的映射。我们创建了一个 Integer,Integer 的 TreeMap 对象。然后添加了一些条目,并使用 higherEntry() 打印给定键的相关映射。
package com.tutorialspoint; import java.util.TreeMap; public class TreeMapDemo { public static void main(String[] args) { // creating tree map TreeMap<Integer, Integer> treemap = new TreeMap<>(); // populating tree map treemap.put(2, 2); treemap.put(1, 1); treemap.put(3, 3); treemap.put(6, 6); treemap.put(5, 5); // getting higher entry for key 4 System.out.println("Checking values of the map"); System.out.println("Value is: "+ treemap.higherEntry(4)); } }
输出
让我们编译并运行上面的程序,这将产生以下结果。
Checking values of the map Value is: 5=5
基于给定键从 Integer, String 对的 TreeMap 获取更高条目示例
以下示例演示了 Java TreeMap higherEntry() 方法的使用,以获取与严格大于给定键的最小键关联的映射。我们创建了一个 Integer,String 对的 TreeMap 对象。然后添加了一些条目,并使用 higherEntry() 打印给定键的相关映射。
package com.tutorialspoint; import java.util.TreeMap; public class TreeMapDemo { public static void main(String[] args) { // creating tree map TreeMap<Integer, String> treemap = new TreeMap<>(); // populating tree map treemap.put(2, "two"); treemap.put(1, "one"); treemap.put(3, "three"); treemap.put(6, "six"); treemap.put(5, "five"); // getting higher entry for key 4 System.out.println("Checking values of the map"); System.out.println("Value is: "+ treemap.higherEntry(4)); } }
输出
让我们编译并运行上面的程序,这将产生以下结果。
Checking values of the map Value is: 5=five
基于给定键从 Integer, Object 对的 TreeMap 获取更高条目示例
以下示例演示了 Java TreeMap higherEntry() 方法的使用,以获取与严格大于给定键的最小键关联的映射。我们创建了一个 Integer,Student 对的 TreeMap 对象。然后添加了一些条目,并使用 higherEntry() 打印给定键的相关映射。
package com.tutorialspoint; import java.util.TreeMap; public class TreeMapDemo { public static void main(String[] args) { // creating tree map TreeMap<Integer, Student> treemap = new TreeMap<>(); // populating tree map treemap.put(2, new Student(2, "Robert")); treemap.put(1, new Student(1, "Julie")); treemap.put(3, new Student(3, "Adam")); treemap.put(6, new Student(5, "Julia")); treemap.put(5, new Student(5, "Tom")); // getting higher entry for key 4 System.out.println("Checking values of the map"); System.out.println("Value is: "+ treemap.higherEntry(4)); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } @Override public boolean equals(Object obj) { if(obj == null) return false; Student s = (Student)obj; return this.rollNo == s.rollNo && this.name.equalsIgnoreCase(s.name); } }
输出
让我们编译并运行上面的程序,这将产生以下结果。
Checking values of the map Value is: 5=[ 5, Tom ]