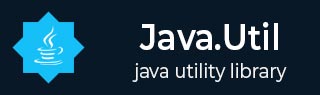
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包补充
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java TreeMap put() 方法
描述
Java TreeMap put(K key,V value) 方法用于在此映射中将指定的值与指定的键关联。如果映射先前包含该键的映射,则旧值将被替换。
声明
以下是java.util.TreeMap.put() 方法的声明。
public V put(K key,V value)
参数
key − 这是要与其关联指定值的键。
value − 这是要与指定键关联的值。
返回值
方法调用返回与键关联的上一个值,如果键没有映射,则返回 null。
异常
无
向 Integer,Integer 对的 TreeMap 添加键值对示例
以下示例演示了 Java TreeMap put() 方法的使用,用于在此映射中添加/更新键值映射。我们创建了一个 Integer,Integer 对的 TreeMap 对象。然后使用 put() 方法添加了一些条目,打印映射,然后使用 put() 更新键的值,并再次打印映射以查看更新条目的效果。
package com.tutorialspoint; import java.util.TreeMap; public class TreeMapDemo { public static void main(String[] args) { // creating tree map TreeMap<Integer, Integer> treemap = new TreeMap<>(); // populating tree map treemap.put(2, 2); treemap.put(1, 1); treemap.put(3, 3); treemap.put(6, 6); treemap.put(5, 5); // Putting value at key 3 System.out.println("Value before modification: "+ treemap); System.out.println("Value returned: "+ treemap.put(3,7)); System.out.println("Value after modification: "+ treemap); } }
输出
让我们编译并运行上述程序,这将产生以下结果。
Value before modification: {1=1, 2=2, 3=3, 5=5, 6=6} Value returned: 3 Value after modification: {1=1, 2=2, 3=7, 5=5, 6=6}
向 Integer,String 对的 TreeMap 添加键值对示例
以下示例演示了 Java TreeMap put() 方法的使用,用于在此映射中添加/更新键值映射。我们创建了一个 Integer,String 对的 TreeMap 对象。然后使用 put() 方法添加了一些条目,打印映射,然后使用 put() 更新键的值,并再次打印映射以查看更新条目的效果。
package com.tutorialspoint; import java.util.TreeMap; public class TreeMapDemo { public static void main(String[] args) { // creating tree map TreeMap<Integer, String> treemap = new TreeMap<>(); // populating tree map treemap.put(2, "two"); treemap.put(1, "one"); treemap.put(3, "three"); treemap.put(6, "six"); treemap.put(5, "five"); // Putting value at key 3 System.out.println("Value before modification: "+ treemap); System.out.println("Value returned: "+ treemap.put(3,"TP")); System.out.println("Value after modification: "+ treemap); } }
输出
让我们编译并运行上述程序,这将产生以下结果。
Value before modification: {1=one, 2=two, 3=three, 5=five, 6=six} Value returned: three Value after modification: {1=one, 2=two, 3=TP, 5=five, 6=six}
向 Integer,Object 对的 TreeMap 添加键值对示例
以下示例演示了 Java TreeMap put() 方法的使用,用于在此映射中添加/更新键值映射。我们创建了一个 Integer,Student 对的 TreeMap 对象。然后使用 put() 方法添加了一些条目,打印映射,然后使用 put() 更新键的值,并再次打印映射以查看更新条目的效果。
package com.tutorialspoint; import java.util.TreeMap; public class TreeMapDemo { public static void main(String[] args) { // creating tree map TreeMap<Integer, Student> treemap = new TreeMap<>(); // populating tree map treemap.put(2, new Student(2, "Robert")); treemap.put(1, new Student(1, "Julie")); treemap.put(3, new Student(3, "Adam")); treemap.put(6, new Student(6, "Julia")); treemap.put(5, new Student(5, "Tom")); // Putting value at key 3 System.out.println("Value before modification: "+ treemap); System.out.println("Value returned: "+ treemap.put(3, new Student(7, "Alfred"))); System.out.println("Value after modification: "+ treemap); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } @Override public boolean equals(Object obj) { if(obj == null) return false; Student s = (Student)obj; return this.rollNo == s.rollNo && this.name.equalsIgnoreCase(s.name); } }
输出
让我们编译并运行上述程序,这将产生以下结果。
Value before modification: {1=[ 1, Julie ], 2=[ 2, Robert ], 3=[ 3, Adam ], 5=[ 5, Tom ], 6=[ 6, Julia ]} Value returned: [ 3, Adam ] Value after modification: {1=[ 1, Julie ], 2=[ 2, Robert ], 3=[ 7, Alfred ], 5=[ 5, Tom ], 6=[ 6, Julia ]}
java_util_treemap.htm
广告