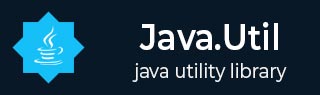
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包附加内容
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java TreeMap subMap() 方法
描述
Java TreeMap subMap(K fromKey,K toKey) 方法用于返回此映射的一部分的视图,其键的范围从 fromKey(包含)到 toKey(不包含)。(如果 fromKey 和 toKey 相等,则返回的映射为空。)返回的映射由此映射支持,因此返回的映射中的更改会反映在此映射中,反之亦然。
声明
以下是java.util.TreeMap.subMap() 方法的声明。
public SortedMap<K,V> subMap(K fromKey,K toKey)
参数
fromKey − 这是返回映射中键的低端点(包含)。
toKey − 这是返回映射中键的高端点(不包含)。
返回值
方法调用返回此映射的一部分的视图,其键的范围从 fromKey(包含)到 toKey(不包含)。
异常
ClassCastException − 如果无法使用此映射的比较器将 fromKey 和 toKey 相互比较,则抛出此异常。
NullPointerException − 如果 fromKey 或 toKey 为 null,并且此映射使用自然排序,或者其比较器不允许 null 键,则抛出此异常。
IllegalArgumentException − 如果 fromKey 大于 toKey;或者如果此映射本身具有受限范围,并且 fromKey 或 toKey 位于范围之外,则抛出此异常。
Java TreeMap subMap(K fromKey, boolean fromInclusive, K toKey, boolean toInclusive) 方法
描述
subMap(K fromKey, boolean fromInclusive, K toKey, boolean toInclusive) 方法用于返回此映射的一部分的视图,其键的范围从 fromKey 到 toKey。如果 fromKey 和 toKey 相等,则返回的映射为空,除非 fromExclusive 和 toExclusive 都为 true。返回的映射由此映射支持,因此返回的映射中的更改会反映在此映射中,反之亦然。
声明
以下是java.util.TreeMap.subMap() 方法的声明。
public NavigableMap<K,V> subMap(K fromKey, boolean fromInclusive, K toKey, boolean toInclusive)
参数
fromKey − 这是返回映射中键的低端点。
fromInclusive − 如果要将低端点包含在返回的视图中,则为 true。
toKey − 这是返回映射中键的高端点。
toInclusive − 如果要将高端点包含在返回的视图中,则为 true。
返回值
方法调用返回此映射的一部分的视图,其键的范围从 fromKey 到 toKey。
异常
ClassCastException − 如果无法使用此映射的比较器将 fromKey 和 toKey 相互比较,则抛出此异常。
NullPointerException − 如果 fromKey 或 toKey 为 null,并且此映射使用自然排序,或者其比较器不允许 null 键,则抛出此异常。
IllegalArgumentException − 如果 fromKey 大于 toKey;或者如果此映射本身具有受限范围,并且 fromKey 或 toKey 位于范围之外,则抛出此异常。
Integer,Integer 对的 TreeMap 的子映射示例
以下示例演示了如何使用 Java TreeMap subMap(K fromKey, K toKey) 方法获取此映射的子映射,其键的范围从 fromKey(包含)到 toKey(不包含)。我们创建了两个 Integer,Integer 对的 TreeMap 对象。然后将一些条目添加到第一个映射中,并使用 subMap() 从第一个映射中检索和打印子映射。
package com.tutorialspoint; import java.util.SortedMap; import java.util.TreeMap; public class TreeMapDemo { public static void main(String[] args) { // creating maps TreeMap<Integer, Integer> treemap = new TreeMap<>(); SortedMap<Integer, Integer> treemapincl = new TreeMap<>(); // populating tree map treemap.put(2, 2); treemap.put(1, 1); treemap.put(3, 3); treemap.put(6, 6); treemap.put(5, 5); System.out.println("Getting a portion of the map"); treemapincl = treemap.subMap(1,5); System.out.println("Sub map values: "+treemapincl); } }
输出
让我们编译并运行上述程序,这将产生以下结果。
Getting a portion of the map Sub map values: {1=1, 2=2, 3=3}
Integer,String 对的 TreeMap 的子映射示例
以下示例演示了如何使用 Java TreeMap subMap(K fromKey, K toKey) 方法获取此映射的子映射,其键的范围从 fromKey(包含)到 toKey(不包含)。我们创建了两个 Integer,String 对的 TreeMap 对象。然后将一些条目添加到第一个映射中,并使用 subMap() 从第一个映射中检索和打印子映射。
package com.tutorialspoint; import java.util.SortedMap; import java.util.TreeMap; public class TreeMapDemo { public static void main(String[] args) { // creating maps TreeMap<Integer, String> treemap = new TreeMap<>(); SortedMap<Integer, String> treemapincl = new TreeMap<>(); // populating tree map treemap.put(2, "two"); treemap.put(1, "one"); treemap.put(3, "three"); treemap.put(6, "six"); treemap.put(5, "five"); System.out.println("Getting a portion of the map"); treemapincl = treemap.subMap(1,5); System.out.println("Sub map values: "+treemapincl); } }
输出
让我们编译并运行上述程序,这将产生以下结果。
Getting a portion of the map Sub map values: {1=one, 2=two, 3=three}
Integer,Object 对的 TreeMap 的子映射示例
以下示例演示了如何使用 Java TreeMap subMap(K fromKey, boolean fromInclusive, K toKey, boolean toInclusive) 方法获取此映射的子映射,其键的范围从 fromKey 到 toKey,并对两个键都使用包含标志。我们创建了两个 Integer,String 对的 TreeMap 对象。然后将一些条目添加到第一个映射中,并使用 subMap() 从第一个映射中检索和打印子映射。
package com.tutorialspoint; import java.util.NavigableMap; import java.util.TreeMap; public class TreeMapDemo { public static void main(String[] args) { // creating maps TreeMap<Integer, String> treemap = new TreeMap<>(); NavigableMap<Integer, String> treemapincl = new TreeMap<>(); // populating tree map treemap.put(2, "two"); treemap.put(1, "one"); treemap.put(3, "three"); treemap.put(6, "six"); treemap.put(5, "five"); System.out.println("Getting a portion of the map"); treemapincl = treemap.subMap(1, true, 3, true); System.out.println("Sub map values: "+treemapincl); } }
输出
让我们编译并运行上述程序,这将产生以下结果。
Getting a portion of the map Sub map values: {1=one, 2=two, 3=three}