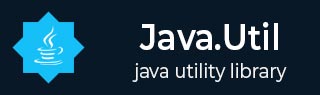
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包其他内容
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java TreeSet contains() 方法
描述
Java TreeSet contains(Object o) 方法用于返回 true,当且仅当此集合包含指定的元素。
声明
以下是 java.util.TreeSet.contains() 方法的声明。
public boolean contains(Object o)
参数
o − 要检查此集合中是否包含的对象。
返回值
如果此集合包含指定的元素,则方法调用返回 true。
异常
ClassCastException − 如果指定的元素无法与集合中当前存在的元素进行比较,则抛出此异常。
NullPointerException − 如果指定的元素为 null 且此集合使用自然排序,或者其比较器不允许 null 元素,则抛出此异常。
在 Integer 类型 TreeSet 中检查元素是否存在示例
以下示例演示了如何使用 Java TreeSet contains() 方法来检查元素是否出现在 TreeSet 中。我们创建了一个 Integer 类型的 TreeSet 对象。然后使用 add() 方法添加了一些条目,并在集合中检查一个元素,并打印结果。
package com.tutorialspoint; import java.util.TreeSet; public class TreeSetDemo { public static void main(String[] args) { // creating a TreeSet TreeSet <Integer>treeset = new TreeSet<>(); // adding in the tree set treeset.add(12); treeset.add(13); treeset.add(14); treeset.add(15); // check existence of 15 System.out.println("Checking existence of 15 "); System.out.println("Is 15 there in the set: "+treeset.contains(15)); } }
输出
让我们编译并运行以上程序,这将产生以下结果。
Checking existence of 15 Is 15 there in the set: true
在 String 类型 TreeSet 中检查元素是否存在示例
以下示例演示了如何使用 Java TreeSet contains() 方法来检查元素是否出现在 TreeSet 中。我们创建了一个 String 类型的 TreeSet 对象。然后使用 add() 方法添加了一些条目,并在集合中检查一个元素,并打印结果。
package com.tutorialspoint; import java.util.TreeSet; public class TreeSetDemo { public static void main(String[] args) { // creating a TreeSet TreeSet <String>treeset = new TreeSet<>(); // adding in the tree set treeset.add("12"); treeset.add("13"); treeset.add("14"); treeset.add("15"); // check existence of "15" System.out.println("Checking existence of 15 "); System.out.println("Is 15 there in the set: "+treeset.contains("15")); } }
输出
让我们编译并运行以上程序,这将产生以下结果。
Checking existence of 15 Is 15 there in the set: true
在 Object 类型 TreeSet 中检查元素是否存在示例
以下示例演示了如何使用 Java TreeSet contains() 方法来检查元素是否出现在 TreeSet 中。我们创建了一个 Student 对象类型的 TreeSet 对象。然后使用 add() 方法添加了一些条目,并在集合中检查一个元素,并打印结果。
package com.tutorialspoint; import java.util.TreeSet; public class TreeSetDemo { public static void main(String[] args) { // creating a TreeSet TreeSet <Student>treeset = new TreeSet<>(); // adding in the tree set treeset.add(new Student(1, "Robert")); treeset.add(new Student(2, "Julie")); treeset.add(new Student(3, "Adam")); treeset.add(new Student(4, "Julia")); // check existence of "Adam" System.out.println("Checking existence of 15 "); System.out.println("Is Adam there in the set: "+treeset.contains(new Student(3, "Adam"))); } } class Student implements Comparable<Student> { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } @Override public boolean equals(Object obj) { Student s = (Student)obj; return this.rollNo == s.rollNo && this.name.equalsIgnoreCase(s.name); } @Override public int compareTo(Student student) { return this.rollNo - student.rollNo; } }
输出
让我们编译并运行以上程序,这将产生以下结果。
Checking existence of 15 Is Adam there in the set: true
java_util_treeset.htm
广告