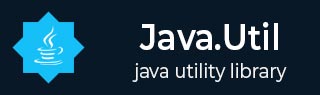
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包额外内容
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java Vector copyInto() 方法
描述
Java Vector copyInto(Object[] anArray) 方法用于将此向量的组件复制到指定的数组中。此向量中索引 k处的项目将复制到数组的组件 k中。这意味着元素在向量和数组中的位置相同。数组必须足够大以容纳此向量中的所有对象,否则将抛出IndexOutOfBoundsException异常。
声明
以下是java.util.Vector.copyInto()方法的声明
public void copyInto(Object[] anArray)
参数
anArray - 这是将组件复制到的数组。
返回值
返回类型为void,因此不返回任何内容。
异常
NullPointerException - 如果给定的数组为空。
将整数向量元素复制到数组示例
以下示例演示了如何使用 Java Vector copyInto() 方法将此向量的组件复制到指定的数组中。我们正在创建一个向量对象和一个 Integer 数组。然后将元素添加到向量和数组中。在使用 copyinto() 方法之前打印向量和数组的元素。然后使用 copyInto() 方法,将向量的元素复制到数组中,并再次打印更新后的数组以验证结果。
package com.tutorialspoint; import java.util.Vector; public class VectorDemo { public static void main(String[] args) { // create an empty Vector vec with an initial capacity of 4 Vector<Integer> vec = new Vector<>(4); Integer anArray[] = new Integer[4]; anArray[0] = 100; anArray[1] = 100; anArray[2] = 100; anArray[3] = 100; // use add() method to add elements in the vector vec.add(4); vec.add(3); vec.add(2); vec.add(1); // elements in the array before copy System.out.println("Elements in the array before copy"); for (Integer number : anArray) { System.out.println("Element = " + number); } // copy into the array vec.copyInto(anArray); // elements in the array after copy System.out.println("Elements in the array after copy"); for (Integer number : anArray) { System.out.println("Element = " + number); } } }
输出
让我们编译并运行上述程序,这将产生以下结果。
Elements in the array before copy Element = 100 Element = 100 Element = 100 Element = 100 Elements in the array after copy Element = 4 Element = 3 Element = 2 Element = 1
将字符串向量元素复制到数组示例
以下示例演示了如何使用 Java Vector copyInto() 方法将此向量的组件复制到指定的数组中。我们正在创建一个向量对象和一个 String 数组。然后将元素添加到向量和数组中。在使用 copyinto() 方法之前打印向量和数组的元素。然后使用 copyInto() 方法,将向量的元素复制到数组中,并再次打印更新后的数组以验证结果。
package com.tutorialspoint; import java.util.Vector; public class VectorDemo { public static void main(String[] args) { // create an empty Vector vec with an initial capacity of 4 Vector<String> vec = new Vector<>(4); String anArray[] = new String[4]; anArray[0] = "A"; anArray[1] = "A"; anArray[2] = "A"; anArray[3] = "A"; // use add() method to add elements in the vector vec.add("D"); vec.add("B"); vec.add("C"); vec.add("A"); // elements in the array before copy System.out.println("Elements in the array before copy"); for (String element : anArray) { System.out.println("Element = " + element); } // copy into the array vec.copyInto(anArray); // elements in the array after copy System.out.println("Elements in the array after copy"); for (String element : anArray) { System.out.println("Element = " + element); } } }
输出
让我们编译并运行上述程序,这将产生以下结果。
Elements in the array before copy Element = A Element = A Element = A Element = A Elements in the array after copy Element = D Element = C Element = B Element = A
将对象向量元素复制到数组示例
以下示例演示了如何使用 Java Vector copyInto() 方法将此向量的组件复制到指定的数组中。我们正在创建一个向量对象和一个 Student 数组。然后将元素添加到向量和数组中。在使用 copyinto() 方法之前打印向量和数组的元素。然后使用 copyInto() 方法,将向量的元素复制到数组中,并再次打印更新后的数组以验证结果。
package com.tutorialspoint; import java.util.Vector; public class VectorDemo { public static void main(String[] args) { // create an empty Vector vec with an initial capacity of 4 Vector<Student> vec = new Vector<>(4); Student anArray[] = new Student[4]; anArray[0] = new Student(1, "Julie"); anArray[1] = new Student(1, "Julie"); anArray[2] = new Student(1, "Julie"); anArray[3] = new Student(1, "Julie"); // use add() method to add elements in the vector vec.add(new Student(1, "Julie")); vec.add(new Student(2, "Robert")); vec.add(new Student(3, "Adam")); vec.add(new Student(4, "Jane")); // elements in the array before copy System.out.println("Elements in the array before copy"); for (Student element : anArray) { System.out.println("Element = " + element); } // copy into the array vec.copyInto(anArray); // elements in the array after copy System.out.println("Elements in the array after copy"); for (Student element : anArray) { System.out.println("Element = " + element); } } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } @Override public boolean equals(Object obj) { Student s = (Student)obj; return this.rollNo == s.rollNo && this.name.equalsIgnoreCase(s.name); } }
输出
让我们编译并运行上述程序,这将产生以下结果。
Elements in the array before copy Element = [ 1, Julie ] Element = [ 1, Julie ] Element = [ 1, Julie ] Element = [ 1, Julie ] Elements in the array after copy Element = [ 1, Julie ] Element = [ 2, Robert ] Element = [ 3, Adam ] Element = [ 4, Jane ]