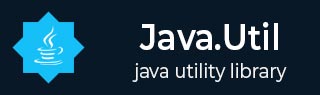
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包扩展
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java Vector hashCode() 方法
描述
Java Vector hashCode() 方法用于返回此向量的哈希码值。
声明
以下是 java.util.Vector.hashCode() 方法的声明
public int hashCode()
参数
无
返回值
方法调用返回此列表的哈希码值(int)。
异常
无
获取整数向量哈希码示例
以下示例演示了如何使用 Java Vector hashCode() 方法获取此向量的哈希码。我们使用 add() 方法调用每个元素将几个整数添加到 Vector 对象中,并使用 hashCode() 方法获取哈希码并打印它。
package com.tutorialspoint; import java.util.Vector; public class VectorDemo { public static void main(String[] args) { // create an empty Vector vec with an initial capacity of 4 Vector<Integer> vec = new Vector<>(4); // use add() method to add elements in the vector vec.add(4); vec.add(3); vec.add(2); vec.add(1); // let us get the hashcode of the vector System.out.println("Hash code: "+vec.hashCode()); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Hash code: 1045631
获取字符串向量哈希码示例
以下示例演示了如何使用 Java Vector hashCode() 方法获取此向量的哈希码。我们使用 add() 方法调用每个元素将几个字符串添加到 Vector 对象中,并使用 hashCode() 方法获取哈希码并打印它。
package com.tutorialspoint; import java.util.Vector; public class VectorDemo { public static void main(String[] args) { // create an empty Vector vec with an initial capacity of 4 Vector<String> vec = new Vector<>(4); // use add() method to add elements in the vector vec.add("Welcome"); vec.add("To"); vec.add("Tutorialspoint"); // let us get the hashcode of the vector System.out.println("Hash code: "+vec.hashCode()); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Hash code: -1700837919
获取对象向量哈希码示例
以下示例演示了如何使用 Java Vector hashCode() 方法获取此向量的哈希码。我们使用 add() 方法调用每个元素将几个 Student 对象添加到 Vector 对象中,并使用 hashCode() 方法获取哈希码并打印它。
package com.tutorialspoint; import java.util.Vector; public class VectorDemo { public static void main(String[] args) { // create an empty Vector vec with an initial capacity of 4 Vector<Student> vec = new Vector<>(4); // use add() method to add elements in the vector vec.add(new Student(1, "Julie")); vec.add(new Student(2, "Robert")); vec.add(new Student(3, "Adam")); // let us get the hashcode of the vector System.out.println("Hash code: "+vec.hashCode()); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Hash code: 368956094
java_util_vector.htm
广告