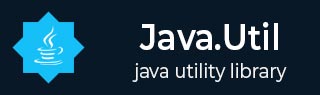
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包其他内容
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java Vector removeAllElements() 方法
描述
Java Vector removeAllElements() 方法用于移除此向量中的所有组件并将大小设置为零。此方法的功能与 clear 方法相同。
声明
以下是 java.util.Vector.removeAllElements() 方法的声明
public void removeAllElements()
参数
无
返回值
无
异常
无
移除整数向量所有元素的示例
以下示例演示了如何使用 Java Vector removeAllElements() 方法移除向量的所有元素。我们创建了一个整数向量,添加了一些元素,打印其大小,然后使用 removeAllElements() 方法移除所有元素。最后,我们打印了向量的新大小,以查看是否所有元素都被移除。
package com.tutorialspoint; import java.util.Vector; public class VectorDemo { public static void main(String[] args) { // create an empty Vector vec with an initial capacity of 4 Vector<Integer> vec = new Vector<>(4); // use add() method to add elements in the vector vec.add(4); vec.add(3); vec.add(2); vec.add(1); System.out.println("Size of the vector: "+vec.size()); System.out.println("Removing all elements"); // lets remove all the elements vec.removeAllElements(); System.out.println("Now size of the vector: "+vec.size()); } }
输出
让我们编译并运行以上程序,这将产生以下结果。
Size of the vector: 4 Removing all elements Now size of the vector: 0
移除字符串向量所有元素的示例
以下示例演示了如何使用 Java Vector removeAllElements() 方法移除向量的所有元素。我们创建了一个字符串向量,添加了一些元素,打印其大小,然后使用 removeAllElements() 方法移除所有元素。最后,我们打印了向量的新大小,以查看是否所有元素都被移除。
package com.tutorialspoint; import java.util.Vector; public class VectorDemo { public static void main(String[] args) { // create an empty Vector vec with an initial capacity of 4 Vector<String> vec = new Vector<>(4); // use add() method to add elements in the vector vec.add("D"); vec.add("C"); vec.add("B"); vec.add("A"); System.out.println("Size of the vector: "+vec.size()); System.out.println("Removing all elements"); // lets remove all the elements vec.removeAllElements(); System.out.println("Now size of the vector: "+vec.size()); } }
输出
让我们编译并运行以上程序,这将产生以下结果。
Size of the vector: 4 Removing all elements Now size of the vector: 0
移除学生向量所有元素的示例
以下示例演示了如何使用 Java Vector removeAllElements() 方法移除向量的所有元素。我们创建了一个学生对象向量,添加了一些元素,打印其大小,然后使用 removeAllElements() 方法移除所有元素。最后,我们打印了向量的新大小,以查看是否所有元素都被移除。
package com.tutorialspoint; import java.util.Vector; public class VectorDemo { public static void main(String[] args) { // create an empty Vector vec with an initial capacity of 4 Vector<Student> vec = new Vector<>(4); // use add() method to add elements in the vector vec.add(new Student(1, "Julie")); vec.add(new Student(2, "Robert")); vec.add(new Student(3, "Adam")); vec.add(new Student(4, "Jene")); System.out.println("Size of the vector: "+vec.size()); System.out.println("Removing all elements"); // lets remove all the elements vec.removeAllElements(); System.out.println("Now size of the vector: "+vec.size()); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } @Override public boolean equals(Object obj) { Student s = (Student)obj; return this.rollNo == s.rollNo && this.name.equalsIgnoreCase(s.name); } }
输出
让我们编译并运行以上程序,这将产生以下结果。
Size of the vector: 4 Removing all elements Now size of the vector: 0
java_util_vector.htm
广告