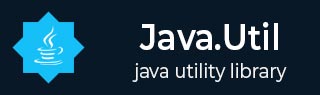
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包的其他内容
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java Vector size() 方法
描述
Java Vector size() 方法用于返回此向量中组件的数量。
声明
以下是 java.util.Vector.size() 方法的声明
public int size()
参数
无
返回值
方法调用返回此向量中组件的数量。
异常
无
获取整数向量大小的示例
以下示例演示了 Java Vector size() 方法的用法。我们使用 add() 方法为每个元素向 Vector 对象添加几个整数。使用 size() 方法打印向量的尺寸。并使用 remove(index) 方法移除一个元素,然后再次打印向量的尺寸。
package com.tutorialspoint; import java.util.Vector; public class VectorDemo { public static void main(String[] args) { // create an empty array list Vector<Integer> vector = new Vector<>(); // use add() method to add elements in the vector vector.add(20); vector.add(30); vector.add(20); vector.add(30); vector.add(15); vector.add(22); vector.add(11); // let us print the size of the vector again System.out.println("Vector Size = " + vector.size()); // remove an element at index 2 vector.remove(2); // let us print the size of the vector again System.out.println("Vector Size = " + vector.size()); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Vector Size = 7 Vector Size = 6
设置字符串向量大小的示例
以下示例演示了 Java Vector size() 方法的用法。我们使用 add() 方法为每个元素向 Vector 对象添加几个字符串。使用 size() 方法打印向量的尺寸。并使用 remove(index) 方法移除一个元素,然后再次打印向量的尺寸。
package com.tutorialspoint; import java.util.Vector; public class VectorDemo { public static void main(String[] args) { // create an empty array list Vector<String> vector = new Vector<>(); // use add() method to add elements in the vector vector.add("Welcome"); vector.add("To"); vector.add("Tutorialspoint"); // let us print the size of the vector again System.out.println("Vector Size = " + vector.size()); // remove an element at index 2 vector.remove(2); // let us print the size of the vector again System.out.println("Vector Size = " + vector.size()); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Vector Size = 3 Vector Size = 2
设置对象向量大小的示例
以下示例演示了 Java Vector size() 方法的用法。我们使用 add() 方法为每个元素向 Vector 对象添加几个 Student 对象。使用 size() 方法打印向量的尺寸。并使用 remove(index) 方法移除一个元素,然后再次打印向量的尺寸。
package com.tutorialspoint; import java.util.Vector; public class VectorDemo { public static void main(String[] args) { // create an empty vector Vector<Student> vector = new Vector<>(); // use add() method to add elements in the vector vector.add(new Student(1, "Julie")); vector.add(new Student(2, "Robert")); vector.add(new Student(3, "Adam")); // let us print the size of the vector again System.out.println("Vector Size = " + vector.size()); // remove an element at index 2 vector.remove(2); // let us print the size of the vector again System.out.println("Vector Size = " + vector.size()); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Vector Size = 3 Vector Size = 2
java_util_vector.htm
广告