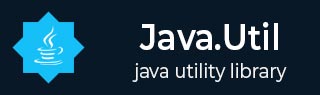
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包扩展
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java Vector trimToSize() 方法
描述
Java Vector trimToSize() 方法用于将此向量的容量调整为向量的当前大小。如果此向量的容量大于其当前大小,则容量将更改为当前大小。
声明
以下是java.util.Vector.trimToSize() 方法的声明
public void trimToSize()
参数
无
返回值
无
异常
无
将整数向量调整为指定大小的示例
以下示例演示了 Java Vector trimToSize() 方法的用法。我们使用 add() 方法为 Vector 对象添加几个整数,并打印向量的容量。然后,我们使用 trimToSize() 调整向量的大小,并再次打印其容量以反映更改。
package com.tutorialspoint; import java.util.Vector; public class VectorDemo { public static void main(String[] args) { // create an empty Vector vec with an initial capacity of 4 Vector<Integer> vec = new Vector<>(10); // use add() method to add elements in the vector vec.add(4); vec.add(3); vec.add(2); vec.add(1); // let us print the size of the vector System.out.println("Size of the vector: "+vec.capacity()); // trim the size of the vector System.out.println("Trimming the vector"); vec.trimToSize(); System.out.println("Size of the vector: "+vec.capacity()); } }
输出
让我们编译并运行上面的程序,这将产生以下结果。
Size of the vector: 10 Trimming the vector Size of the vector: 4
将字符串向量调整为指定大小的示例
以下示例演示了 Java Vector trimToSize() 方法的用法。我们使用 add() 方法为 Vector 对象添加几个字符串,并打印向量的容量。然后,我们使用 trimToSize() 调整向量的大小,并再次打印其容量以反映更改。
package com.tutorialspoint; import java.util.Vector; public class VectorDemo { public static void main(String[] args) { // create an empty Vector vec with an initial capacity of 4 Vector<String> vec = new Vector<>(10); // use add() method to add elements in the vector vec.add("Welcome"); vec.add("To"); vec.add("Tutorialspoint"); // let us print the size of the vector System.out.println("Size of the vector: "+vec.capacity()); // trim the size of the vector System.out.println("Trimming the vector"); vec.trimToSize(); System.out.println("Size of the vector: "+vec.capacity()); } }
输出
让我们编译并运行上面的程序,这将产生以下结果。
Size of the vector: 10 Trimming the vector Size of the vector: 3
将对象向量调整为指定大小的示例
以下示例演示了 Java Vector trimToSize() 方法的用法。我们使用 add() 方法为 Vector 对象添加几个 Student 对象,并打印向量的容量。然后,我们使用 trimToSize() 调整向量的大小,并再次打印其容量以反映更改。
package com.tutorialspoint; import java.util.Vector; public class VectorDemo { public static void main(String[] args) { // create an empty Vector vec with an initial capacity of 4 Vector<Student> vec = new Vector<>(10); // use add() method to add elements in the vector vec.add(new Student(1, "Julie")); vec.add(new Student(2, "Robert")); vec.add(new Student(3, "Adam")); // let us print the size of the vector System.out.println("Size of the vector: "+vec.capacity()); // trim the size of the vector System.out.println("Trimming the vector"); vec.trimToSize(); System.out.println("Size of the vector: "+vec.capacity()); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
输出
让我们编译并运行上面的程序,这将产生以下结果。
Size of the vector: 10 Trimming the vector Size of the vector: 3
java_util_vector.htm
广告