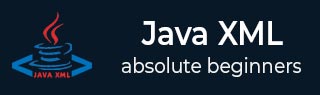
- Java XML 教程
- Java XML 首页
- Java XML 概述
- Java XML 解析器
- Java DOM 解析器
- Java DOM 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java SAX 解析器
- Java SAX 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- JDOM XML 解析器
- JDOM XML 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java StAX 解析器
- Java StAX 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java XPath 解析器
- Java XPath 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java DOM4J 解析器
- Java DOM4J 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java XML 有用资源
- Java XML - 问题和解答
- Java XML - 快速指南
- Java XML - 有用资源
- Java XML - 讨论
Java JDOM Document addContent() 方法
Java JDOM 的 addContent() 方法是 Document 类的一个方法,用于向 XML 文档添加内容。使用此方法,我们可以向文档添加根元素、注释和处理指令。
如果未提供索引值,此方法会将内容添加到文档的末尾。如果使用了索引值,则会在指定位置追加内容对象或内容列表。如果传递的索引为负数或大于文档现有子元素的数量,则会抛出 IndexOutOfBoundsException 异常。如果我们尝试添加的内容对象已存在父元素,则会抛出 IllegalAddException 异常。
语法
以下是 Java JDOM Document addContent() 方法的语法:
Document.addContent(child) Document.addContent(index, child) Document.addContent(list) Document.addContent(index,list)
参数
Java addContent() 方法是一个多态方法,每个方法都有不同的参数。
child - 表示一个 Content 对象。这可以是 Element、Comment、DocType、EntityRef、ProcessingInstruction 和 Text。
index - 表示我们需要插入 Content 对象或 Content 对象列表的索引。
list - 表示 Content 对象的列表。
返回值
此方法返回添加内容的 Document。
示例 1
这是一个基本示例,它使用 Java JDOM Document addContent() 方法向 XML 文档添加根元素。
import org.jdom2.Document; import org.jdom2.Element; import org.jdom2.output.XMLOutputter; public class AddRootElement { public static void main(String args[]) { try { //Create a new Document Document doc = new Document(); //Create root Element Element root = new Element("company").setText("xyz"); //Add root Element doc.addContent(root); //Print document XMLOutputter xmlOutput = new XMLOutputter(); xmlOutput.output(doc, System.out); } catch (Exception e) { e.printStackTrace(); } } }
输出窗口显示包含具有文本内容的根元素的 XML 文档。
<?xml version="1.0" encoding="UTF-8"?> <company>xyz</company>
示例 2
现在,让我们尝试在与根元素相同的级别添加另一个元素,这将抛出一个名为 IllegalAddException 的异常。以下示例说明了这种情况。
import org.jdom2.Document; import org.jdom2.Element; import org.jdom2.output.XMLOutputter; public class AddRootElement { public static void main(String args[]) { try { //Adding root to new Document Document doc = new Document(); Element root = new Element("company").setText("xyz"); //Trying to add one more root doc.addContent(0,root); Element root2 = new Element("footer"); doc.addContent(1,root2); //Print document XMLOutputter xmlOutput = new XMLOutputter(); xmlOutput.output(doc, System.out); } catch (Exception e) { e.printStackTrace(); } } }
org.jdom2.IllegalAddException: Cannot add a second root element, only one is allowed
示例 3
我们需要在下面的 EmpData.xml 文件末尾添加注释:
<Company> <Employee>John</Employee> <Employee>Daniel</Employee> </Company>
为了在文档末尾添加注释,在以下示例中,我们创建了一个新的 Comment 对象,并使用了 addContent(Comment) 方法将其添加到 XML 文档中。
import java.io.File; import org.jdom2.Comment; import org.jdom2.Document; import org.jdom2.input.SAXBuilder; import org.jdom2.output.Format; import org.jdom2.output.XMLOutputter; public class AddComment { public static void main(String args[]) { try { //Reading the XML file SAXBuilder saxBuilder = new SAXBuilder(); File inputFile = new File("EmpData.xml"); Document doc = saxBuilder.build(inputFile); //Adding comment at the end Comment comment = new Comment("You have reached the end of the document!"); doc.addContent(comment); //Printing the document XMLOutputter xmlOutput = new XMLOutputter(); xmlOutput.setFormat(Format.getPrettyFormat()); xmlOutput.output(doc, System.out); } catch (Exception e) { e.printStackTrace(); } } }
输出窗口显示文档的内容以及末尾的注释。
<?xml version="1.0" encoding="UTF-8"?> <Company> <Employee>John</Employee> <Employee>Daniel</Employee> </Company> <!--You have reached the end of the document!-->
示例 4
addContent(List<Content>) 方法将内容列表添加到文档的末尾。以下程序创建一个新文档并添加包含注释和根元素的内容列表。
import java.util.ArrayList; import java.util.List; import org.jdom2.Comment; import org.jdom2.Content; import org.jdom2.Document; import org.jdom2.Element; import org.jdom2.output.Format; import org.jdom2.output.XMLOutputter; public class AddContent { public static void main(String args[]) { try { Document doc = new Document(); //Preparing the content list Comment comment = new Comment("This is the start of the document!"); Element root = new Element("company").setText("xyz"); List<Content> list = new ArrayList<>(); list.add(comment); list.add(root); //Adding the list doc.addContent(list); //Print document XMLOutputter xmlOutput = new XMLOutputter(); xmlOutput.setFormat(Format.getPrettyFormat()); xmlOutput.output(doc, System.out); } catch (Exception e) { e.printStackTrace(); } } }
输出窗口显示 XML 文档的内容。
<?xml version="1.0" encoding="UTF-8"?> <!--This is the start of the document!--> <company>xyz</company>