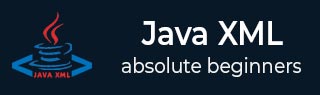
- Java XML 教程
- Java XML 首页
- Java XML 概述
- Java XML 解析器
- Java DOM 解析器
- Java DOM 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java SAX 解析器
- Java SAX 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- JDOM XML 解析器
- JDOM XML 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java StAX 解析器
- Java StAX 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java XPath 解析器
- Java XPath 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java DOM4J 解析器
- Java DOM4J 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java XML 有用资源
- Java XML - 问答
- Java XML - 快速指南
- Java XML - 有用资源
- Java XML - 讨论
Java JDOM Document clone() 方法
Java JDOM 的clone()方法用于获取文档的深拷贝。它创建当前文档的精确副本。
语法
以下是 Java JDOM Document clone() 方法的语法:
Document.clone();
参数
Java clone() 方法不接受任何参数。
返回值
Java clone() 方法返回当前 Document 对象的副本。
示例 1
在这个基本示例中,我们创建了一个 XML 文档,并使用 Java JDOM Document clone() 方法获取其精确副本。
import org.jdom2.Document; import org.jdom2.Element; import org.jdom2.output.XMLOutputter; public class CloneDocument { public static void main(String args[]) { try { //Create a new Document Document doc = new Document(); //Create root Element Element root = new Element("bookstore").setText("Coffee with Pages"); //Add root Element doc.addContent(root); //Cloning the document Document cloneDoc = doc.clone(); //Printing document XMLOutputter xmlOutput = new XMLOutputter(); System.out.println("-----------Original Document-----------\n"); xmlOutput.output(doc, System.out); System.out.println("\n-----------Cloned Document-----------\n"); xmlOutput.output(cloneDoc, System.out); } catch (Exception e) { e.printStackTrace(); } } }
输出窗口显示原始文档和克隆文档。
-----------Original Document----------- <?xml version="1.0" encoding="UTF-8"?> <bookstore>Coffee with Pages</bookstore> -----------Cloned Document----------- <?xml version="1.0" encoding="UTF-8"?> <bookstore>Coffee with Pages</bookstore>
示例 2
我们需要克隆以下bookstore.xml文件:
<?xml version="1.0" encoding="UTF-8"?> <!-- Information of a Bookstore (last updated o5-08-2024) --> <bookstore> <!-- All the books in the store are listed --> <book> <name>Treasure Island</name> <author>Robert Louis</author> <price>1400</price> </book> <book> <name>Oliver Twist</name> <author>Charles Dickens</author> <price>2000</price> </book> <book> <name>War and Peace</name> <author>Leo Tolstoy</author> <price>1500</price> </book> </bookstore>
让我们读取现有的 bookstore.xml 文件并获取此文档的克隆。为此,我们首先读取 bookstore.xml 文件,并使用 SAXBuilder 构建文档。然后,我们使用 clone() 方法获取此文档的深拷贝。
import java.io.File; import org.jdom2.Document; import org.jdom2.input.SAXBuilder; import org.jdom2.output.Format; import org.jdom2.output.XMLOutputter; public class CloneDocument { public static void main(String args[]) { try { //Reading the document SAXBuilder saxBuilder = new SAXBuilder(); File inputFile = new File("bookstore.xml"); Document doc = saxBuilder.build(inputFile); //Cloning the document Document cloneDoc = doc.clone(); //Printing document XMLOutputter xmlOutput = new XMLOutputter(); xmlOutput.setFormat(Format.getPrettyFormat()); System.out.println("-----------Original Document-----------\n"); xmlOutput.output(doc, System.out); System.out.println("\n-----------Cloned Document-----------\n"); xmlOutput.output(cloneDoc, System.out); } catch (Exception e) { e.printStackTrace(); } } }
输出窗口显示原始 bookstore.xml 文件及其克隆文档。
-----------Original Document----------- <?xml version="1.0" encoding="UTF-8"?> <!-- Information of a Bookstore (last updated o5-08-2024) --> <bookstore> <!-- All the books in the store are listed --> <book> <name>Treasure Island</name> <author>Robert Louis</author> <price>1400</price> </book> <book> <name>Oliver Twist</name> <author>Charles Dickens</author> <price>2000</price> </book> <book> <name>War and Peace</name> <author>Leo Tolstoy</author> <price>1500</price> </book> </bookstore> -----------Cloned Document----------- <?xml version="1.0" encoding="UTF-8"?> <!-- Information of a Bookstore (last updated o5-08-2024) --> <bookstore> <!-- All the books in the store are listed --> <book> <name>Treasure Island</name> <author>Robert Louis</author> <price>1400</price> </book> <book> <name>Oliver Twist</name> <author>Charles Dickens</author> <price>2000</price> </book> <book> <name>War and Peace</name> <author>Leo Tolstoy</author> <price>1500</price> </book> </bookstore>
广告