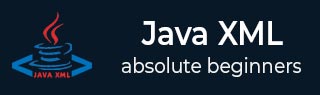
- Java XML 教程
- Java XML 主页
- Java XML 概述
- Java XML 解析器
- Java DOM 解析器
- Java DOM 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java SAX 解析器
- Java SAX 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- JDOM XML 解析器
- JDOM XML 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java StAX 解析器
- Java StAX 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java XPath 解析器
- Java XPath 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java DOM4J 解析器
- Java DOM4J 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java XML 有用资源
- Java XML - 问题和解答
- Java XML - 快速指南
- Java XML - 有用资源
- Java XML - 讨论
Java JDOM Document cloneContent() 方法
Document 类的 Java JDOM cloneContent() 方法用于以列表形式获取 XML 文档的内容。该列表包含所有项目,形式为 Content 对象。这些 Content 对象包括注释、根元素和 XML DTD。
语法
以下是 Java JDOM Document cloneContent() 方法的语法 -
Document.cloneContent();
参数
Java cloneContent() 方法不接受任何参数。
返回值
Java cloneContent() 方法返回 Content 对象列表。
示例 1
以下是使用 Java JDOM Document cloneContent() 方法的基本示例 -
import java.util.List; import org.jdom2.Content; import org.jdom2.Document; import org.jdom2.Element; public class CloneContent { public static void main(String args[]) { try { //Create a new Document Document doc = new Document(); //Create root Element Element root = new Element("bookstore").setText("Coffee with Pages"); //Add root Element doc.addContent(root); //Cloning the document content List<Content> clonedList = doc.cloneContent(); //Printing the list items for(Content c : clonedList) { System.out.println(c); } } catch (Exception e) { e.printStackTrace(); } } }
输出窗口显示根元素的名称。
[Element: <bookstore/>]
示例 2
我们需要解析以下 bookstore.xml 文件 -
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE bookstore[ <!ELEMENT bookstore (book+)> <!ELEMENT book (name,author,price)> <!ELEMENT name (#PCDATA)> <!ELEMENT author (#PCDATA)> <!ELEMENT price (#PCDATA)> ]> <!-- Information of a Bookstore (last updated 05-08-2024) --> <!-- All the books in the store are listed --> <bookstore> <book> <name>Treasure Island</name> <author>Robert Louis</author> <price>1400</price> </book> <book> <name>Oliver Twist</name> <author>Charles Dickens</author> <price>2000</price> </book> <book> <name>War and Peace</name> <author>Leo Tolstoy</author> <price>1500</price> </book> </bookstore>
现在,让我们读取上述 bookstore.xml 文件并使用 cloneContent() 方法获取 Content 对象列表。
import java.io.File; import java.util.List; import org.jdom2.Content; import org.jdom2.Document; import org.jdom2.input.SAXBuilder; public class CloneContent { public static void main(String args[]) { try { //Reading the document SAXBuilder saxBuilder = new SAXBuilder(); File inputFile = new File("bookstore.xml"); Document doc = saxBuilder.build(inputFile); //Cloning the document content List<Content> clonedList = doc.cloneContent(); //Printing the list items for(Content c : clonedList) { System.out.println(c); } } catch (Exception e) { e.printStackTrace(); } } }
XML 文档的所有四个 Content 对象都已打印出来。
[DocType: <!DOCTYPE bookstore>] [Comment: <!-- Information of a Bookstore (last updated 05-08-2024) -->] [Comment: <!-- All the books in the store are listed -->] [Element: <bookstore/>]
广告