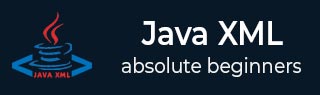
- Java XML 教程
- Java XML 首页
- Java XML 概述
- Java XML 解析器
- Java DOM 解析器
- Java DOM 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java SAX 解析器
- Java SAX 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- JDOM XML 解析器
- JDOM XML 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java StAX 解析器
- Java StAX 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java XPath 解析器
- Java XPath 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java DOM4J 解析器
- Java DOM4J 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java XML 有用资源
- Java XML - 问题与解答
- Java XML - 快速指南
- Java XML - 有用资源
- Java XML - 讨论
Java JDOM 文档 detachRootElement() 方法
Java JDOM 的 detachRootElement() 方法是 Document 类的方法,用于分离并返回 XML 文档中的根元素。使用此方法,我们只能获取根元素的名称。它不会返回文本内容或与子元素相关的任何信息。
此方法会移除根元素及其包含的所有内容。要获取根元素而不将其从文档中删除,可以使用 Document 类的 getRootElement() 方法。
语法
以下是 Java JDOM Document detachRootElement() 方法的语法:
Document.detachRootElement();
参数
Java detachRootElement() 方法不接受任何参数。
返回值
Java detachRootElement() 方法以 Element 对象的形式返回根元素。
示例 1
以下是使用 Java JDOM Document detachRootElement() 方法的基本示例:
import org.jdom2.Document; import org.jdom2.Element; public class DetachRootElement { public static void main(String args[]) { try { //Creating a new Document and adding the root Document doc = new Document(); Element element = new Element("root"); doc.addContent(element); //Detaching the root Element root = doc.detachRootElement(); System.out.println(root); } catch (Exception e) { e.printStackTrace(); } } }
根元素名称“root”将显示在输出屏幕上。
[Element: <root/>]
示例 2
如果 XML 文档中没有可用的根元素,则 detachRootElement() 方法将返回 null。以下程序尝试从空文档中分离根元素,并返回 null。
import org.jdom2.Document; import org.jdom2.Element; public class DetachRootElement { public static void main(String args[]) { try { //Creating a new Document Document doc = new Document(); //Detaching the root Element root = doc.detachRootElement(); System.out.println(root); } catch (Exception e) { e.printStackTrace(); } } }
输出窗口显示 null。
null
示例 3
以下是 bookstore.xml 文件,我们需要从中分离根元素:
<?xml version="1.0" encoding="UTF-8"?> <!-- Information of a Bookstore (last updated 05-08-2024) --> <bookstore> <book>Treasure Island</book> <book>War and Peace</book>> </bookstore>
以下 Java 程序读取上述 bookstore.xml 文件,使用 SAXBuilder 构建文档,并使用 detachRootElement() 方法分离根元素。
import java.io.File; import org.jdom2.Document; import org.jdom2.Element; import org.jdom2.input.SAXBuilder; import org.jdom2.output.XMLOutputter; public class DetachRootElement { public static void main(String args[]) { try { //Reading the document SAXBuilder saxBuilder = new SAXBuilder(); File inputFile = new File("bookstore.xml"); Document doc = saxBuilder.build(inputFile); //Detaching the root Element root = doc.detachRootElement(); System.out.println(root); //Printing the document XMLOutputter xmlOutput = new XMLOutputter(); System.out.println("\n--------XML document after detaching the root--------\n"); xmlOutput.output(doc, System.out); } catch (Exception e) { e.printStackTrace(); } } }
输出窗口显示分离的根元素和分离根元素后的文档。
[Element: <bookstore/>] --------XML document after detaching the root-------- <?xml version="1.0" encoding="UTF-8"?> <!-- Information of a Bookstore (last updated 05-08-2024) -->
广告