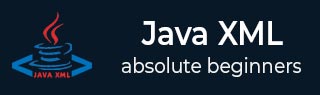
- Java XML 教程
- Java XML 首页
- Java XML 概述
- Java XML 解析器
- Java DOM 解析器
- Java DOM 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java SAX 解析器
- Java SAX 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- JDOM XML 解析器
- JDOM XML 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java StAX 解析器
- Java StAX 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java XPath 解析器
- Java XPath 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java DOM4J 解析器
- Java DOM4J 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java XML 有用资源
- Java XML - 问题与解答
- Java XML - 快速指南
- Java XML - 有用资源
- Java XML - 讨论
Java JDOM Document 的 getContentSize() 方法
Java JDOM 的getContentSize()方法是Document类的一个方法,用于检索XML文档中内容对象的个数。此方法统计DTD声明、处理指令、注释和根元素。它不统计根元素内部的子元素和注释;而是将根元素的整个内容作为一个单一元素进行计数。
语法
以下是Java JDOM Document getContentSize()方法的语法:
Document.getContentSize();
参数
Java getContentSize()方法不接受任何参数。
返回值
Java getContentSize()方法返回内容对象的个数,以整数形式表示。
示例1
以下基本示例创建一个文档并添加一个根元素。使用Java JDOM Document getContentSize()方法,我们将检索内容对象的个数。
import org.jdom2.Document; import org.jdom2.Element; public class GetContentSize { public static void main(String args[]) { try { //Creating a new Document and adding the root Document doc = new Document(); Element root = new Element("book"); doc.addContent(root); //Get the content size int content_objs = doc.getContentSize(); System.out.println("No.of content objects : "+content_objs); } catch (Exception e) { e.printStackTrace(); } } }
输出窗口显示内容对象的个数。
No.of content objects : 1
示例2
如果文档中没有内容,getContentSize()方法将返回'0'。以下示例说明了这种情况:
import org.jdom2.Document; public class GetContentSize { public static void main(String args[]) { try { //Creating a new document Document doc = new Document(); //Get the content size int content_objs = doc.getContentSize(); System.out.println("No.of content objects : "+content_objs); } catch (Exception e) { e.printStackTrace(); } } }
输出窗口显示内容对象的个数为'0'。
No.of content objects : 0
示例3
让我们计算以下bookstore.xml文件中内容对象的个数。
<?xml version="1.0" encoding="UTF-8"?> <?xml-stylesheet type="text/xsl" href="style.xsl"?> <!DOCTYPE bookstore[ <!ELEMENT bookstore (book+)> <!ELEMENT book (name,author,price)> <!ELEMENT name (#PCDATA)> <!ELEMENT author (#PCDATA)> <!ELEMENT price (#PCDATA)> ]> <!-- Information of a Bookstore (last updated 05-08-2024) --> <!-- All the books in the store are listed --> <bookstore> <book> <name>Treasure Island</name> <author>Robert Louis</author> <price>1400</price> </book> <book> <name>Oliver Twist</name> <author>Charles Dickens</author> <price>2000</price> </book> <book> <name>War and Peace</name> <author>Leo Tolstoy</author> <price>1500</price> </book> </bookstore>
以下程序读取上述XML文件,并使用getContentSize()方法计算内容对象的个数。
import java.io.File; import org.jdom2.Document; import org.jdom2.input.SAXBuilder; public class GetContentSize { public static void main(String args[]) { try { //Reading the document SAXBuilder saxBuilder = new SAXBuilder(); File inputFile = new File("bookstore.xml"); Document doc = saxBuilder.build(inputFile); //Get the content size int content_objs = doc.getContentSize(); System.out.println("No.of content objects : "+content_objs); } catch (Exception e) { e.printStackTrace(); } } }
程序计算出1个处理指令、1个DTD声明、2个注释和1个根元素,并将计数显示为5。
No.of content objects : 5
广告