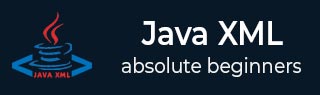
- Java XML 教程
- Java XML 首页
- Java XML 概述
- Java XML 解析器
- Java DOM 解析器
- Java DOM 解析器
- 解析XML文档
- 查询XML文档
- 创建XML文档
- 修改XML文档
- Java SAX 解析器
- Java SAX 解析器
- 解析XML文档
- 查询XML文档
- 创建XML文档
- 修改XML文档
- JDOM XML 解析器
- JDOM XML 解析器
- 解析XML文档
- 查询XML文档
- 创建XML文档
- 修改XML文档
- Java StAX 解析器
- Java StAX 解析器
- 解析XML文档
- 查询XML文档
- 创建XML文档
- 修改XML文档
- Java XPath 解析器
- Java XPath 解析器
- 解析XML文档
- 查询XML文档
- 创建XML文档
- 修改XML文档
- Java DOM4J 解析器
- Java DOM4J 解析器
- 解析XML文档
- 查询XML文档
- 创建XML文档
- 修改XML文档
- Java XML有用资源
- Java XML - 问答
- Java XML - 快速指南
- Java XML - 有用资源
- Java XML - 讨论
Java JDOM文档的getRootElement()方法
Java JDOM Document 的getRootElement()方法用于从Document类中检索XML文档的根元素。
语法
以下是Java JDOM Document getRootElement()方法的语法:
Document.getRootElement();
参数
Java getRootElement()方法不接受任何参数。
返回值
Java getRootElement()方法返回一个Element对象,表示根元素。
示例1
这是一个基本的示例,解释了Java JDOM Document getRootElement()方法的使用:
import org.jdom2.Document; import org.jdom2.Element; public class GetRootElement { public static void main(String args[]) { try { //Creating a new Document and adding the root Document doc = new Document(); Element element = new Element("company"); doc.addContent(element); //Getting the root Element root = doc.getRootElement(); System.out.println(root); } catch (Exception e) { e.printStackTrace(); } } }
输出窗口显示根元素的名称。
[Element: <company/>]
示例2
当尝试获取没有根元素的文档的根元素时,getRootElement()方法会抛出IllegalStateException异常。
以下示例说明了这种情况:
import org.jdom2.Document; import org.jdom2.Element; public class GetRootElement { public static void main(String args[]) { try { //Creating a new Document Document doc = new Document(); //Getting the root Element root = doc.getRootElement(); System.out.println(root); } catch (Exception e) { e.printStackTrace(); } } }
以上程序抛出以下异常:
java.lang.IllegalStateException: Root element not set at org.jdom2.Document.getRootElement(Document.java:220) at getRootElement.Example2.main(Example2.java:12)
示例3
getRootElement()方法只从文档中检索根元素。它不会像detachRootElement()方法那样删除根元素。
我们要从中获取根元素的bookstore.xml文件:
<?xml version="1.0" encoding="UTF-8"?> <!-- Information of a Bookstore (last updated 05-08-2024) --> <bookstore> <book>Treasure Island</book> <book>War and Peace</book> </bookstore>
下面的程序使用getRootElement()方法检索根元素,并打印文档以查看根元素是否保留在文档中。
import java.io.File; import org.jdom2.Document; import org.jdom2.Element; import org.jdom2.input.SAXBuilder; import org.jdom2.output.Format; import org.jdom2.output.XMLOutputter; public class GetRootElement { public static void main(String args[]) { try { //Reading the document SAXBuilder saxBuilder = new SAXBuilder(); File inputFile = new File("bookstore.xml"); Document doc = saxBuilder.build(inputFile); //Getting the root Element root = doc.getRootElement(); System.out.println(root); //Printing the document XMLOutputter xmlOutput = new XMLOutputter(); xmlOutput.setFormat(Format.getPrettyFormat()); System.out.println("\n--------XML document after getting the root--------\n"); xmlOutput.output(doc, System.out); } catch (Exception e) { e.printStackTrace(); } } }
输出窗口显示根元素以及使用该方法后的整个文档。
[Element: <bookstore/>] --------XML document after getting the root-------- <?xml version="1.0" encoding="UTF-8"?> <!-- Information of a Bookstore (last updated 05-08-2024) --> <bookstore> <book>Treasure Island</book> <book>War and Peace</book> </bookstore>
广告