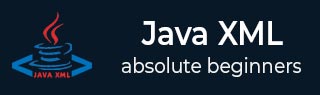
- Java XML 教程
- Java XML 首页
- Java XML 概述
- Java XML 解析器
- Java DOM 解析器
- Java DOM 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java SAX 解析器
- Java SAX 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- JDOM XML 解析器
- JDOM XML 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java StAX 解析器
- Java StAX 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java XPath 解析器
- Java XPath 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java DOM4J 解析器
- Java DOM4J 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java XML 有用资源
- Java XML - 问答
- Java XML - 快速指南
- Java XML - 有用资源
- Java XML - 讨论
Java JDOM Document removeContent() 方法
Java JDOM 的removeContent()方法(Document 类的方法)用于从 XML 文档中移除文档级别的内容对象。使用此方法,可以从 XML 文档中移除注释、DocType声明和根元素。如果尝试移除索引超出文档内容对象数量范围的内容对象,此方法将抛出IndexOutOfBoundsException异常。
removeContent()方法可用于移除特定类型的内容对象,例如,移除文档中的所有注释。此方法不用于移除根元素内包含的子级元素。它会移除整个根元素及其内部的内容(子元素、注释)。
要移除根元素内的子元素,可以使用 JDOM 的 Element 类的removeChild()、removeChildren() 和 removeContent() 方法。
语法
以下是 Java JDOM Document removeContent() 方法的语法:
Document.removeContent() Document.removeContent(index) Document.removeContent(child) Document.removeContent(filter)
参数
Java removeContent() 方法是一个多态方法,每个方法都有不同的参数。
child - 表示要移除的内容对象。可以是 Element、Comment、DocType、EntityRef、ProcessingInstruction 和 Text。
index - 表示需要移除的内容对象的索引。
filter - 表示特定类型内容的过滤器对象(Element 过滤器、注释过滤器等)。
返回值
此方法返回已移除的内容对象的列表。
示例 1
以下bookstore.xml文件用于所有示例中,以了解 Java JDOM removeContent() 方法的功能。
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE bookstore[ <!ELEMENT bookstore (book+)> <!ELEMENT book (#PCDATA)> ]> <!-- Information of a Bookstore (last updated 05-08-2024) --> <!-- All the books in the store are listed --> <bookstore> <book>Treasure Island</book> <book>Oliver Twist</book> </bookstore>
当没有传递参数时,removeContent() 方法会移除 XML 文档中的所有内容,只留下处理指令。
import java.io.File; import org.jdom2.Document; import org.jdom2.input.SAXBuilder; import org.jdom2.output.Format; import org.jdom2.output.XMLOutputter; public class RemoveContent { public static void main(String args[]) { try { //Reading the document SAXBuilder saxBuilder = new SAXBuilder(); File inputFile = new File("bookstore.xml"); Document doc = saxBuilder.build(inputFile); //Printing the document XMLOutputter xmlOutput = new XMLOutputter(); xmlOutput.setFormat(Format.getPrettyFormat()); System.out.println("-------XML Document BEFORE removing the content-------\n"); xmlOutput.output(doc, System.out); //Removing content doc.removeContent(); System.out.println("\n-------XML Document AFTER removing the content-------\n"); xmlOutput.output(doc, System.out); } catch (Exception e) { e.printStackTrace(); } } }
显示移除内容前后的 XML 文档内容。
-------XML Document BEFORE removing the content------- <?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE bookstore> <!-- Information of a Bookstore (last updated 05-08-2024) --> <!-- All the books in the store are listed --> <bookstore> <book>Treasure Island</book> <book>Oliver Twist</book> </bookstore> -------XML Document AFTER removing the content------- <?xml version="1.0" encoding="UTF-8"?>
示例 2
要移除指定索引处的 Content 对象,可以将索引作为参数传递给 removeContent() 方法,如下所示:
import java.io.File; import org.jdom2.Document; import org.jdom2.input.SAXBuilder; public class RemoveContent { public static void main(String args[]) { try { //Reading the document SAXBuilder saxBuilder = new SAXBuilder(); File inputFile = new File("bookstore.xml"); Document doc = saxBuilder.build(inputFile); //Removing Content object doc.removeContent(2); System.out.println("Content object at index 2 is removed."); } catch (Exception e) { e.printStackTrace(); } } }
输出窗口显示一个简单的打印语句,表明内容已移除。
Content object at index 2 is removed.
示例 3
可以通过将内容对象作为参数传递给 removeContent() 方法,从 XML 文档中移除特定内容对象。
import org.jdom2.Comment; import org.jdom2.Document; import org.jdom2.Element; import org.jdom2.output.Format; import org.jdom2.output.XMLOutputter; public class RemoveContent { public static void main(String args[]) { try { //Create a new Document and add root Document doc = new Document(); Element root = new Element("company").setText("xyz"); Comment comment = new Comment("This is the company data."); doc.addContent(comment); doc.addContent(root); //Print document XMLOutputter xmlOutput = new XMLOutputter(); xmlOutput.setFormat(Format.getPrettyFormat()); System.out.println("-------XML Document after adding content-------\n"); xmlOutput.output(doc, System.out); //remove comment doc.removeContent(comment); System.out.println("\n-------XML Document after removing the comment-------\n"); xmlOutput.output(doc, System.out); } catch (Exception e) { e.printStackTrace(); } } }
输出窗口显示移除 Comment 对象前后的 XML 文档。
-------XML Document after adding content------- <?xml version="1.0" encoding="UTF-8"?> <!--This is the company data.--> <company>xyz</company> -------XML Document after removing the comment------- <?xml version="1.0" encoding="UTF-8"?> <company>xyz</company>
示例 4
Filters 类的 comment() 方法返回 Comment 对象的过滤器,可以将其作为参数传递给 removeContent() 方法,以仅移除文档中的注释。类似地,可以使用 Element 过滤器来移除根元素。
import org.jdom2.Comment; import org.jdom2.filter.Filter; import org.jdom2.filter.Filters; import java.io.File; import java.util.List; import org.jdom2.Document; import org.jdom2.input.SAXBuilder; import org.jdom2.output.XMLOutputter; public class RemoveComments { public static void main(String args[]) { try { //Reading the document SAXBuilder saxBuilder = new SAXBuilder(); File inputFile = new File("bookstore.xml"); Document doc = saxBuilder.build(inputFile); //Removing comments Filter<Comment> comment_filter = Filters.comment(); List<Comment> commentList = doc.removeContent(comment_filter); //Printing the removed comments System.out.println("Removed Comments: \n"); for(Comment c : commentList) { System.out.println(c); } XMLOutputter xmlOutput = new XMLOutputter(); System.out.println("\n-------XML Document after removing comments-------\n"); xmlOutput.output(doc, System.out); } catch (Exception e) { e.printStackTrace(); } } }
输出窗口显示移除注释前后的 XML 文档。
Removed Comments: [Comment: <!-- Information of a Bookstore (last updated 05-08-2024) -->] [Comment: <!-- All the books in the store are listed -->] -------XML Document after removing comments------- <?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE bookstore><bookstore> <book>Treasure Island</book> <book>Oliver Twist</book> </bookstore>