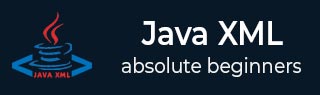
- Java XML 教程
- Java XML 首页
- Java XML 概述
- Java XML 解析器
- Java DOM 解析器
- Java DOM 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java SAX 解析器
- Java SAX 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- JDOM XML 解析器
- JDOM XML 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java StAX 解析器
- Java StAX 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java XPath 解析器
- Java XPath 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java DOM4J 解析器
- Java DOM4J 解析器
- 解析 XML 文档
- 查询 XML 文档
- 创建 XML 文档
- 修改 XML 文档
- Java XML 有用资源
- Java XML - 问题与解答
- Java XML - 快速指南
- Java XML - 有用资源
- Java XML - 讨论
Java JDOM Document setContent() 方法
Java JDOM 的 setContent() 方法是 Document 类的方法,用于替换 XML 文档中的文档级内容对象。内容对象包括 DocType 定义、注释、处理指令和根元素。
当我们尝试在文档中错误的索引处替换内容对象时,setContent() 方法会抛出 indexOutOfBoundsException 异常。
语法
以下是 Java JDOM Document setContent() 方法的语法:
Document.setContent(child) Document.setContent(index, child) Document.setContent(list) Document.setContent(index, list)
参数
Java setContent() 方法是一个多态方法,每个方法都有不同的参数。
child - 表示一个 Content 对象。可以是 Element、Comment、DocType、EntityRef、ProcessingInstruction 和 Text。
index - 表示我们需要插入 Content 或 Content 对象列表的索引。
list - 表示 Content 对象的列表。
返回值
此方法返回替换内容的 Document 对象。
示例 1
Java JDOM Document setContent() 方法用作为参数传递的新根元素替换现有的根元素。
import org.jdom2.Document; import org.jdom2.Element; public class setNewRoot { public static void main(String args[]) { try { //Creating new document and adding root Document doc = new Document(); Element root = new Element("root"); doc.addContent(root); //Replacing the root Element new_root = new Element("new_root"); doc.setContent(new_root); System.out.println(doc); } catch(Exception e) { e.printStackTrace(); } } }
显示设置新根元素后的更新文档。
[Document: No DOCTYPE declaration, Root is [Element: <new_root/>]]
示例 2
我们需要替换以下 collegeData.xml 文件的注释:
<!-- This is college data.--> <college> <department>Computer Science</department> <department>Electrical and Electronics</department> <department>Mechanical</department> </college>
setContent(index,comment) 方法用作为第二个参数传递的注释替换指定索引处的 content 对象。
import java.io.File; import org.jdom2.Comment; import org.jdom2.Document; import org.jdom2.input.SAXBuilder; import org.jdom2.output.Format; import org.jdom2.output.XMLOutputter; public class ReplaceComment { public static void main(String args[]) { try { //Reading the document SAXBuilder saxBuilder = new SAXBuilder(); File inputFile = new File("collegeData.xml"); Document doc = saxBuilder.build(inputFile); //Replacing comment Comment comment = new Comment("Start of the document"); doc.setContent(0,comment); //Printing the document XMLOutputter xmlOutput = new XMLOutputter(); xmlOutput.setFormat(Format.getPrettyFormat()); xmlOutput.output(doc, System.out); } catch(Exception e) { e.printStackTrace(); } } }
显示替换注释后的更新文档。
<?xml version="1.0" encoding="UTF-8"?> <!--Start of the document--> <college> <department>Computer Science</department> <department>Electrical and Electronics</department> <department>Mechanical</department> </college>
示例 3
setContent(index,list) 方法用作为第二个参数提供的 content 列表替换指定索引处的 content 对象。
import java.io.File; import java.util.ArrayList; import java.util.List; import org.jdom2.Comment; import org.jdom2.Content; import org.jdom2.DocType; import org.jdom2.Document; import org.jdom2.input.SAXBuilder; import org.jdom2.output.Format; import org.jdom2.output.XMLOutputter; public class SetContentList { public static void main(String args[]) { try { //Reading the document SAXBuilder saxBuilder = new SAXBuilder(); File inputFile = new File("collegeData.xml"); Document doc = saxBuilder.build(inputFile); //Adding content list DocType docType = new DocType("college"); Comment comment = new Comment("Last updated date : 09-08-2024"); List<Content> list = new ArrayList<Content>(); list.add(docType); list.add(comment); doc.setContent(0,list); //Printing the document XMLOutputter xmlOutput = new XMLOutputter(); xmlOutput.setFormat(Format.getPrettyFormat()); xmlOutput.output(doc, System.out); } catch(Exception e) { e.printStackTrace(); } } }
显示在索引 0 处添加 content 列表后的更新文档。
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE college> <!--Last updated date : 09-08-2024--> <college> <department>Computer Science</department> <department>Electrical and Electronics</department> <department>Mechanical</department> </college>
广告