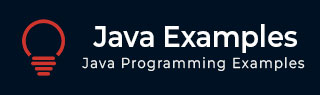
- Java 编程示例
- 示例 - 首页
- 示例 - 环境
- 示例 - 字符串
- 示例 - 数组
- 示例 - 日期和时间
- 示例 - 方法
- 示例 - 文件
- 示例 - 目录
- 示例 - 异常
- 示例 - 数据结构
- 示例 - 集合
- 示例 - 网络
- 示例 - 多线程
- 示例 - 小应用程序
- 示例 - 简单 GUI
- 示例 - JDBC
- 示例 - 常规表达式
- 示例 - Apache PDF Box
- 示例 - Apache POI PPT
- 示例 - Apache POI Excel
- 示例 - Apache POI Word
- 示例 - OpenCV
- 示例 - Apache Tika
- 示例 - iText
- Java 教程
- Java - 教程
- 有用的 Java 资源
- Java - 快速指南
- Java - 有用资源
如何在 Java 中打印数字总和
问题描述
如何打印数字总和?
解决方案
以下示例演示了如何使用堆栈概念来相加前 n 个自然数。
import java.io.IOException; public class AdditionStack { static int num; static int ans; static Stack theStack; public static void main(String[] args) throws IOException { num = 50; stackAddition(); System.out.println("Sum = " + ans); } public static void stackAddition() { theStack = new Stack(10000); ans = 0; while (num > 0) { theStack.push(num); --num; } while (!theStack.isEmpty()) { int newN = theStack.pop(); ans += newN; } } } class Stack { private int maxSize; private int[] data; private int top; public Stack(int s) { maxSize = s; data = new int[maxSize]; top = -1; } public void push(int p) { data[++top] = p; } public int pop() { return data[top--]; } public int peek() { return data[top]; } public boolean isEmpty() { return (top == -1); } }
结果
上面的代码示例会产生以下结果。
Sum = 1275
以下是从 1 到 n 的自然数的另一个示例
public class Demo { public static void main(String[] args) { int sum = 0; int n = 50; for (int i = 1; i <= n; i++) { sum = sum + i; } System.out.println("The Sum Of " + n + "is" + sum); } }
上面的代码示例会产生以下结果。
The Sum Of 50 is 1275
java_data_structure.htm
广告