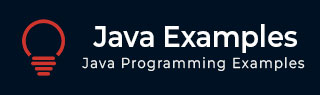
- Java 编程示例
- 示例 - 主页
- 示例 - 环境
- 示例 - 字符串
- 示例 - 数组
- 示例 - 日期和时间
- 示例 - 方法
- 示例 - 文件
- 示例 - 目录
- 示例 - 异常
- 示例 - 数据结构
- 示例 - 集合
- 示例 - 网络
- 示例 - 线程
- 示例 - 小程序
- 示例 - 简单图形用户界面
- 示例 - JDBC
- 示例 - 正则表达式
- 示例 - Apache PDF Box
- 示例 - Apache POI PPT
- 示例 - Apache POI Excel
- 示例 - Apache POI Word
- 示例 - OpenCV
- 示例 - Apache Tika
- 示例 - iText
- Java 教程
- Java - 教程
- Java 实用资源
- Java - 快速指南
- Java - 实用资源
如何使用框架在 Java 中显示饼状图
问题说明
如何使用框架显示饼状图?
解决方案
以下示例展示了如何通过制作“切片”类和根据切片创建弧来显示饼状图。
import java.awt.Color; import java.awt.Graphics; import java.awt.Graphics2D; import java.awt.Rectangle; import javax.swing.JComponent; import javax.swing.JFrame; class Slice { double value; Color color; public Slice(double value, Color color) { this.value = value; this.color = color; } } class MyComponent extends JComponent { Slice[] slices = { new Slice(5, Color.black), new Slice(33, Color.green), new Slice(20, Color.yellow), new Slice(15, Color.red) }; MyComponent() {} public void paint(Graphics g) { drawPie((Graphics2D) g, getBounds(), slices); } void drawPie(Graphics2D g, Rectangle area, Slice[] slices) { double total = 0.0D; for (int i = 0; i < slices.length; i++) { total += slices[i].value; } double curValue = 0.0D; int startAngle = 0; for (int i = 0; i < slices.length; i++) { startAngle = (int) (curValue * 360 / total); int arcAngle = (int) (slices[i].value * 360 / total); g.setColor(slices[i].color); g.fillArc(area.x, area.y, area.width, area.height, startAngle, arcAngle); curValue += slices[i].value; } } } public class Main { public static void main(String[] argv) { JFrame frame = new JFrame(); frame.getContentPane().add(new MyComponent()); frame.setSize(300, 200); frame.setVisible(true); } }
结果
上述代码示例将生成以下结果。
Displays a piechart in a frame.
以下是使用框架显示饼状图的一个示例。
import java.awt.Color; import java.awt.Graphics; import java.awt.Graphics2D; import java.awt.Rectangle; import javax.swing.JComponent; import javax.swing.JFrame; class Part { double value; Color color; public Part(double value, Color color) { this.value = value; this.color = color; } } class MyComponent extends JComponent { Part[] slices = { new Part(15, Color.yellow), new Part(30, Color.white), new Part(25, Color.blue), new Part(30, Color.red) }; MyComponent() { } public void paint(Graphics g) { drawPie((Graphics2D) g, getBounds(), slices); } void drawPie(Graphics2D g, Rectangle area, Part[] slices) { double total = 0.0D; for (int i = 0; i < slices.length; i++) { total += slices[i].value; } double curValue = 0.0D; int startAngle = 0; for (int i = 0; i < slices.length; i++) { startAngle = (int) (curValue * 360 / total); int arcAngle = (int) (slices[i].value * 360 / total); g.setColor(slices[i].color); g.fillArc(area.x, area.y, area.width, area.height, startAngle, arcAngle); curValue += slices[i].value; } } } public class Panel { public static void main(String[] argv) { JFrame frame = new JFrame(); frame.getContentPane().add(new MyComponent()); frame.setSize(300, 200); frame.setVisible(true); } }
java_simple_gui.htm
广告