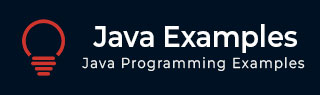
- Java 编程示例
- 示例 - 首页
- 示例 - 环境
- 示例 - 字符串
- 示例 - 数组
- 示例 - 日期和时间
- 示例 - 方法
- 示例 - 文件
- 示例 - 目录
- 示例 - 异常
- 示例 - 数据结构
- 示例 - 集合
- 示例 - 网络
- 示例 - 线程
- 示例 - 小程序
- 示例 - 简单 GUI
- 示例 - JDBC
- 示例 - 正则表达式
- 示例 - Apache PDF Box
- 示例 - Apache POI PPT
- 示例 - Apache POI Excel
- 示例 - Apache POI Word
- 示例 - OpenCV
- 示例 - Apache Tika
- 示例 - iText
- Java 教程
- Java - 教程
- Java 有用资源
- Java - 快速指南
- Java - 有用资源
如何用 Java 读取和下载网页
问题描述
如何读取和下载网页?
解决方案
以下示例展示如何读取和下载网址 net.URL 类的 URL() 构造器。
import java.io.BufferedReader; import java.io.BufferedWriter; import java.io.FileWriter; import java.io.InputStreamReader; import java.net.URL; public class Main { public static void main(String[] args) throws Exception { URL url = new URL("http://www.google.com"); BufferedReader reader = new BufferedReader(new InputStreamReader(url.openStream())); BufferedWriter writer = new BufferedWriter(new FileWriter("data.html")); String line; while ((line = reader.readLine()) != null) { System.out.println(line); writer.write(line); writer.newLine(); } reader.close(); writer.close(); } }
结果
上述代码示例将产生以下结果。
Welcome to Java Tutorial Here we have plenty of examples for you! Come and Explore Java!
以下是读取和下载网页的另一个示例。
import java.io.BufferedReader; import java.io.IOException; import java.io.InputStream; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.MalformedURLException; import java.net.URL; public class NewClass { public static void main(String[] args) { URL url; InputStream is = null; BufferedReader br; String line; try { url = new URL("https://tutorialspoint.com/javaexamples/net_singleuser.htm"); is = url.openStream(); // throws an IOException br = new BufferedReader(new InputStreamReader(is)); while ((line = br.readLine()) != null) { System.out.println(line); } } catch (MalformedURLException mue) { mue.printStackTrace(); } catch (IOException ioe) { ioe.printStackTrace(); } finally { try { if (is != null) is.close(); } catch (IOException ioe) {} } } }
结果
上述代码示例将产生以下结果。
<!DOCTYPE html> <!--[if IE 8]><html class="ie ie8"> <![endif]--> <!--[if IE 9]><html class="ie ie9"> <![endif]--> <!--[if gt IE 9]><!--> <html> <!--<![endif]--> <head> <!-- Basic --> <meta charset="utf-8"> <title>Java Examples - Socket to a single client</title> <meta name="description" content="Java Examples Socket to a single client : A beginner's tutorial containing complete knowledge of Java Syntax Object Oriented Language, Methods, Overriding, Inheritance, Polymorphism, Interfaces, Packages, Collections, Networking, Multithreading, Generics, Multimedia, Serialization, GUI." /> <meta name="keywords" content="Java, Tutorials, Learning, Beginners, Basics, Object Oriented Language, Methods, Overriding, Inheritance, Polymorphism, Interfaces, Packages, Collections, Networking, Multithreading, Generics, Multimedia, Serialization, GUI." /> <base href="https://tutorialspoint.com/" /> <link rel="shortcut icon" href="/favicon.ico" type="image/x-icon" /> <meta name="viewport" content="width=device-width,initial-scale=1.0,user-scalable=yes"> <meta property="og:locale" content="en_US" /> <meta property="og:type" content="website" /> <meta property="fb:app_id" content="471319149685276" /> <meta property="og:site_name" content="www.tutorialspoint.com" /> <meta name="robots" content="index, follow"/> <meta name="apple-mobile-web-app-capable" content="yes"> <meta name="apple-mobile-web-app-status-bar-style" content="black"> <meta name="author" content="tutorialspoint.com"> <script type="text/javascript" src="/theme/js/script-min-v4.js"></script>
java_networking.htm
广告