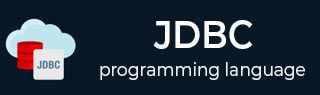
- JDBC 教程
- JDBC - 首页
- JDBC - 简介
- JDBC - SQL 语法
- JDBC - 环境配置
- JDBC - 示例代码
- JDBC - 驱动程序类型
- JDBC - 连接
- JDBC - 语句
- JDBC - 结果集
- JDBC - 数据类型
- JDBC - 事务
- JDBC - 异常
- JDBC - 批量处理
- JDBC - 存储过程
- JDBC - 流式数据
- JDBC - RowSet
- JDBC - 复制数据库
- JDBC - ACID 属性
- JDBC - 连接池
- JDBC 示例
- JDBC - 创建数据库
- JDBC - 选择数据库
- JDBC - 删除数据库
- JDBC - 创建表
- JDBC - 删除表
- JDBC - 插入记录
- JDBC - 查询记录
- JDBC - 更新记录
- JDBC - 删除记录
- JDBC - WHERE 子句
- JDBC - LIKE 子句
- JDBC - 数据排序
- JDBC 有用资源
- JDBC - 问答
- JDBC - 快速指南
- JDBC - 有用资源
- JDBC - 讨论
- 有用资源 - Java 教程
JDBC - 使用PreparedStatement对象的批量处理
以下是使用 PrepareStatement 对象进行批量处理的典型步骤:
创建带有占位符的 SQL 语句。
使用 `prepareStatement()` 方法创建 PrepareStatement 对象。
使用 `setAutoCommit()` 将自动提交设置为 false。
使用已创建的语句对象的 `addBatch()` 方法将任意数量的 SQL 语句添加到批处理中。
使用已创建的语句对象的 `executeBatch()` 方法执行所有 SQL 语句。
最后,使用 `commit()` 方法提交所有更改。
此示例代码是基于前面章节中完成的环境和数据库设置编写的。
JDBC 示例中将 PreparedStatement(INSERT)以自动提交为 False 的方式批量执行
在这个例子中,我们有三个静态字符串,包含数据库连接 URL、用户名和密码。我们定义了一个 `printResultSet()` 方法,它以结果集作为参数,迭代并打印结果集的所有记录。一旦所有更改提交后,`printResultSet()` 方法被调用以打印所有记录。
现在使用 `DriverManager.getConnection()` 方法,我们准备了一个数据库连接。使用 `setAutoCommit(false)`,我们将自动提交设置为 false,默认为 true。连接准备就绪后,我们使用 `connection.createPreparedStatement()` 方法创建了一个 PreparedStatement 对象,同时传递了一个带有占位符的 INSERT 查询。然后使用 `statement.executeQuery()`,所有记录都被提取并使用 `printResultSet()` 方法打印。
现在我们已经为 preparedStatement 设置了值,然后使用 `addBatch()` 方法将 preparedStatement 添加到批处理中。添加后,我们再次为 preparedStatement 对象设置值,并使用 `addBatch()` 方法将 preparedStatement 添加到批处理中。然后使用 `executeBatch()` 方法,我们一次性执行所有语句,并使用 `commit()` 方法提交更改。现在使用 `printResultSet()`,我们正在打印 Employees 表中可用的所有记录。
将以下示例复制并粘贴到 JDBCExample.java 中,编译并运行如下:
import java.sql.*; public class JDBCExample { static final String DB_URL = "jdbc:mysql://127.0.0.1/TUTORIALSPOINT"; static final String USER = "guest"; static final String PASS = "guest123"; public static void printResultSet(ResultSet rs) throws SQLException{ // Ensure we start with first row rs.beforeFirst(); while(rs.next()){ // Display values System.out.print("ID: " + rs.getInt("id")); System.out.print(", Age: " + rs.getInt("age")); System.out.print(", First: " + rs.getString("first")); System.out.println(", Last: " + rs.getString("last")); } System.out.println(); } public static void main(String[] args) { // Open a connection try(Connection conn = DriverManager.getConnection(DB_URL, USER, PASS); conn.setAutoCommit(false); // Create SQL statement String SQL = "INSERT INTO Employees(id,first,last,age) " + "VALUES(?, ?, ?, ?)"; // Create preparedStatement System.out.println("Creating statement..."); stmt = conn.prepareStatement(SQL); // print all the records ResultSet rs = stmt.executeQuery("Select * from Employees"); printResultSet(rs); // Set the variables stmt.setInt( 1, 400 ); stmt.setString( 2, "Pappu" ); stmt.setString( 3, "Singh" ); stmt.setInt( 4, 33 ); // Add it to the batch stmt.addBatch(); // Set the variables stmt.setInt( 1, 401 ); stmt.setString( 2, "Pawan" ); stmt.setString( 3, "Singh" ); stmt.setInt( 4, 31 ); // Add it to the batch stmt.addBatch(); // Create an int[] to hold returned values int[] count = stmt.executeBatch(); System.out.print("Batch Executed."); //Explicitly commit statements to apply changes conn.commit(); rs = stmt.executeQuery("Select * from Employees"); printResultSet(rs); stmt.close(); rs.close(); } catch (SQLException e) { e.printStackTrace(); } } }
现在让我们编译上面的示例,如下所示:
C:\>javac JDBCExample.java C:\>
运行 **JDBCExample** 时,会产生以下结果:
C:\>java JDBCExample Creating statement... ID: 95, Age: 20, First: Sima, Last: Chug ID: 100, Age: 35, First: Zara, Last: Ali ID: 101, Age: 25, First: Mahnaz, Last: Fatma ID: 102, Age: 30, First: Zaid, Last: Khan ID: 103, Age: 30, First: Sumit, Last: Mittal ID: 110, Age: 20, First: Sima, Last: Chug ID: 200, Age: 30, First: Zia, Last: Ali ID: 201, Age: 35, First: Raj, Last: Kumar Batch Executed. ID: 95, Age: 20, First: Sima, Last: Chug ID: 100, Age: 35, First: Zara, Last: Ali ID: 101, Age: 25, First: Mahnaz, Last: Fatma ID: 102, Age: 30, First: Zaid, Last: Khan ID: 103, Age: 30, First: Sumit, Last: Mittal ID: 110, Age: 20, First: Sima, Last: Chug ID: 200, Age: 30, First: Zia, Last: Ali ID: 201, Age: 35, First: Raj, Last: Kumar ID: 400, Age: 33, First: Pappu, Last: Singh ID: 401, Age: 31, First: Pawan, Last: Singh C:\>
JDBC 示例中将 PreparedStatement(UPDATE)以自动提交为 False 的方式批量执行
在这个例子中,我们有三个静态字符串,包含数据库连接 URL、用户名和密码。我们定义了一个 `printResultSet()` 方法,它以结果集作为参数,迭代并打印结果集的所有记录。一旦所有更改提交后,`printResultSet()` 方法被调用以打印所有记录。
现在使用 `DriverManager.getConnection()` 方法,我们准备了一个数据库连接。使用 `setAutoCommit(false)`,我们将自动提交设置为 false,默认为 true。连接准备就绪后,我们使用 `connection.createPreparedStatement()` 方法创建了一个 PreparedStatement 对象,同时传递了一个带有占位符的 UPDATE 查询。然后使用 `statement.executeQuery()`,所有记录都被提取并使用 `printResultSet()` 方法打印。
现在我们已经为 preparedStatement 设置了值,然后使用 `addBatch()` 方法将 preparedStatement 添加到批处理中。添加后,我们再次为 preparedStatement 对象设置值,并使用 `addBatch()` 方法将 preparedStatement 添加到批处理中。然后使用 `executeBatch()` 方法,我们一次性执行所有语句,并使用 `commit()` 方法提交更改。现在使用 `printResultSet()`,我们正在打印 Employees 表中可用的所有记录。
将以下示例复制并粘贴到 JDBCExample.java 中,编译并运行如下:
import java.sql.*; public class JDBCExample { static final String DB_URL = "jdbc:mysql://127.0.0.1/TUTORIALSPOINT"; static final String USER = "guest"; static final String PASS = "guest123"; public static void printResultSet(ResultSet rs) throws SQLException{ // Ensure we start with first row rs.beforeFirst(); while(rs.next()){ // Display values System.out.print("ID: " + rs.getInt("id")); System.out.print(", Age: " + rs.getInt("age")); System.out.print(", First: " + rs.getString("first")); System.out.println(", Last: " + rs.getString("last")); } System.out.println(); } public static void main(String[] args) { // Open a connection try(Connection conn = DriverManager.getConnection(DB_URL, USER, PASS); conn.setAutoCommit(false); // Create SQL statement String SQL = "update employees set age=50 where id=?"; // Create preparedStatement System.out.println("Creating statement..."); stmt = conn.prepareStatement(SQL); // print all the records ResultSet rs = stmt.executeQuery("Select * from Employees"); printResultSet(rs); stmt.setInt(1, 35); stmt.addBatch(); stmt.setInt(1, 36); stmt.addBatch(); stmt.setInt(1, 37); stmt.addBatch(); // Create an int[] to hold returned values int[] count = stmt.executeBatch(); System.out.print("Batch Executed."); //Explicitly commit statements to apply changes conn.commit(); rs = stmt.executeQuery("Select * from Employees"); printResultSet(rs); stmt.close(); rs.close(); } catch (SQLException e) { e.printStackTrace(); } } }
现在让我们编译上面的示例,如下所示:
C:\>javac JDBCExample.java C:\>
运行 **JDBCExample** 时,会产生以下结果:
C:\>java JDBCExample Creating statement... ID: 1, Age: 18, First: Zara, Last: Ali ID: 2, Age: 25, First: Mahnaz, Last: Fatma ID: 3, Age: 30, First: Zaid, Last: Khan ID: 4, Age: 28, First: Sumit, Last: Mittal ID: 7, Age: 20, First: Rita, Last: Tez ID: 8, Age: 20, First: Sita, Last: Singh ID: 21, Age: 35, First: Jeevan, Last: Rao ID: 22, Age: 40, First: Aditya, Last: Chaube ID: 25, Age: 35, First: Jeevan, Last: Rao ID: 26, Age: 35, First: Aditya, Last: Chaube ID: 34, Age: 45, First: Ahmed, Last: Ali ID: 35, Age: 31, First: Raksha, Last: Agarwal ID: 36, Age: 35, First: Sankalp, Last: Hawladar ID: 37, Age: 48, First: Anand, Last: Roy Batch Executed. ID: 1, Age: 18, First: Zara, Last: Ali ID: 2, Age: 25, First: Mahnaz, Last: Fatma ID: 3, Age: 30, First: Zaid, Last: Khan ID: 4, Age: 28, First: Sumit, Last: Mittal ID: 7, Age: 20, First: Rita, Last: Tez ID: 8, Age: 20, First: Sita, Last: Singh ID: 21, Age: 35, First: Jeevan, Last: Rao ID: 22, Age: 40, First: Aditya, Last: Chaube ID: 25, Age: 35, First: Jeevan, Last: Rao ID: 26, Age: 35, First: Aditya, Last: Chaube ID: 34, Age: 45, First: Ahmed, Last: Ali ID: 35, Age: 50, First: Raksha, Last: Agarwal ID: 36, Age: 50, First: Sankalp, Last: Hawladar ID: 37, Age: 50, First: Anand, Last: Roy C:\>