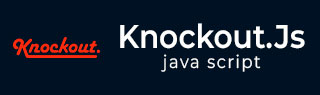
- KnockoutJS 教程
- KnockoutJS - 首页
- KnockoutJS - 概述
- KnockoutJS - 环境设置
- KnockoutJS - 应用
- KnockoutJS - MVVM 框架
- KnockoutJS - 可观察对象
- 计算可观察对象
- KnockoutJS - 声明式绑定
- KnockoutJS - 依赖项跟踪
- KnockoutJS - 模板
- KnockoutJS - 组件
- KnockoutJS 资源
- KnockoutJS - 快速指南
- KnockoutJS - 资源
- KnockoutJS - 讨论
KnockoutJS - 点击绑定
点击绑定是最简单的绑定之一,用于根据点击事件调用与 DOM 元素关联的 JavaScript 函数。此绑定类似于事件处理程序。
它最常与按钮、输入和a等元素一起使用,但实际上适用于任何可见的 DOM 元素。
语法
click: <binding-function>
参数
此处的参数将是一个 JavaScript 函数,需要根据点击事件调用。这可以是任何函数,不一定是 ViewModel 函数。
示例
让我们看下面的示例,它演示了点击绑定的用法。
<!DOCTYPE html> <head> <title>KnockoutJS Click Binding</title> <script src = "https://ajax.aspnetcdn.com/ajax/knockout/knockout-3.3.0.js" type = "text/javascript"></script> </head> <body> <p>Enter your name: <input data-bind = "value: someValue" /></p> <p><button data-bind = "click: showMessage">Click here</button></p> <script type = "text/javascript"> function ViewModel () { this.someValue = ko.observable(); this.showMessage = function() { alert("Hello "+ this.someValue()+ "!!! How are you today?"+ "\nClick Binding is used here !!!"); } }; var vm = new ViewModel(); ko.applyBindings(vm); </script> </body> </html>
输出
让我们执行以下步骤来了解上述代码的工作原理:
将上述代码保存在click-bind.htm文件中。
在浏览器中打开此 HTML 文件。
单击“点击此处”按钮,屏幕上将显示一条消息。
观察结果
还可以将当前项目作为参数传递
在调用处理程序函数时,还可以提供当前模型值作为参数。这在处理数据集合时非常有用,在数据集合中,需要对一组项目执行相同的操作。
示例
让我们看下面的示例来更好地理解它。
<!DOCTYPE html> <head> <title>KnockoutJS Click binding</title> <script src = "https://ajax.aspnetcdn.com/ajax/knockout/knockout-3.3.0.js" type = "text/javascript"></script> </head> <body> <p>List of product details:</p> <ul data-bind = "foreach: productArray "> <li> <span data-bind = "text: productName"></span> <a href = "#" data-bind = "click: $parent.removeProduct">Remove </a> </li> </ul> <script type = "text/javascript"> function AppViewModel() { self = this; self.productArray = ko.observableArray ([ {productName: 'Milk'}, {productName: 'Oil'}, {productName: 'Shampoo'} ]); self.removeProduct = function() { self.productArray.remove(this); } }; var vm = new AppViewModel(); ko.applyBindings(vm); </script> </body> </html>
输出
让我们执行以下步骤来了解上述代码的工作原理:
将上述代码保存在click-for-current-item.htm文件中。
在浏览器中打开此 HTML 文件。
每次单击“删除”链接时都会调用removeProduct函数,并为数组中的该特定项目调用。
请注意,使用$parent绑定上下文来访问处理程序函数。
传递更多参数
DOM 事件以及当前模型值也可以传递给处理程序函数。
示例
让我们看下面的示例来更好地理解它。
<!DOCTYPE html> <head> <title>KnockoutJS Click Binding</title> <script src = "https://ajax.aspnetcdn.com/ajax/knockout/knockout-3.3.0.js" type = "text/javascript"></script> </head> <body> <p>Press Control key + click below button.</p> <p><button data-bind = "click: showMessage">Click here to read message</button></p> <script type = "text/javascript"> function ViewModel () { this.showMessage = function(data,event) { alert("Click Binding is used here !!!"); if (event.ctrlKey) { alert("User was pressing down the Control key."); } } }; var vm = new ViewModel(); ko.applyBindings(vm); </script> </body> </html>
输出
让我们执行以下步骤来了解上述代码的工作原理:
将上述代码保存在click-bind-more-params.htm文件中。
在浏览器中打开此 HTML 文件。
此绑定捕获 Control 键的按下。
允许默认点击操作
默认情况下,KnockoutJS 会阻止点击事件执行任何默认操作。这意味着如果在<a>标签上使用点击绑定,则浏览器只会调用处理程序函数,而不会真正将您带到 href 中提到的链接。
如果希望在点击绑定中执行默认操作,则只需从处理程序函数中返回 true。
示例
让我们看下面的示例,它演示了点击绑定执行的默认操作。
<!DOCTYPE html> <head> <title>KnockoutJS Click Binding - allowing default action</title> <script src = "https://ajax.aspnetcdn.com/ajax/knockout/knockout-3.3.0.js" type = "text/javascript"></script> </head> <body> <a href = "https://tutorialspoint.com//" target = "_blank" data-bind = "click: callUrl">Click here to see how default Click binding works. </a> <script type = "text/javascript"> function ViewModel() { this.callUrl = function() { alert("Default action in Click Binding is allowed here !!! You are redirected to link."); return true; } }; var vm = new ViewModel(); ko.applyBindings(vm); </script> </body> </html>
输出
让我们执行以下步骤来了解上述代码的工作原理:
将上述代码保存在click-default-bind.htm文件中。
在浏览器中打开此 HTML 文件。
单击链接,屏幕上将显示一条消息。href 中提到的 URL 在新窗口中打开。
阻止事件冒泡
KO 将允许点击事件冒泡到更高级别的事件处理程序。这意味着如果您嵌套了两个点击事件,则将调用这两个事件的点击处理程序函数。如果需要,可以通过添加一个名为 clickBubble 的额外绑定并将 false 值传递给它来阻止此冒泡。
示例
让我们看下面的示例,它演示了 clickBubble 绑定的用法。
<!DOCTYPE html> <head> <title>KnockoutJS Click Binding - handling clickBubble</title> <script src = "https://ajax.aspnetcdn.com/ajax/knockout/knockout-3.3.0.js" type = "text/javascript"></script> </head> <body> <div data-bind = "click: outerFunction"> <button data-bind = "click: innerFunction, clickBubble:false"> Click me to see use of clickBubble. </button> </div> <script type = "text/javascript"> function ViewModel () { this.outerFunction = function() { alert("Handler function from Outer loop called."); } this.innerFunction = function() { alert("Handler function from Inner loop called."); } }; var vm = new ViewModel(); ko.applyBindings(vm); </script> </body> </html>
输出
让我们执行以下步骤来了解上述代码的工作原理:
将上述代码保存在click-cllickbubble-bind.htm文件中。
在浏览器中打开此 HTML 文件。
单击按钮,并观察将 clickBubble 绑定设置为 false 如何阻止事件传递给 innerFunction。