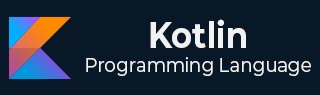
- Kotlin 教程
- Kotlin - 首页
- Kotlin - 概述
- Kotlin - 环境搭建
- Kotlin - 架构
- Kotlin - 基本语法
- Kotlin - 注释
- Kotlin - 关键字
- Kotlin - 变量
- Kotlin - 数据类型
- Kotlin - 运算符
- Kotlin - 布尔值
- Kotlin - 字符串
- Kotlin - 数组
- Kotlin - 范围
- Kotlin - 函数
- Kotlin 控制流
- Kotlin - 控制流
- Kotlin - if...Else 表达式
- Kotlin - When 表达式
- Kotlin - For 循环
- Kotlin - While 循环
- Kotlin - Break 和 Continue
- Kotlin 集合
- Kotlin - 集合
- Kotlin - 列表
- Kotlin - 集合
- Kotlin - 映射
- Kotlin 对象和类
- Kotlin - 类和对象
- Kotlin - 构造函数
- Kotlin - 继承
- Kotlin - 抽象类
- Kotlin - 接口
- Kotlin - 可见性控制
- Kotlin - 扩展
- Kotlin - 数据类
- Kotlin - 密封类
- Kotlin - 泛型
- Kotlin - 委托
- Kotlin - 解构声明
- Kotlin - 异常处理
- Kotlin 有用资源
- Kotlin - 快速指南
- Kotlin - 有用资源
- Kotlin - 讨论
Kotlin 数组 - indexOfFirst() 函数
Kotlin 数组 indexOfFirst() 函数用于返回 ArrayList 中第一个满足提供的断言的元素的索引。如果没有任何元素匹配提供的断言函数,则返回 -1。
第一个元素的索引表示,如果存在两个具有相同值的元素,此函数将返回第一个元素的索引。
语法
以下是 Kotlin 数组 indexOfFirst() 函数的语法:
fun <T> Array<out T>.indexOfFirst(predicate: (T) -> Boolean): Int
参数
此函数接受一个断言作为参数。断言表示一个给出布尔值的条件。
返回值
此函数返回第一个满足断言的元素的索引。否则返回 -1。
示例 1
以下是一个基本示例,用于演示 indexOfFirst() 函数的使用:
fun main(args: Array<String>) { var list = ArrayList<Int>() list.add(5) list.add(6) list.add(7) println("The ArrayList is $list") var firstIndex = list.indexOfFirst({it % 2 == 0 }) println("\nThe first index of even element is $firstIndex") }
输出
执行上述代码后,我们将获得以下结果:
The ArrayList is [5, 6, 7] The first index of even element is 1
示例 2
现在,让我们看另一个示例。在这里,我们使用indexOfFirst()函数来显示长度大于 5 的第一个元素的索引:
fun main(args: Array<String>) { // let's create an array var array = arrayOf("tutorialspoint", "India", "tutorix", "India") // Find the index of the first element with a length greater than 5 val indx = array.indexOfFirst { it.length > 5 } // Print the array elements println("Array: [${array.joinToString { "$it" }}]") if (indx != -1) { println("The first element with length more than 5 is at index $indx, which is \"${array[indx]}\" with length ${array[indx].length}") } else { println("No element has length more than 5.") } }
输出
执行上述代码后,我们将获得以下输出:
Array: [tutorialspoint, India, tutorix, India] The first element with length more than 5 is at index 0, which is "tutorialspoint" with length 14
示例 3
下面的示例创建一个数组。然后我们使用indexOfFirst函数来显示第一个能被 3 整除的元素的索引:
fun main(args: Array<String>){ // let's create an array var array = arrayOf<Int>(1, 2, 3, 6, 4, 5) // using indexOfFirst val indx = array.indexOfFirst({it%3==0}) if(indx == -1){ println("The element is not available in the array!") }else{ println("The element which is divisible by 3 is ${array[indx]} at index: $indx") } }
输出
上述代码将产生以下输出:
The element which is divisible by 3 is 3 at index: 2
kotlin_arrays.htm
广告