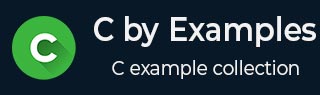
- 用示例学 C 语言时间
- 用示例学 C 语言 - 主页
- C 语言示例 - 简单程序
- C 语言示例 - 循环/迭代
- C 语言示例 - 模式
- C 语言示例 - 数组
- C 语言示例 - 字符串
- C 语言示例 - 数学
- C 语言示例 - 链表
- C 语言编程有用资源
- 用示例学 C 语言 - 快速入门指南
- 用示例学 C 语言 - 资源
- 用示例学 C 语言 - 讨论
C 语言中的变量
变量是某一值的一个占位符。所有变量都有一些与其关联的类型,该类型表示可以为其分配的“类型”的值。C 语言提供了一组丰富的变量 −
类型 | 格式字符串 | 说明 |
---|---|---|
char | %c | 字符类型变量(ASCII 值) |
int | %d | 机器中最自然的整数大小。 |
float | %f | 单精度浮点数。 |
double | %e | 双精度浮点数。 |
void | − N/A − | 表示不存在类型。 |
C 语言中的字符 (char
) 变量
字符 (char
) 变量保存一个单个字符。
#include <stdio.h> int main() { char c; // char variable declaration c = 'A'; // defining a char variable printf("value of c is %c", c); return 0; }
该程序的输出应为 −
value of c is A
C 语言中的整数 (int
) 变量
int
变量保存单个字符的有符号整数值。
#include <stdio.h> int main() { int i; // integer variable declaration i = 123; // defining integer variable printf("value of i is %d", i); return 0; }
该程序的输出应为 −
value of i is 123
C 语言中的浮点数 (float
) 变量
float
变量保存单精度浮点数。
#include <stdio.h> int main() { float f; // floating point variable declaration f = 12.001234; // defining float variable printf("value of f is %f", f); return 0; }
该程序的输出应为 −
value of f is 12.001234
C 语言中的双精度 (double
) 浮点数变量
double
变量保存双精度浮点数。
#include <stdio.h> int main() { double d; // double precision variable declaration d = 12.001234; // defining double precision variable printf("value of d is %e", d); return 0; }
该程序的输出应为 −
value of d is 1.200123e+01
C 语言中的 void (void
) 数据类型
C 语言中的 void
表示“无”或“无值”。它与指针声明或函数声明一同使用。
// declares function which takes no arguments but returns an integer value int status(void) // declares function which takes an integer value but returns nothing void status(int) // declares a pointer p which points to some unknown type void * p
simple_programs_in_c.htm
广告