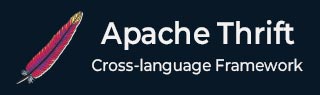
- Apache Thrift 教程
- Apache Thrift - 首页
- Apache Thrift - 简介
- Apache Thrift – 安装
- Apache Thrift - IDL
- Apache Thrift - 代码生成
- Apache Thrift - 服务实现
- Apache Thrift - 服务运行
- Apache Thrift - 传输和协议层
- Apache Thrift - 序列化
- Apache Thrift - 反序列化
- Apache Thrift - 负载均衡
- Apache Thrift - 服务发现
- Apache Thrift - 安全性考虑
- Apache Thrift - 跨语言兼容性
- Apache Thrift - 微服务架构
- Apache Thrift - 测试和调试
- Apache Thrift - 性能优化
- Apache Thrift - 案例研究
- Apache Thrift - 结论
- Apache Thrift 有用资源
- Apache Thrift - 有用资源
- Apache Thrift - 讨论
Apache Thrift - 微服务架构
Thrift 中的微服务架构
微服务架构是一种设计模式,其中应用程序由小型、独立的服务组成,这些服务通过网络进行通信。每个服务负责一项特定功能,可以独立开发、部署和扩展。
微服务架构的优势
以下是 Apache Thrift 中微服务架构的优势:
- 可扩展性:可以根据需求独立扩展服务。
- 灵活性:可以使用不同的技术和语言来构建不同的服务。
- 弹性:一个服务的故障不会必然影响其他服务。
- 更快的开发速度:团队可以同时处理不同的服务,从而加快开发速度。
Apache Thrift 在微服务中的作用
Apache Thrift 通过提供一个框架来定义服务、数据类型和通信协议(以与语言无关的方式),从而促进微服务的开发。它允许用不同语言编写的服务有效地相互通信。
Apache Thrift 通过提供以下功能在微服务架构中扮演着重要的角色:
- 跨语言通信:使用不同语言编写的服务能够使用公共协议进行通信。
- 高效的序列化:将数据转换为可以跨网络传输的格式,并在接收端重建数据。
- 灵活的协议和传输:支持各种协议(例如,二进制、紧凑型)和传输(例如,TCP、HTTP)进行通信。
使用 Thrift 设计微服务架构
使用 Thrift 设计微服务架构涉及使用 Thrift 的接口定义语言 (IDL) 定义服务,以指定数据结构和服务接口,然后生成各种编程语言的代码来实现这些服务。
这种方法可以轻松实现不同语言编写的服务之间的通信,从而确保高效的微服务环境。
使用 Thrift IDL 定义服务
设计微服务架构的第一步是使用 Thrift 的接口定义语言 (IDL) 定义服务。这涉及指定数据类型和服务接口。
IDL 定义示例
以下是一个 Thrift IDL 文件示例,其中定义了一个 "UserService" 服务。"User" 结构体定义了一个具有 "userId" 和 "username" 的用户;"UserService" 服务提供获取和更新用户的方法:
namespace py example namespace java example struct User { 1: string userId 2: string userName } service UserService { User getUser(1: string userId), void updateUser(1: User user) } service OrderService { void placeOrder(1: string userId, 2: string productId) string getOrderStatus(1: string orderId) }
为微服务生成代码
在 Thrift IDL 中定义服务后,需要为微服务中使用的语言生成代码:
- 创建 Thrift IDL 文件:在一个 ".thrift" 文件中编写服务和数据结构定义。
- 运行 Thrift 编译器:使用 Thrift 编译器为所需的语言生成代码。
- 实现服务:使用生成的代码在您选择的编程语言中实现服务逻辑。
要生成 Python 代码,请使用带有--gen选项的 Thrift 编译器。此命令创建一个 Python 模块,其中包含基于 IDL 定义的类和方法:
thrift --gen py microservices.thrift
同样,您可以使用--gen选项生成 Java 代码。此命令创建一个包含基于 IDL 定义的类和方法的 Java 包:
thrift --gen java microservices.thrift
实现微服务
使用生成的代码,现在可以以不同的语言实现微服务。在这里,我们将介绍两个示例微服务的实现:"UserService"(Python)和 "OrderService"(Java)。
Python 实现
以下是使用 Python 实现 "UserService" 的分步说明:
导入必要的模块
- TSocket, TTransport:用于处理网络通信。
- TBinaryProtocol:用于序列化和反序列化数据。
- UserService:Thrift 生成的服务定义。
定义服务处理程序
- "UserServiceHandler" 实现 "UserService.Iface" 接口。
- getUser(self, userId):一个用于检索用户信息的方法。它返回一个用户名为“Alice”的虚拟用户。
- updateUser(self, user):一个用于更新用户信息的方法。当用户被更新时,它会打印一条消息。
设置服务器
- TSocket.TServerSocket(port=9090):设置服务器以侦听端口 9090。
- TTransport.TBufferedTransportFactory():使用缓冲传输进行高效通信。
- TBinaryProtocol.TBinaryProtocolFactory():使用二进制协议进行数据序列化。
启动服务器
- TServer.TSimpleServer:一个简单的单线程服务器,一次处理一个请求。
- server.serve():启动服务器以接受和处理传入的请求。
在这个例子中,我们使用 Python 实现 "UserService"。此服务处理与用户相关的操作,例如检索和更新用户信息:
from thrift.server import TServer from thrift.transport import TSocket, TTransport from thrift.protocol import TBinaryProtocol from example import UserService class UserServiceHandler(UserService.Iface): def getUser(self, userId): # Implement user retrieval logic return User(userId=userId, userName="Alice") def updateUser(self, user): # Implement user update logic print(f"User updated: {user.userName}") # Create the handler instance handler = UserServiceHandler() # Create a processor using the handler processor = UserService.Processor(handler) # Set up the server transport (listening port) transport = TSocket.TServerSocket(port=9090) # Set up the transport factory for buffering tfactory = TTransport.TBufferedTransportFactory() # Set up the protocol factory for binary protocol pfactory = TBinaryProtocol.TBinaryProtocolFactory() # Create and start the server server = TServer.TSimpleServer(processor, transport, tfactory, pfactory) print("Starting UserService server...") server.serve()
Java 实现
类似地,现在让我们使用 Java 实现 "OrderService"。此服务处理与订单相关的操作,例如下单和检索订单状态:
import example.OrderService; import org.apache.thrift.TException; import org.apache.thrift.protocol.TBinaryProtocol; import org.apache.thrift.server.TServer; import org.apache.thrift.server.TSimpleServer; import org.apache.thrift.transport.TServerSocket; import org.apache.thrift.transport.TServerTransport; public class OrderServiceHandler implements OrderService.Iface { @Override public void placeOrder(String userId, String productId) throws TException { // Implement order placement logic System.out.println("Order placed for user " + userId + " and product " + productId); } @Override public String getOrderStatus(String orderId) throws TException { // Implement order status retrieval logic return "Order status for " + orderId; } public static void main(String[] args) { try { // Create the handler instance OrderServiceHandler handler = new OrderServiceHandler(); // Create a processor using the handler OrderService.Processor processor = new OrderService.Processor(handler); // Set up the server transport (listening port) TServerTransport serverTransport = new TServerSocket(9091); // Set up the protocol factory for binary protocol TBinaryProtocol.Factory protocolFactory = new TBinaryProtocol.Factory(); // Create and start the server TSimpleServer server = new TSimpleServer(new TServer.Args(serverTransport).processor(processor).protocolFactory(protocolFactory)); System.out.println("Starting OrderService server..."); server.serve(); } catch (Exception e) { e.printStackTrace(); } } }
使用 Thrift 管理微服务
使用 Thrift 管理微服务包括管理服务注册、发现、负载均衡和监控,以确保微服务架构的轻松操作和可扩展性。
服务发现
服务发现涉及在分布式环境中动态定位服务。Consul、Eureka 或 Zookeeper 等工具可以与 Thrift 一起使用来管理服务注册和发现。
负载均衡
负载均衡将传入请求分发到服务的多个实例,以确保负载均匀和高可用性。这可以使用 HAProxy、Nginx 或 AWS Elastic Load Balancing 等云端解决方案来实现。
监控和日志记录
实现监控和日志记录以跟踪微服务的运行状况和性能。Prometheus、Grafana 和 ELK Stack(Elasticsearch、Logstash、Kibana)等工具可用于收集和可视化指标和日志。