找到 2637 篇文章 关于 Java
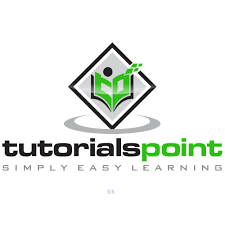
166 次查看
子表达式/元字符“\d”匹配数字。示例 1 import java.util.regex.Matcher; import java.util.regex.Pattern; public class RegexExample { public static void main( String args[] ) { String regex = "\d 24"; String input = "This is sample text 12 24 56 89 24"; Pattern p = Pattern.compile(regex); Matcher m = p.matcher(input); int count = 0; while(m.find()) { count++; } System.out.println("Number of matches: "+count); } }输出匹配数:2示例 2 以下是一个 Java 程序…… 阅读更多
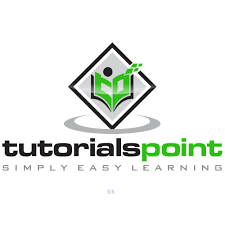
608 次查看
子表达式/元字符“\S”匹配非空白字符。示例 1 import java.util.regex.Matcher; import java.util.regex.Pattern; public class RegexExample { public static void main( String args[] ) { String regex = "\S"; String input = "Hello how are you welcome to Tutorialspoint !"; Pattern p = Pattern.compile(regex); Matcher m = p.matcher(input); int count = 0; while(m.find()) { count++; } System.out.println("Number of matches: "+count); } }输出匹配数:38示例 2 import java.util.Scanner; import java.util.regex.Matcher; import java.util.regex.Pattern; ... 阅读更多
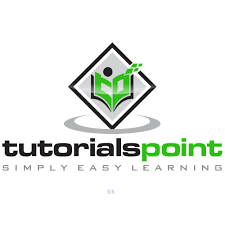
124 次查看
子表达式/元字符“\s”匹配空白字符等价物。示例 1 import java.util.regex.Matcher; import java.util.regex.Pattern; public class RegexExample { public static void main( String args[] ) { String regex = "\s"; String input = "Hello how are you welcome to Tutorialspoint !"; Pattern p = Pattern.compile(regex); Matcher m = p.matcher(input); int count = 0; while(m.find()) { count++; } System.out.println("Number of matches: "+count); } }输出匹配数:7示例 2 以下示例读取一个字符串…… 阅读更多
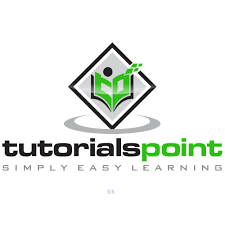
1K+ 次查看
子表达式/元字符“\W”匹配非单词字符。示例 1 import java.util.regex.Matcher; import java.util.regex.Pattern; public class RegexExample { public static void main(String args[]) { String regex = "\W!"; String input = "Hello how are you welcome to Tutorialspoint !"; Pattern p = Pattern.compile(regex); Matcher m = p.matcher(input); int count = 0; while(m.find()) { count++; } System.out.println("Number of matches: "+count); } }输出匹配数:1示例 2 以下示例读取 5 个字符串值并打印包含…… 阅读更多
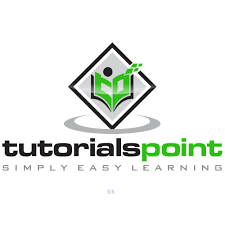
185 次查看
子表达式/元字符“\w”匹配单词字符,即 a 到 z 和 A 到 Z 以及 0 到 9。示例 1 import java.util.regex.Matcher; import java.util.regex.Pattern; public class RegexExample { public static void main( String args[] ) { String regex = "\w to"; String input = "Hello how are you welcome to Tutorialspoint"; Pattern p = Pattern.compile(regex); Matcher m = p.matcher(input); int count = 0; while(m.find()) { count++; } System.out.println("Number of matches: "+count); ... 阅读更多
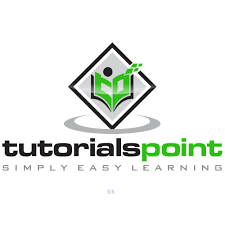
144 次查看
子表达式/元字符“[^...]”匹配任何不在括号中的单个字符。示例 1 import java.util.regex.Matcher; import java.util.regex.Pattern; public class SpecifiedCharacters { public static void main( String args[] ) { String regex = "[^hwtyoupi]"; String input = "Hi how are you welcome to Tutorialspoint"; Pattern p = Pattern.compile(regex); Matcher m = p.matcher(input); int count = 0; while(m.find()) { count++; } System.out.println("Number of matches: "+count); } }输出匹配数:21示例 2 以下 Java 程序接受…… 阅读更多
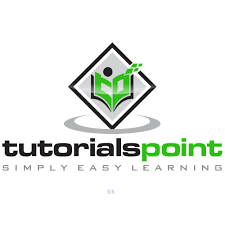
116 次查看
子表达式/元字符“re*”匹配前一个表达式的 0 个或多个出现。示例 1 import java.util.regex.Matcher; import java.util.regex.Pattern; public class RegexExample { public static void main( String args[] ) { String regex = "aabc*"; String input = "aabcabcaabcabbcaabcbcaabc"; Pattern p = Pattern.compile(regex); Matcher m = p.matcher(input); int count = 0; while(m.find()) { count++; } System.out.println("Number of matches: "+count); } }输出匹配数:4示例 2 import java.util.Scanner; import java.util.regex.Matcher; import java.util.regex.Pattern; public class MatchAllCharacters ... 阅读更多

2K+ 次查看
@ConstructorProperties 注解来自 java.beans 包,用于通过带注解的构造函数将 JSON 反序列化为 Java 对象。此注解从 Jackson 2.7 版本开始支持。此注解的工作方式非常简单,与其在构造函数中为每个参数添加注解,不如提供一个包含每个构造函数参数的属性名称的数组。语法@Documented @Target(value=CONSTRUCTOR) @Retention(value=RUNTIME) public @interface ConstructorProperties示例 import com.fasterxml.jackson.databind.ObjectMapper; import java.beans.ConstructorProperties; public class ConstructorPropertiesAnnotationTest { public static void main(String args[]) throws Exception { ObjectMapper mapper = new ObjectMapper(); Employee emp = new Employee(115, "Raja"); String jsonString = mapper.writerWithDefaultPrettyPrinter().writeValueAsString(emp); ... 阅读更多
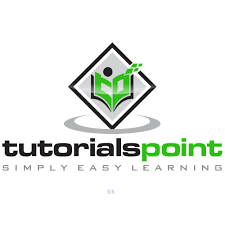
188 次查看
子表达式/元字符“\A”匹配整个字符串的开头。示例 1 import java.util.regex.Matcher; import java.util.regex.Pattern; public class RegexExample { public static void main( String args[] ) { String regex = "\AHi"; String input = "Hi how are you welcome to Tutorialspoint"; Pattern p = Pattern.compile(regex); Matcher m = p.matcher(input); int count = 0; while(m.find()) { count++; } System.out.println("Number of matches: "+count); } }输出匹配数:1示例 2 以下 Java 程序接受一个字符串…… 阅读更多
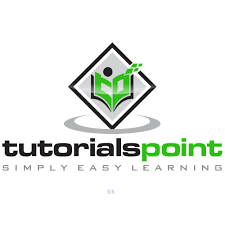
1K+ 次查看
子表达式/元字符“.”匹配除换行符之外的任何单个字符。示例1import java.util.regex.Matcher; import java.util.regex.Pattern; public class MatchesAll { public static void main( String args[] ) { String regex = "."; String input = "Hi how are you welcome to Tutorialspoint"; Pattern p = Pattern.compile(regex); Matcher m = p.matcher(input); int count = 0; while(m.find()) { count++; } System.out.println("Number of matches: "+count); } }输出匹配数:40示例 2以下Java程序接受5个... 阅读更多