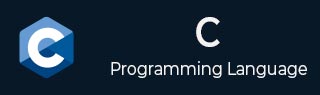
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - fclose() 函数
C 库 fclose() 函数用于关闭打开的文件流。它关闭流,并刷新所有缓冲区。
语法
以下为 C 库中 fclose() 函数的语法 −
int fclose(FILE *stream);
参数
此函数只接受一个参数 −
- *FILE stream: 用于标识要关闭的流的 FILE 对象的指针。此指针由以下函数获取:fopen、freopen 或 tmpfile。
返回值
如果成功关闭文件,则函数返回 0。如果关闭文件时发生错误,则返回 EOF(通常为 -1)。设置 errno 变量来指示错误。
示例 1:写入数据后关闭文件
此示例展示如何使用 fclose 向文件中写入数据,然后关闭文件。
以下是 C 库 fclose() 函数的演示。
#include <stdio.h> int main() { FILE *file = fopen("example1.txt", "w"); if (file == NULL) { perror("Error opening file"); return 1; } fprintf(file, "Hello, World!\n"); if (fclose(file) == EOF) { perror("Error closing file"); return 1; } printf("File closed successfully.\n"); return 0; }
输出
以上代码产生以下结果−
File closed successfully.
示例 2:处理文件关闭错误
此示例处理关闭文件失败的情况,演示了适当的错误处理。
#include <stdio.h> #include <errno.h> int main() { FILE *file = fopen("example3.txt", "w"); if (file == NULL) { perror("Error opening file"); return 1; } // Intentionally causing an error for demonstration (rare in practice) if (fclose(file) != 0) { perror("Error closing file"); return 1; } // Trying to close the file again to cause an error if (fclose(file) == EOF) { perror("Error closing file"); printf("Error code: %d\n", errno); return 1; } return 0; }
输出
执行以上代码后,我们得到以下结果 −
Error closing file: Bad file descriptor Error code: 9
广告