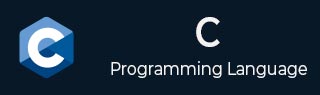
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - fgetc() 函数
C 库的 fgetc() 函数从指定的流中获取下一个字符(一个无符号字符),并推进流的位置指示器。它通常用于从文件中读取字符。
语法
以下是 C 库 fgetc() 函数的语法:
int fgetc(FILE *stream);
参数
stream : 指向 FILE 对象的指针,该对象标识输入流。此流通常通过使用 fopen 函数打开文件来获取。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
返回值
该函数返回从流中读取的字符,作为转换为 int 类型的无符号字符。如果遇到文件结尾或发生错误,则该函数返回 EOF,它是一个通常定义为 -1 的宏。为了区分实际字符和 EOF 返回值,应该使用 feof 或 ferror 函数来检查是否已到达文件结尾或发生错误。
示例 1
此示例读取并打印“example1.txt”的第一个字符。如果文件为空或发生错误。
#include <stdio.h> int main() { FILE *file = fopen("example1.txt", "r"); if (file == NULL) { perror("Error opening file"); return 1; } int ch = fgetc(file); if (ch != EOF) { printf("The first character is: %c\n", ch); } else { printf("No characters to read or error reading file\n"); } fclose(file); return 0; }
输出
以上代码产生以下结果:
The first character is: H
示例 2:统计文件中的字符数
此示例统计并打印“example3.txt”中的字符总数。
#include <stdio.h> int main() { FILE *file = fopen("example3.txt", "r"); if (file == NULL) { perror("Error opening file"); return 1; } int ch; int count = 0; while ((ch = fgetc(file)) != EOF) { count++; } printf("Total number of characters: %d\n", count); fclose(file); return 0; }
输出
执行上述代码后,我们得到以下结果:
Total number of characters: 57
广告