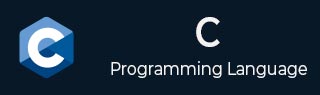
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - fscanf() 函数
C 库函数 int fscanf(FILE *stream, const char *format, ...) 从流中读取格式化输入。它是标准 I/O 库的一部分,并在 <stdio.h> 头文件中定义。此函数允许根据指定的格式提取和解析文件中的数据。
语法
以下是 C 库函数 fscanf() 的语法:
int fscanf(FILE *stream, const char *format, ...);
参数
此函数使用各种参数来处理文件 -
- *FILE stream : 指向 FILE 对象的指针,该对象标识要从中读取数据的输入流。
- *const char format:包含格式字符串的 C 字符串,格式字符串由转换说明符组成。这些说明符确定要读取的数据的类型和格式。
- ... (省略号):表示可变数量的其他参数,这些参数指向将存储解析值的地址。每个参数都必须与格式字符串中的一个格式说明符对应。
序号 | 参数及描述 |
---|---|
1 |
* 可选的起始星号表示数据要从流中读取但要忽略,即它不会存储在相应的参数中。 |
2 |
宽度 指定当前读取操作中要读取的最大字符数。 |
3 |
修饰符 指定与对应附加参数指向的数据不同的 int 大小(在 d、i 和 n 的情况下)、unsigned int 大小(在 o、u 和 x 的情况下)或 float 大小(在 e、f 和 g 的情况下):h:short int(对于 d、i 和 n),或 unsigned short int(对于 o、u 和 x)l:long int(对于 d、i 和 n),或 unsigned long int(对于 o、u 和 x),或 double(对于 e、f 和 g)L:long double(对于 e、f 和 g) |
4 |
类型 指定要读取的数据类型以及预期读取方式的字符。请参阅下表。 |
类型 | 限定输入 | 参数类型 |
---|---|---|
c | 单个字符:读取下一个字符。如果指定了与 1 不同的宽度,则该函数将读取宽度个字符并将它们存储在作为参数传递的数组的连续位置中。末尾不附加空字符。 | char * |
d | 十进制整数:前面可选带 + 或 - 号的数字 | int * |
e、E、f、g、G | 浮点数:包含小数点的十进制数,前面可选带 + 或 - 号,后面可选带 e 或 E 字符和十进制数。两个有效条目的示例是 -732.103 和 7.12e4 | float * |
o | 八进制整数 | int * |
s | 字符字符串。这将读取后续字符,直到找到空格(空格字符被认为是空格、换行符和制表符)。 | char * |
u | 无符号十进制整数。 | unsigned int * |
x、X | 十六进制整数 | int * |
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
返回值
fscanf 函数返回一个整数,表示成功匹配和赋值的输入项数。如果输入在第一次匹配失败之前结束或发生错误,则返回 EOF(文件结束)。
示例 1:读取整数和字符串
以下代码从文件中读取整数和字符串并打印它们。
以下是 C 库函数 fscanf() 的示例。
#include <stdio.h> #include <stdlib.h> int main() { const char *filename = "example1.txt"; FILE *file = fopen(filename, "r"); // If the file does not exist, create and write initial data to it if (file == NULL) { file = fopen(filename, "w"); if (file == NULL) { perror("Error creating file"); return 1; } fprintf(file, "123 HelloWorld\n"); fclose(file); file = fopen(filename, "r"); if (file == NULL) { perror("Error opening file"); return 1; } } int number; char word[50]; if (fscanf(file, "%d %49s", &number, word) == 2) { printf("Number: %d, Word: %s\n", number, word); } else { printf("Failed to read data from file.\n"); } fclose(file); return 0; }
输出
以上代码产生以下结果:
Number: 123, Word: HelloWorld
示例 2:读取多个浮点数
以下代码从文件中读取三个浮点数并打印它们。
#include <stdio.h> #include <stdlib.h> int main() { const char *filename = "example2.txt"; FILE *file = fopen(filename, "r"); // If the file does not exist, create and write initial data to it if (file == NULL) { file = fopen(filename, "w"); if (file == NULL) { perror("Error creating file"); return 1; } fprintf(file, "3.14 2.718 1.618\n"); fclose(file); file = fopen(filename, "r"); if (file == NULL) { perror("Error opening file"); return 1; } } float num1, num2, num3; if (fscanf(file, "%f %f %f", &num1, &num2, &num3) == 3) { if (fscanf(file, "%f %f %f", &num1, &num2, &num3) == 3) { printf("Numbers: %.2f, %.2f, %.2f\n", num1, num2, num3); } else { printf("Failed to read data from file.\n"); } fclose(file); return 0; }
输出
执行以上代码后,我们将得到以下结果:
Numbers: 3.14, 2.72, 1.62
示例 3:从文件读取日期
以下代码读取 DD/MM/YYYY 格式的日期并将其以 DD-MM-YYYY 格式打印。
#include <stdio.h> #include <stdlib.h> int main() { const char *filename = "example3.txt"; FILE *file = fopen(filename, "r"); // If the file does not exist, create and write initial data to it if (file == NULL) { file = fopen(filename, "w"); if (file == NULL) { perror("Error creating file"); return 1; } fprintf(file, "23/05/2024\n"); fclose(file); file = fopen(filename, "r"); if (file == NULL) { perror("Error opening file"); return 1; } } int day, month, year; if (fscanf(file, "%d/%d/%d", &day, &month, &year) == 3) { printf("Date: %02d-%02d-%04d\n", day, month, year); } else { printf("Failed to read data from file.\n"); } fclose(file); return 0; }
输出
以上代码的输出如下:
Date: 23-05-2024