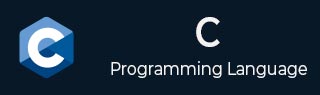
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - va_end() 宏
C 的stdarg 库 va_end() 宏用于执行对由va_start 或 va_copy 初始化的ap 对象(类型为 va_list 的变量)的清理。它可能会修改 ap,使其不再可用。
在使用使用va_start 或“va_copy”的函数之后,应调用va_end。它不能在没有相应的“va_start”或“va_copy”的情况下调用。这样做会导致未定义的行为。
此宏用于内存管理,并进一步防止内存泄漏。
语法
以下是 va_end() 宏的 C 库语法:
void va_end(va_list ap)
参数
此宏接受单个参数:
-
ap - 这是一个 va_list 类型的变量,它将由“va_start”初始化。此变量用于遍历参数列表。
返回值
此宏不返回值。
示例 1
以下是演示 va_end() 用法的基本 c 示例。
#include <stdio.h> #include <stdarg.h> int sum(int count, ...) { va_list args; int tot = 0; // Set the va_list variable with the last fixed argument va_start(args, count); // Retrieve the arguments and calculate the sum for (int i = 0; i < count; i++) { tot = tot + va_arg(args, int); } // use the va_end to clean va_list variable va_end(args); return tot; } int main() { // Call the sum, with number of arguments printf("Sum of 3, 5, 7, 9: %d\n", sum(4, 3, 5, 7, 9)); printf("Sum of 1, 2, 3, 4, 5: %d\n", sum(5, 1, 2, 3, 4, 5)); return 0; }
输出
以下是输出:
Sum of 3, 5, 7, 9: 24 Sum of 1, 2, 3, 4, 5: 15
示例 2:计算参数个数
以下 c 示例演示了在可变参数函数中使用 va_end()。
#include <stdio.h> #include <stdarg.h> int cnt(int count, ...) { va_list args; int num_of_arg = 0; // Set the va_list variable with the last fixed argument va_start(args, count); // Retrieve the arguments and count the arguments for (int i = 0; i < count; i++) { num_of_arg= num_of_arg + 1; } // use the va_end to clean va_list variable va_end(args); return num_of_arg; } int main() { // Call the sum, with number of arguments printf("Number of arguments: %d\n", cnt(4, 3, 5, 7, 9)); printf("Number of arguments: %d\n", cnt(5, 1, 2, 3, 4, 5)); return 0; }
输出
以下是输出:
Number of arguments: 4 Number of arguments: 5
示例 3:查找最大数
让我们看另一个例子,我们使用 va_end() 在找到最大数后清理 va_list 变量。
#include <stdarg.h> #include <stdio.h> int Largest_num(int n, ...) { va_list ap; va_start(ap, n); int max = va_arg(ap, int); for (int i = 0; i < n - 1; i++) { int temp = va_arg(ap, int); max = temp > max ? temp : max; } // use va_end to clean up va_list variable va_end(ap); return max; } int main() { printf("%d\n", Largest_num(2, 1, 2)); printf("%d\n", Largest_num(3, 1, 2, 3)); printf("%d\n", Largest_num(4, 6, 2, 3, 4)); return 0; }
输出
以下是输出:
2 3 6
广告