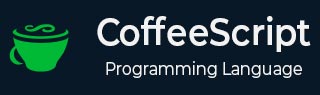
- CoffeeScript 教程
- CoffeeScript - 首页
- CoffeeScript - 概述
- CoffeeScript - 环境
- CoffeeScript - 命令行工具
- CoffeeScript - 语法
- CoffeeScript - 数据类型
- CoffeeScript - 变量
- CoffeeScript - 运算符和别名
- CoffeeScript - 条件语句
- CoffeeScript - 循环
- CoffeeScript - 列表推导式
- CoffeeScript - 函数
- CoffeeScript 面向对象
- CoffeeScript - 字符串
- CoffeeScript - 数组
- CoffeeScript - 对象
- CoffeeScript - 范围
- CoffeeScript - 展开运算符
- CoffeeScript - 日期
- CoffeeScript - 数学
- CoffeeScript - 异常处理
- CoffeeScript - 正则表达式
- CoffeeScript - 类和继承
- CoffeeScript 高级
- CoffeeScript - Ajax
- CoffeeScript - jQuery
- CoffeeScript - MongoDB
- CoffeeScript - SQLite
- CoffeeScript 有用资源
- CoffeeScript - 快速指南
- CoffeeScript - 有用资源
- CoffeeScript - 讨论
CoffeeScript - 位运算符
CoffeeScript 支持以下位运算符。假设变量A 为2,变量B 为3,则:
序号 | 运算符和描述 | 示例 |
---|---|---|
1 | & (按位与) 它对每个整数参数的每一位执行布尔与运算。 |
(A & B) 为 2。 |
2 | | (按位或) 它对每个整数参数的每一位执行布尔或运算。 |
(A | B) 为 3。 |
3 | ^ (按位异或) 它对每个整数参数的每一位执行布尔异或运算。异或意味着操作数一或操作数二为真,但不能同时为真。 |
(A ^ B) 为 1。 |
4 | ~ (按位非) 这是一个一元运算符,通过反转操作数中的所有位来操作。 |
(~B) 为 -4。 |
5 | << (左移) 它将第一个操作数中的所有位向左移动第二个操作数指定的位数。新的位用零填充。将值左移一位相当于将其乘以 2,左移两位相当于将其乘以 4,依此类推。 |
(A << 1) 为 4。 |
6 | >> (右移) 二进制右移运算符。左操作数的值向右移动右操作数指定的位数。 |
(A >> 1) 为 1。 |
示例
以下示例演示了在 CoffeeScript 中使用位运算符的方法。将此代码保存在名为bitwise_example.coffee 的文件中。
a = 2 # Bit presentation 10 b = 3 # Bit presentation 11 console.log "The result of (a & b) is " result = a & b console.log result console.log "The result of (a | b) is " result = a | b console.log result console.log "The result of (a ^ b) is " result = a ^ b console.log result console.log "The result of (~b) is " result = ~b console.log result console.log "The result of (a << b) is " result = a << b console.log result console.log "The result of (a >> b) is " result = a >> b console.log result
打开命令提示符并编译 .coffee 文件,如下所示。
c:/> coffee -c bitwise_example.coffee
编译后,它会生成以下 JavaScript 代码。
// Generated by CoffeeScript 1.10.0 (function() { var a, b, result; a = 2; b = 3; console.log("The result of (a & b) is "); result = a & b; console.log(result); console.log("The result of (a | b) is "); result = a | b; console.log(result); console.log("The result of (a ^ b) is "); result = a ^ b; console.log(result); console.log("The result of (~b) is "); result = ~b; console.log(result); console.log("The result of (a << b) is "); result = a << b; console.log(result); console.log("The result of (a >> b) is "); result = a >> b; console.log(result); }).call(this);
现在,再次打开命令提示符并运行 CoffeeScript 文件,如下所示。
c:/> coffee bitwise_example.coffee
执行后,CoffeeScript 文件会产生以下输出。
The result of (a & b) is 2 The result of (a | b) is 3 The result of (a ^ b) is 1 The result of (~b) is -4 The result of (a << b) is 16 The result of (a >> b) is 0
coffeescript_operators_and_aliases.htm
广告