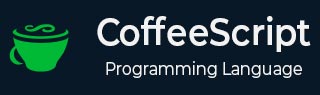
- CoffeeScript 教程
- CoffeeScript - 首页
- CoffeeScript - 概述
- CoffeeScript - 环境
- CoffeeScript - 命令行工具
- CoffeeScript - 语法
- CoffeeScript - 数据类型
- CoffeeScript - 变量
- CoffeeScript - 运算符和别名
- CoffeeScript - 条件语句
- CoffeeScript - 循环
- CoffeeScript - 列表推导式
- CoffeeScript - 函数
- CoffeeScript 面向对象编程
- CoffeeScript - 字符串
- CoffeeScript - 数组
- CoffeeScript - 对象
- CoffeeScript - 范围
- CoffeeScript - 展开运算符
- CoffeeScript - 日期
- CoffeeScript - 数学
- CoffeeScript - 异常处理
- CoffeeScript - 正则表达式
- CoffeeScript - 类和继承
- CoffeeScript 高级
- CoffeeScript - Ajax
- CoffeeScript - jQuery
- CoffeeScript - MongoDB
- CoffeeScript - SQLite
- CoffeeScript 有用资源
- CoffeeScript - 快速指南
- CoffeeScript - 有用资源
- CoffeeScript - 讨论
CoffeeScript - 比较运算符
JavaScript 支持以下比较运算符。假设变量A值为10,变量B值为20,则:
序号 | 运算符和描述 | 示例 |
---|---|---|
1 | == (等于) 检查两个操作数的值是否相等,如果相等,则条件为真。 |
(A == B) 为假。 |
2 | != (不等于) 检查两个操作数的值是否相等,如果不相等,则条件为真。 |
(A != B) 为真。 |
3 | > (大于) 检查左侧操作数的值是否大于右侧操作数的值,如果大于,则条件为真。 |
(A > B) 为假。 |
4 | < (小于) 检查左侧操作数的值是否小于右侧操作数的值,如果小于,则条件为真。 |
(A < B) 为真。 |
5 | >= (大于或等于) 检查左侧操作数的值是否大于或等于右侧操作数的值,如果大于或等于,则条件为真。 |
(A >= B) 为假。 |
6 | <= (小于或等于) 检查左侧操作数的值是否小于或等于右侧操作数的值,如果小于或等于,则条件为真。 |
(A <= B) 为真。 |
示例
以下代码演示如何在 CoffeeScript 中使用比较运算符。将此代码保存到名为comparison_example.coffee的文件中。
a = 10 b = 20 console.log "The result of (a == b) is " result = a == b console.log result console.log "The result of (a < b) is " result = a < b console.log result console.log "The result of (a > b) is " result = a > b console.log result console.log "The result of (a != b) is " result = a != b console.log result console.log "The result of (a >= b) is " result = a <= b console.log result console.log "The result of (a <= b) is " result = a >= b console.log result
打开命令提示符并编译 comparison_example.coffee 文件,如下所示。
c:/> coffee -c comparison_example.coffee
编译后,将生成以下 JavaScript 代码。
// Generated by CoffeeScript 1.10.0 (function() { var a, b, result; a = 10; b = 20; console.log("The result of (a == b) is "); result = a === b; console.log(result); console.log("The result of (a < b) is "); result = a < b; console.log(result); console.log("The result of (a > b) is "); result = a > b; console.log(result); console.log("The result of (a != b) is "); result = a !== b; console.log(result); console.log("The result of (a >= b) is "); result = a <= b; console.log(result); console.log("The result of (a <= b) is "); result = a >= b; console.log(result); }).call(this);
现在,再次打开命令提示符并运行 CoffeeScript 文件,如下所示。
c:/> coffee comparison_example.coffee
执行后,CoffeeScript 文件将产生以下输出。
The result of (a == b) is false The result of (a < b) is true The result of (a > b) is false The result of (a != b) is true The result of (a >= b) is true The result of (a <= b) is false
coffeescript_operators_and_aliases.htm
广告