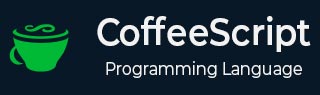
- CoffeeScript 教程
- CoffeeScript - 首页
- CoffeeScript - 概述
- CoffeeScript - 环境
- CoffeeScript - 命令行工具
- CoffeeScript - 语法
- CoffeeScript - 数据类型
- CoffeeScript - 变量
- CoffeeScript - 运算符和别名
- CoffeeScript - 条件语句
- CoffeeScript - 循环
- CoffeeScript - 列表推导
- CoffeeScript - 函数
- CoffeeScript 面向对象
- CoffeeScript - 字符串
- CoffeeScript - 数组
- CoffeeScript - 对象
- CoffeeScript - 范围
- CoffeeScript - 展开运算符
- CoffeeScript - 日期
- CoffeeScript - 数学
- CoffeeScript - 异常处理
- CoffeeScript - 正则表达式
- CoffeeScript - 类和继承
- CoffeeScript 高级
- CoffeeScript - Ajax
- CoffeeScript - jQuery
- CoffeeScript - MongoDB
- CoffeeScript - SQLite
- CoffeeScript 有用资源
- CoffeeScript - 快速指南
- CoffeeScript - 有用资源
- CoffeeScript - 讨论
CoffeeScript - switch 语句
switch 语句允许测试一个变量是否等于一个值列表中的某个值。每个值称为一个case,被切换的变量会针对每个 switch case 进行检查。以下是 JavaScript 中switch 的语法。
switch (expression){ case condition 1: statement(s) break; case condition 2: statement(s) break; case condition n: statement(s) break; default: statement(s) }
在 JavaScript 中,每个 switch case 之后,我们必须使用break 语句。如果我们不小心忘记了break 语句,则有可能从一个 switch case 掉到另一个 switch case。
CoffeeScript 中的 Switch 语句
CoffeeScript 通过使用switch-when-else子句的组合来解决这个问题。这里有一个可选的 switch 表达式,后跟 case 语句。
每个 case 语句都有两个子句when 和then。when 后面跟着条件,then 后面跟着一组语句,如果满足特定条件,则执行这些语句。最后,我们有一个可选的else 子句,其中包含默认条件的操作。
语法
以下是 CoffeeScript 中switch 语句的语法。我们指定没有括号的表达式,并通过保持适当的缩进来分隔 case 语句。
switch expression when condition1 then statements when condition2 then statements when condition3 then statements else statements
流程图
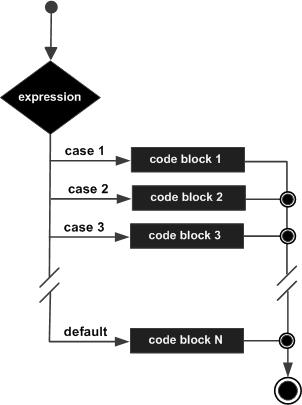
示例
以下示例演示了在 CoffeeScript 中使用switch 语句的方法。将此代码保存在名为switch_example.coffee 的文件中。
name="Ramu" score=75 message = switch when score>=75 then "Congrats your grade is A" when score>=60 then "Your grade is B" when score>=50 then "Your grade is C" when score>=35 then "Your grade is D" else "Your grade is F and you are failed in the exam" console.log message
打开命令提示符并编译 .coffee 文件,如下所示。
c:\> coffee -c switch_exmple.coffee
编译后,它会生成以下 JavaScript 代码。
// Generated by CoffeeScript 1.10.0 (function() { var message, name, score; name = "Ramu"; score = 75; message = (function() { switch (false) { case !(score >= 75): return "Congrats your grade is A"; case !(score >= 60): return "Your grade is B"; case !(score >= 50): return "Your grade is C"; case !(score >= 35): return "Your grade is D"; default: return "Your grade is F and you are failed in the exam"; } })(); console.log(message); }).call(this);
现在,再次打开命令提示符并运行 CoffeeScript 文件,如下所示:
c:\> coffee switch_exmple.coffee
执行后,CoffeeScript 文件会产生以下输出。
Congrats your grade is A
when 子句的多个值
我们还可以通过在 switch case 中使用逗号 (,) 分隔它们来为单个 when 子句指定多个值。
示例
以下示例显示了如何通过为when 子句指定多个值来编写 CoffeeScript switch 语句。将此代码保存在名为switch_multiple_example.coffee 的文件中。
name="Ramu" score=75 message = switch name when "Ramu","Mohammed" then "You have passed the examination with grade A" when "John","Julia" then "You have passed the examination with grade is B" when "Rajan" then "Sorry you failed in the examination" else "No result" console.log message
打开命令提示符并编译 .coffee 文件,如下所示。
c:\> coffee -c switch_multiple_example.coffee
编译后,它会生成以下 JavaScript 代码。
// Generated by CoffeeScript 1.10.0 (function() { var message, name, score; name = "Ramu"; score = 75; message = (function() { switch (name) { case "Ramu": case "Mohammed": return "You have passed the examination with grade A"; case "John": case "Julia": return "You have passed the examination with grade is B"; case "Rajan": return "Sorry you failed in the examination"; default: return "No result"; } })(); console.log(message); }).call(this);
现在,再次打开命令提示符并运行 CoffeeScript 文件,如下所示:
c:\> coffee switch_multiple_example.coffee
执行后,CoffeeScript 文件会产生以下输出。
You have passed the examination with grade A