使用Python和Weatherstack API创建天气预报GUI
为什么需要天气预报?
天气预报是人类生活中必不可少的一部分。近年来,技术的进步使得高精度的天气预报更容易实现。OpenWeatherMap是一个流行的API,允许开发者检索天气数据。在本教程中,我们将使用Python和OpenWeatherMap API创建一个天气预报图形用户界面(GUI)。本教程将涵盖构建GUI和从OpenWeatherMap API检索数据的必要步骤。
前提条件
在我们开始任务之前,需要在您的系统上安装以下内容:
推荐设置列表:
pip install tkinter
用户应该能够访问任何独立的IDE,例如VS-Code、PyCharm、Atom或Sublime Text。
也可以使用在线Python编译器,例如Kaggle.com、Google Cloud Platform或其他任何编译器。
更新版本的Python。在撰写本文时,我使用了3.10.9版本。
了解如何使用Jupyter Notebook。
了解和应用虚拟环境将是有益的,但不是必需的。
还期望用户对统计学和数学有良好的理解。
完成任务所需的步骤
步骤1:导入必要的模块
import tkinter as tk import requests
tkinter模块用于创建GUI窗口、标签和输入字段。requests模块用于向Weatherstack API发出API请求。
步骤2:设置您的Weatherstack API密钥
API_KEY = "your_weatherstack_api_key"
将your_weatherstack_api_key替换为您实际的API密钥。
步骤3:定义Weatherstack API的URL
API_URL = "http://api.weatherstack.com/current"
这是将用于向Weatherstack API发出请求的URL。
步骤4:定义GUI窗口
root = tk.Tk() root.title("Weather Forecast")
这将创建GUI的主窗口,并将标题设置为“天气预报”。
步骤5:定义城市和国家/地区的标签和输入字段
city_label = tk.Label(root, text="City:") city_label.grid(row=0, column=0, padx=5, pady=5) city_entry = tk.Entry(root) city_entry.grid(row=0, column=1, padx=5, pady=5) country_label = tk.Label(root, text="Country:") country_label.grid(row=1, column=0, padx=5, pady=5) country_entry = tk.Entry(root) country_entry.grid(row=1, column=1, padx=5, pady=5)
这些将创建标签和输入字段,供用户输入他们想要获取天气数据的城市和国家/地区。
步骤6:定义从API获取天气数据的函数
def get_weather(): city = city_entry.get() country = country_entry.get() # Build the URL with the API key, city, and country url = f"{API_URL}?access_key={API_KEY}&query={city},{country}" # Make the API request and get the JSON response response = requests.get(url).json() # Extract the relevant weather data from the JSON response temperature = response["current"]["temperature"] weather_desc = response["current"]["weather_descriptions"][0] # Display the weather data in the GUI temperature_label.config(text=f"Temperature: {temperature}°C") weather_desc_label.config(text=f"Weather Description: {weather_desc}")
此函数从输入字段获取城市和国家/地区,使用API密钥、城市和国家/地区构建API请求URL,发出API请求,并从JSON响应中提取相关的天气数据。然后,它将温度和天气描述显示在GUI中的相应标签中。
步骤7:定义获取天气数据的按钮
get_weather_button = tk.Button(root, text="Get Weather", command=get_weather) get_weather_button.grid(row=2, column=0, columnspan=2, padx=5, pady=5)
这将创建一个按钮,单击时调用get_weather函数。
步骤8:定义显示天气数据的标签
temperature_label = tk.Label(root, text="") temperature_label.grid(row=3, column=0, columnspan=2, padx=5, pady=5) weather_desc_label = tk.Label(root, text="") weather_desc_label.grid(row=4, column=0, columnspan=2, padx=5, pady=5)
这些将创建显示温度和天气描述的标签。
步骤9:运行GUI窗口
root.mainloop()
这将启动GUI,并在用户需要时保持运行。
最终代码,程序
import tkinter as tk import requests # Set your weatherstack API key here API_KEY = "your_weatherstack_api_key" # Define the URL for the weatherstack API API_URL = "http://api.weatherstack.com/current" # Define the GUI window root = tk.Tk() root.title("Weather Forecast") # Define the labels and entry fields for the city and country city_label = tk.Label(root, text="City:") city_label.grid(row=0, column=0, padx=5, pady=5) city_entry = tk.Entry(root) city_entry.grid(row=0, column=1, padx=5, pady=5) country_label = tk.Label(root, text="Country:") country_label.grid(row=1, column=0, padx=5, pady=5) country_entry = tk.Entry(root) country_entry.grid(row=1, column=1, padx=5, pady=5) # Define the function to get the weather data from the API def get_weather(): city = city_entry.get() country = country_entry.get() # Build the URL with the API key, city, and country url = f"{API_URL}?access_key={API_KEY}&query={city},{country}" # Make the API request and get the JSON response response = requests.get(url).json() # Extract the relevant weather data from the JSON response temperature = response["current"]["temperature"] weather_desc = response["current"]["weather_descriptions"][0] # Display the weather data in the GUI temperature_label.config(text=f"Temperature: {temperature}°C") weather_desc_label.config(text=f"Weather Description: {weather_desc}") # Define the button to get the weather data get_weather_button = tk.Button(root, text="Get Weather", command=get_weather) get_weather_button.grid(row=2, column=0, columnspan=2, padx=5, pady=5) # Define the labels to display the weather data temperature_label = tk.Label(root, text="") temperature_label.grid(row=3, column=0, columnspan=2, padx=5, pady=5) weather_desc_label = tk.Label(root, text="") weather_desc_label.grid(row=4, column=0, columnspan=2, padx=5, pady=5) # Run the GUI window root.mainloop()
在这个例子中,我们首先导入必要的库:tkinter和requests。然后,我们设置Weatherstack API密钥并定义API URL。我们使用tk.Tk()函数创建一个GUI窗口,并设置窗口标题。
我们使用tk.Label()和tk.Entry()函数为城市和国家/地区创建两个标签和两个输入字段。然后,我们定义一个名为get_weather()的函数,该函数从API获取天气数据并在GUI中显示它。该函数使用requests.get()函数向API URL发出GET请求,其中包含来自输入字段的城市和国家/地区。然后,它从JSON响应中提取温度和天气描述数据,并更新GUI中的温度和天气描述标签。
我们使用tk.Button()函数创建一个获取天气数据的按钮,并将command参数设置为get_weather()函数。我们还创建了两个标签来显示温度和天气描述数据。
最后,我们使用root.mainloop()函数运行GUI窗口。当用户输入城市和国家/地区并单击“获取天气”按钮时,get_weather()函数将向
输出
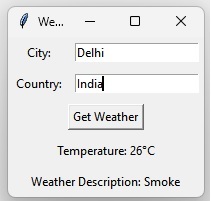
此图片显示了输入城市和国家/地区名称后天气应用程序GUI的输出。API密钥将根据用户而变化。
结论
在本教程中,我们学习了如何使用Python和Weatherstack API创建天气预报GUI。我们已经介绍了构建GUI和从Weatherstack API检索数据的必要步骤。我们还解释了每个小节,并提供了使用Weatherstack API的实际天气预报应用程序示例。