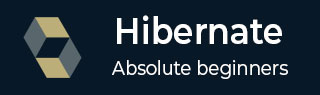
- Hibernate 教程
- Hibernate - 首页
- ORM - 概述
- Hibernate - 概述
- Hibernate - 架构
- Hibernate - 环境
- Hibernate - 配置
- Hibernate - 会话(Sessions)
- Hibernate - 持久化类
- Hibernate - 映射文件
- Hibernate - 映射类型
- Hibernate - 示例
- Hibernate - 对象关系映射(O/R Mappings)
- Hibernate - 级联类型
- Hibernate - 注解
- Hibernate - 查询语言
- Hibernate - Criteria 查询
- Hibernate - 原生 SQL
- Hibernate - 缓存
- Hibernate - 实体生命周期
- Hibernate - 批处理
- Hibernate - 拦截器
- Hibernate - ID 生成器
- Hibernate - 保存图片
- Hibernate - log4j 集成
- Hibernate - Spring 集成
- Hibernate - Struts 2 集成
- Hibernate - Web 应用
- 映射表示例
- Hibernate - 基于表继承(Table Per Hiearchy)
- Hibernate - 基于具体类表(Table Per Concrete Class)
- Hibernate - 基于子类表(Table Per Subclass)
- Hibernate 有用资源
- Hibernate - 问答
- Hibernate - 快速指南
- Hibernate - 有用资源
- Hibernate - 讨论
Hibernate - 排序映射(SortedMap 映射)
SortedMap 是类似于 Map 的 Java 集合,它以键值对的形式存储元素,并对其键提供全序关系。映射中不允许重复元素。映射根据其键的自然顺序排序,或者根据在排序映射创建时通常提供的 Comparator 排序。
SortedMap 在映射表中使用 <map> 元素进行映射,并且可以使用 java.util.TreeMap 初始化有序映射。
定义 RDBMS 表
考虑这样一种情况:我们需要将员工记录存储在 EMPLOYEE 表中,该表具有以下结构:
create table EMPLOYEE ( id INT NOT NULL auto_increment, first_name VARCHAR(20) default NULL, last_name VARCHAR(20) default NULL, salary INT default NULL, PRIMARY KEY (id) );
此外,假设每个员工可以拥有一个或多个与其相关的证书。我们将证书相关信息存储在具有以下结构的单独表中:
create table CERTIFICATE ( id INT NOT NULL auto_increment, certificate_type VARCHAR(40) default NULL, certificate_name VARCHAR(30) default NULL, employee_id INT default NULL, PRIMARY KEY (id) );
EMPLOYEE 和 CERTIFICATE 对象之间将存在一对多关系。
定义 POJO 类
让我们实现一个 POJO 类 Employee,它将用于持久化与 EMPLOYEE 表相关的对象,并在 List 变量中包含证书集合。
import java.util.*; public class Employee { private int id; private String firstName; private String lastName; private int salary; private SortedMap certificates; public Employee() {} public Employee(String fname, String lname, int salary) { this.firstName = fname; this.lastName = lname; this.salary = salary; } public int getId() { return id; } public void setId( int id ) { this.id = id; } public String getFirstName() { return firstName; } public void setFirstName( String first_name ) { this.firstName = first_name; } public String getLastName() { return lastName; } public void setLastName( String last_name ) { this.lastName = last_name; } public int getSalary() { return salary; } public void setSalary( int salary ) { this.salary = salary; } public SortedMap getCertificates() { return certificates; } public void setCertificates( SortedMap certificates ) { this.certificates = certificates; } }
我们需要定义另一个与 CERTIFICATE 表对应的 POJO 类,以便可以将证书对象存储到 CERTIFICATE 表中并从中检索。此类还应实现 Comparable 接口和 compareTo 方法,该方法将用于在映射文件中设置 sort="natural" 时对 SortedMap 的键元素进行排序(参见下面的映射文件)。
public class Certificate implements Comparable <String>{ private int id; private String name; public Certificate() {} public Certificate(String name) { this.name = name; } public int getId() { return id; } public void setId( int id ) { this.id = id; } public String getName() { return name; } public void setName( String name ) { this.name = name; } public int compareTo(String that){ final int BEFORE = -1; final int AFTER = 1; if (that == null) { return BEFORE; } Comparable thisCertificate = this; Comparable thatCertificate = that; if(thisCertificate == null) { return AFTER; } else if(thatCertificate == null) { return BEFORE; } else { return thisCertificate.compareTo(thatCertificate); } } }
定义 Hibernate 映射文件
让我们开发我们的映射文件,该文件指示 Hibernate 如何将定义的类映射到数据库表。<map> 元素将用于定义所用 Map 的规则。
<?xml version = "1.0" encoding = "utf-8"?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD//EN" "http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd"> <hibernate-mapping> <class name = "Employee" table = "EMPLOYEE"> <meta attribute = "class-description"> This class contains the employee detail. </meta> <id name = "id" type = "int" column = "id"> <generator class="native"/> </id> <map name = "certificates" cascade="all" sort="MyClass"> <key column = "employee_id"/> <index column = "certificate_type" type = "string"/> <one-to-many class="Certificate"/> </map> <property name = "firstName" column = "first_name" type = "string"/> <property name = "lastName" column = "last_name" type = "string"/> <property name = "salary" column = "salary" type = "int"/> </class> <class name = "Certificate" table = "CERTIFICATE"> <meta attribute = "class-description"> This class contains the certificate records. </meta> <id name = "id" type = "int" column = "id"> <generator class="native"/> </id> <property name = "name" column = "certificate_name" type = "string"/> </class> </hibernate-mapping>
您应该将映射文档保存在格式为 <classname>.hbm.xml 的文件中。我们将映射文档保存在 Employee.hbm.xml 文件中。您已经熟悉大多数映射细节,但让我们再次查看映射文件的所有元素:
映射文档是一个 XML 文档,其根元素为 <hibernate-mapping>,其中包含两个与每个类对应的 <class> 元素。
<class> 元素用于定义从 Java 类到数据库表的特定映射。Java 类名使用 class 元素的 name 属性指定,数据库表名使用 table 属性指定。
<meta> 元素是可选元素,可用于创建类描述。
<id> 元素将类中唯一的 ID 属性映射到数据库表的主键。id 元素的 name 属性引用类中的属性,column 属性引用数据库表中的列。type 属性包含 Hibernate 映射类型,此映射类型将 Java 类型转换为 SQL 数据类型。
id 元素中的 <generator> 元素用于自动生成主键值。generator 元素的 class 属性设置为 native,以让 Hibernate 根据底层数据库的功能选择 identity、sequence 或 hilo 算法来创建主键。
<property> 元素用于将 Java 类属性映射到数据库表中的列。元素的 name 属性引用类中的属性,column 属性引用数据库表中的列。type 属性包含 Hibernate 映射类型,此映射类型将 Java 类型转换为 SQL 数据类型。
<map> 元素用于设置 Certificate 和 Employee 类之间的关系。我们在 <map> 元素中使用了 cascade 属性来告诉 Hibernate 在同时持久化 Employee 对象时持久化 Certificate 对象。name 属性设置为父类中定义的 SortedMap 变量,在本例中为 certificates。sort 属性可以设置为 natural 以进行自然排序,也可以设置为实现 java.util.Comparator 的自定义类。我们使用了实现 java.util.Comparator 的类 MyClass 来反转在 Certificate 类中实现的排序顺序。
<index> 元素用于表示键值映射对的关键部分。键将使用字符串类型存储在 certificate_type 列中。
<key> 元素是 CERTIFICATE 表中保存到父对象(即 EMPLOYEE 表)的外键的列。
<one-to-many> 元素指示一个 Employee 对象与多个 Certificate 对象相关联,因此 Certificate 对象必须具有与其关联的 Employee 父对象。您可以根据需要使用 <one-to-one>、<many-to-one> 或 <many-to-many> 元素。
如果我们使用 sort="natural" 设置,则不需要创建单独的类,因为 Certificate 类已经实现了 Comparable 接口,Hibernate 将使用 Certificate 类中定义的 compareTo() 方法来比较 SortedMap 键。但是,我们在映射文件中使用自定义比较器类 MyClass,因此我们必须根据我们的排序算法创建此类。让我们对映射中可用的键进行降序排序。
import java.util.Comparator; public class MyClass implements Comparator <String>{ public int compare(String o1, String o2) { final int BEFORE = -1; final int AFTER = 1; /* To reverse the sorting order, multiple by -1 */ if (o2 == null) { return BEFORE * -1; } Comparable thisCertificate = o1; Comparable thatCertificate = o2; if(thisCertificate == null) { return AFTER * 1; } else if(thatCertificate == null) { return BEFORE * -1; } else { return thisCertificate.compareTo(thatCertificate) * -1; } } }
最后,我们将创建包含 main() 方法的应用程序类来运行应用程序。我们将使用此应用程序来保存一些员工记录及其证书,然后我们将对这些记录应用 CRUD 操作。
import java.util.*; import org.hibernate.HibernateException; import org.hibernate.Session; import org.hibernate.Transaction; import org.hibernate.SessionFactory; import org.hibernate.cfg.Configuration; public class ManageEmployee { private static SessionFactory factory; public static void main(String[] args) { try{ factory = new Configuration().configure().buildSessionFactory(); }catch (Throwable ex) { System.err.println("Failed to create sessionFactory object." + ex); throw new ExceptionInInitializerError(ex); } ManageEmployee ME = new ManageEmployee(); /* Let us have a set of certificates for the first employee */ TreeMap set1 = new TreeMap(); set1.put("ComputerScience", new Certificate("MCA")); set1.put("BusinessManagement", new Certificate("MBA")); set1.put("ProjectManagement", new Certificate("PMP")); /* Add employee records in the database */ Integer empID1 = ME.addEmployee("Manoj", "Kumar", 4000, set1); /* Another set of certificates for the second employee */ TreeMap set2 = new TreeMap(); set2.put("ComputerScience", new Certificate("MCA")); set2.put("BusinessManagement", new Certificate("MBA")); /* Add another employee record in the database */ Integer empID2 = ME.addEmployee("Dilip", "Kumar", 3000, set2); /* List down all the employees */ ME.listEmployees(); /* Update employee's salary records */ ME.updateEmployee(empID1, 5000); /* Delete an employee from the database */ ME.deleteEmployee(empID2); /* List down all the employees */ ME.listEmployees(); } /* Method to add an employee record in the database */ public Integer addEmployee(String fname, String lname, int salary, TreeMap cert){ Session session = factory.openSession(); Transaction tx = null; Integer employeeID = null; try{ tx = session.beginTransaction(); Employee employee = new Employee(fname, lname, salary); employee.setCertificates(cert); employeeID = (Integer) session.save(employee); tx.commit(); } catch (HibernateException e) { if (tx!=null) tx.rollback(); e.printStackTrace(); } finally { session.close(); } return employeeID; } /* Method to list all the employees detail */ public void listEmployees( ){ Session session = factory.openSession(); Transaction tx = null; try { tx = session.beginTransaction(); List employees = session.createQuery("FROM Employee").list(); for (Iterator iterator1 = employees.iterator(); iterator1.hasNext();){ Employee employee = (Employee) iterator1.next(); System.out.print("First Name: " + employee.getFirstName()); System.out.print(" Last Name: " + employee.getLastName()); System.out.println(" Salary: " + employee.getSalary()); SortedMap<String, Certificate> map = employee.getCertificates(); for(Map.Entry<String,Certificate> entry : map.entrySet()){ System.out.print("\tCertificate Type: " + entry.getKey()); System.out.println(", Name: " + (entry.getValue()).getName()); } } tx.commit(); } catch (HibernateException e) { if (tx!=null) tx.rollback(); e.printStackTrace(); } finally { session.close(); } } /* Method to update salary for an employee */ public void updateEmployee(Integer EmployeeID, int salary ){ Session session = factory.openSession(); Transaction tx = null; try { tx = session.beginTransaction(); Employee employee = (Employee)session.get(Employee.class, EmployeeID); employee.setSalary( salary ); session.update(employee); tx.commit(); } catch (HibernateException e) { if (tx!=null) tx.rollback(); e.printStackTrace(); } finally { session.close(); } } /* Method to delete an employee from the records */ public void deleteEmployee(Integer EmployeeID){ Session session = factory.openSession(); Transaction tx = null; try { tx = session.beginTransaction(); Employee employee = (Employee)session.get(Employee.class, EmployeeID); session.delete(employee); tx.commit(); } catch (HibernateException e) { if (tx!=null) tx.rollback(); e.printStackTrace(); } finally { session.close(); } } }
编译和执行
以下是编译和运行上述应用程序的步骤。在继续编译和执行之前,请确保已正确设置 PATH 和 CLASSPATH。
创建 hibernate.cfg.xml 配置文件,如配置章节中所述。
创建如上所示的 Employee.hbm.xml 映射文件。
创建如上所示的 Employee.java 源文件并编译它。
创建如上所示的 Certificate.java 源文件并编译它。
创建如上所示的 MyClass.java 源文件并编译它。
创建如上所示的 ManageEmployee.java 源文件并编译它。
执行 ManageEmployee 二进制文件以运行程序。
您将在屏幕上看到以下结果,同时将在 EMPLOYEE 和 CERTIFICATE 表中创建记录。您可以看到证书类型已按相反顺序排序。您可以尝试更改映射文件,只需设置 sort="natural" 并执行程序并比较结果。
$java ManageEmployee .......VARIOUS LOG MESSAGES WILL DISPLAY HERE........ First Name: Manoj Last Name: Kumar Salary: 4000 Certificate Type: ProjectManagement, Name: PMP Certificate Type: ComputerScience, Name: MCA Certificate Type: BusinessManagement, Name: MBA First Name: Dilip Last Name: Kumar Salary: 3000 Certificate Type: ComputerScience, Name: MCA Certificate Type: BusinessManagement, Name: MBA First Name: Manoj Last Name: Kumar Salary: 5000 Certificate Type: ProjectManagement, Name: PMP Certificate Type: ComputerScience, Name: MCA Certificate Type: BusinessManagement, Name: MBA
如果您检查 EMPLOYEE 和 CERTIFICATE 表,它们应该具有以下记录:
mysql> select * from EMPLOYEE; +----+------------+-----------+--------+ | id | first_name | last_name | salary | +----+------------+-----------+--------+ | 74 | Manoj | Kumar | 5000 | +----+------------+-----------+--------+ 1 row in set (0.00 sec) mysql> select * from CERTIFICATE; +----+--------------------+------------------+-------------+ | id | certificate_type | certificate_name | employee_id | +----+--------------------+------------------+-------------+ | 52 | BusinessManagement | MBA | 74 | | 53 | ComputerScience | MCA | 74 | | 54 | ProjectManagement | PMP | 74 | +----+--------------------+------------------+-------------+ 3 rows in set (0.00 sec) mysql>