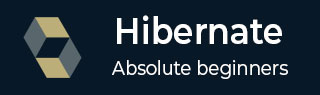
- Hibernate 教程
- Hibernate - 首页
- ORM - 概述
- Hibernate - 概述
- Hibernate - 架构
- Hibernate - 环境
- Hibernate - 配置
- Hibernate - 会话
- Hibernate - 持久化类
- Hibernate - 映射文件
- Hibernate - 映射类型
- Hibernate - 示例
- Hibernate - 对象关系映射
- Hibernate - 级联类型
- Hibernate - 注解
- Hibernate - 查询语言
- Hibernate - Criteria 查询
- Hibernate - 原生 SQL
- Hibernate - 缓存
- Hibernate - 实体生命周期
- Hibernate - 批处理
- Hibernate - 拦截器
- Hibernate - ID 生成器
- Hibernate - 保存图片
- Hibernate - log4j 集成
- Hibernate - Spring 集成
- Hibernate - Struts 2 集成
- Hibernate - Web 应用
- 映射表示例
- Hibernate - 基于层次结构的表
- Hibernate - 基于具体类的表
- Hibernate - 基于子类的表
- Hibernate 有用资源
- Hibernate - 常见问题解答
- Hibernate - 快速指南
- Hibernate - 有用资源
- Hibernate - 讨论
Hibernate - Spring 集成
Spring 是一个用于构建企业级 Java 应用程序的开源框架。Spring 有一个 **applicationContext.xml** 文件。我们将使用此文件提供所有信息,包括数据库配置。由于数据库配置包含在 Hibernate 的 **hibernate.cfg.xml** 中,因此我们不再需要它。
在 Hibernate 中,我们使用 StandardServiceRegistry、MetadataSources、SessionFactory 获取 Session 和 Transaction。Spring 框架提供了一个 **HibernateTemplate** 类,您只需调用 save 方法即可将数据持久化到数据库。**HibernateTemplate** 类位于 **org.springframework.orm.hibernate3** 包中。
语法
// create a new student object Student s1 = new Student( 111, "Karan", "Physics"); // save the student object in database using HibernateTemplate instance hibernateTemplate.persist(s1);
所需库
要使用 HibernateTemplate,您需要从 https://mvnrepository.com/artifact/org.springframework/spring-hibernate3/2.0.8 获取 jar 文件
您需要从 https://mvnrepository.com/artifact/org.springframework/spring-dao/2.0.3 获取 spring-dao jar 文件
您还需要将 Hibernate-core jar 文件放入您的 CLASSPATH 中。
HibernateTemplate 类的有用方法
以下是 HibernateTemplate 类重要方法的列表
序号 | 方法和描述 |
---|---|
1 | void persist(Object entity) 将对象保存到数据库中的映射表中。 |
2 | Serializable save(Object entity) 将对象保存到数据库中的映射表记录中并返回 ID。 |
3 | void saveOrUpdate(Object entity) 保存或更新与对象映射的表。如果输入了 ID,则更新记录;否则保存。 |
4 | void update(Object entity) 更新与对象映射的表。 |
5 | void delete(Object entity) 根据 ID 删除数据库中与表映射的给定对象。 |
示例
让我们详细讨论 Spring Hibernate 集成以及一个示例。
创建映射类
让我们创建 POJO 类,其数据将持久化到数据库中。
Student.java
package com.tutorialspoint; public class Student { private long id; private String name; private String dept; public long getId() { return id; } public void setId(long id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getDept() { return dept; } public void setDept(String dept) { this.dept = dept; } }
创建 DAO 类
创建 DAO 类,它将使用 HibernateTemplate 类来创建、更新和删除学生对象。
StudentDAO.java
package com.tutorialspoint; import org.springframework.orm.hibernate3.HibernateTemplate; public class StudentDAO{ HibernateTemplate template; public void setTemplate(HibernateTemplate template) { this.template = template; } public void saveStudent(Student s){ template.save(s); } public void updateStudent(Student s){ template.update(s); } public void deleteStudent(Student s){ template.delete(s); } }
创建映射 XML
为学生对象创建 Hibernate 映射以持久化到数据库中。
student.hbm.xml
<?xml version='1.0' encoding='UTF-8'?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "https://hibernate.com.cn/dtd/hibernate-mapping-3.0.dtd"> <hibernate-mapping> <class name="Student" table="student_hib"> <id name="id"> <generator class="assigned"></generator> </id> <property name="name"></property> <property name="dept"></property> </class> </hibernate-mapping>
创建 Application Context XML
创建 Spring Application Context
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd"> <bean id="dataSource" class=" com.mysql.cj.jdbc.MysqlDataSource"> <property name="driverClassName" value=" com.mysql.cj.jdbc.Driver"></property> <property name="url" value=" jdbc:mysql://127.0.0.1/TUTORIALSPOINT"></property> <property name="username" value="root"></property> <property name="password" value="guest123"></property> </bean> <bean id="localSessionFactory" class="org.springframework.orm.hibernate3.LocalSessionFactoryBean"> <property name="dataSource" ref="dataSource"></property> <property name="mappingResources"> <list> <value>student.hbm.xml</value> </list> </property> <props> <prop key="hibernate.dialect"> org.hibernate.dialect.MySQL8Dialect</prop> <prop key="hibernate.hbm2ddl.auto">update</prop> </props> </bean> <bean id="hibernateTemplate" class="org.springframework.orm.hibernate3.HibernateTemplate"> <property name="sessionFactory" ref="localSessionFactory"></property> </bean> <bean id="st" class="StudentDAO"> <property name="template" ref="hibernateTemplate"></property> </bean> </beans>
创建应用程序类
最后,我们将创建包含 main() 方法的应用程序类来运行应用程序。我们将使用此应用程序来测试 Spring Hibernate 集成。
PersistStudent.java
package com.tutorialspoint; import org.springframework.beans.factory.BeanFactory; import org.springframework.beans.factory.xml.XmlBeanFactory; import org.springframework.core.io.ClassPathResource; import org.springframework.core.io.Resource; public class PersistStudent { public static void main(String[] args) { Resource r=new ClassPathResource("applicationContext.xml"); BeanFactory factory=new XmlBeanFactory(r); StudentDAO dao=(StudentDAO)factory.getBean("st"); Student s=new Student(); s.setId(190); s.setName("Danny Jing"); s.setDept("Physics"); dao.persistStudent(s); System.out.println(" Successfully persisted the student. Please check your database for results."); } }
编译和执行
执行 PersistStudent 二进制文件以运行程序。
输出
您将得到以下结果,并且记录将被创建到 Shape 表中。
$java PersistStudent Successfully persisted the student. Please check your database for results.
如果您检查您的表,则应该有以下记录:
mysql> select * from student; mysql> select * from student; +------+------------+---------+ | id | name | dept | +------+------------+---------+ | 190 | Danny Jing | Physics | +------+------------+---------+ 1 row in set (0.00 sec)