如何优雅地关闭Android应用程序?
简介
在许多Android应用程序中,我们可以看到,当应用程序关闭时,会添加一个动画,该动画将通过显示关闭动画来关闭应用程序。这将使应用程序用户界面更好。在本文中,我们将了解如何优雅地关闭Android应用程序?
实现
我们将创建一个简单的应用程序,在这个应用程序中,我们将创建一个文本视图,用于显示应用程序的标题。之后,我们将创建一个按钮,我们将使用它来关闭应用程序。现在让我们转向Android Studio,在Android Studio中创建一个新项目。
步骤1:在Android Studio中创建一个新项目
导航到Android Studio,如下面的屏幕所示。在下面的屏幕中,单击“新建项目”以创建一个新的Android Studio项目。
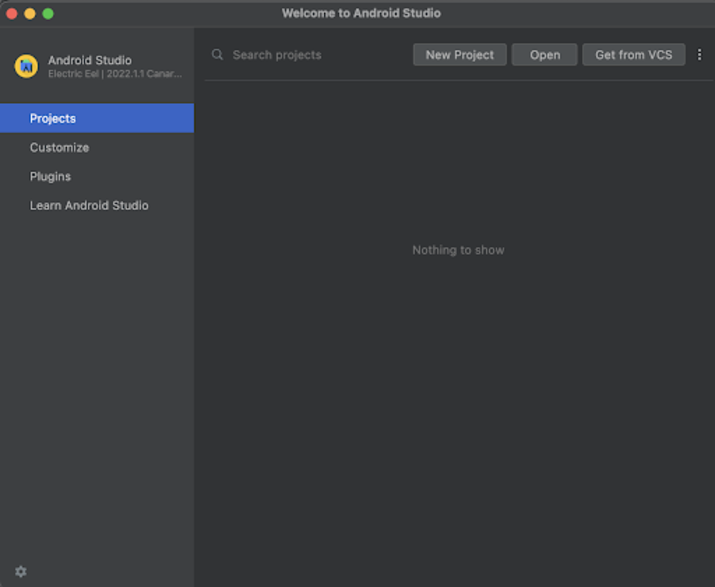
单击“新建项目”后,您将看到下面的屏幕。
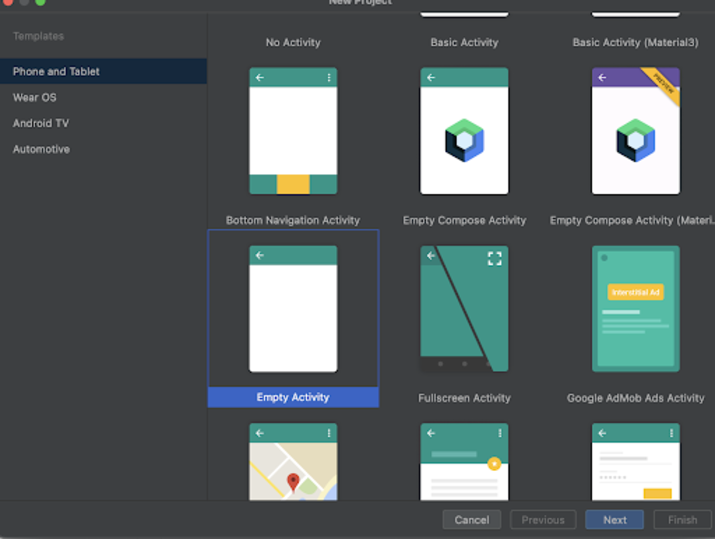
在这个屏幕中,我们只需选择“空活动”,然后单击“下一步”。单击“下一步”后,您将看到下面的屏幕。
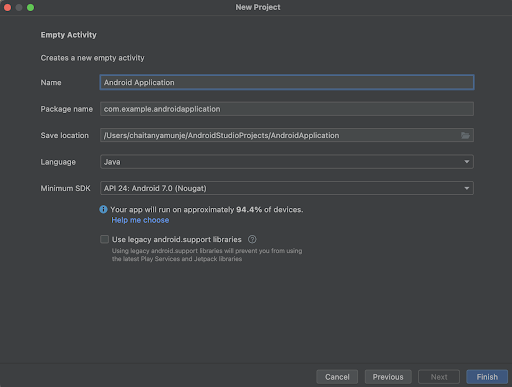
在这个屏幕中,我们只需指定项目名称。然后包名将自动生成。
注意 - 确保选择Java作为语言。
指定所有详细信息后,单击“完成”以创建一个新的Android Studio项目。
项目创建后,我们将看到打开的两个文件,即activity_main.xml和MainActivity.java文件。
步骤2:使用activity_main.xml
导航到activity_main.xml。如果此文件不可见,要打开此文件,在左侧窗格中导航到app>res>layout>activity_main.xml以打开此文件。打开此文件后,将以下代码添加到其中。代码中添加了注释,以便详细了解。
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/idRLLayout" android:layout_width="match_parent" android:layout_height="match_parent" android:layoutDirection="ltr" android:paddingTop="8dp" android:paddingBottom="8dp" tools:context=".MainActivity"> <!-- creating a text view on below line--> <TextView android:id="@+id/idTVHeading" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_centerInParent="true" android:layout_marginStart="20dp" android:layout_marginTop="20dp" android:layout_marginEnd="20dp" android:layout_marginBottom="20dp" android:padding="4dp" android:text="Close Application gracefully in Android" android:textAlignment="center" android:textColor="@color/black" android:textSize="20sp" android:textStyle="bold" /> <!-- creating a button for closing the application on below line --> <Button android:id="@+id/idBtnClose" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_below="@id/idTVHeading" android:layout_centerHorizontal="true" android:layout_margin="16dp" android:backgroundTint="@color/purple_500" android:text="Close Application" android:textAllCaps="false" /> </RelativeLayout>
说明:在上面的代码中,我们创建一个根布局作为相对布局。在这个布局中,我们创建一个文本视图,用于显示应用程序的标题。之后,我们将创建一个按钮,我们将使用它通过添加关闭动画来关闭我们的应用程序。
步骤3:创建一个新的动画文件以添加动画
导航到app>res>右键单击它>新建>资源目录,并将其命名为anim。之后,右键单击该目录并创建一个新的anim文件,并将其命名为animation.xml。创建文件后,将以下代码添加到其中。代码中添加了注释,以便详细了解。
<?xml version="1.0" encoding="utf-8"?> <!-- on below line creating an animation and adding duration to that animation --> <alpha xmlns:android="http://schemas.android.com/apk/res/android" android:duration="1000" android:fromAlpha="1.0" android:interpolator="@android:anim/accelerate_interpolator" android:toAlpha="0.0" />
说明:在上面的代码中,我们通过创建alpha来创建一个动画。在这里,我们首先以毫秒为单位指定动画的持续时间。之后,我们创建了一个fromAlpha,然后是两个alpha。然后我们向其中添加一个插值器。
步骤4:使用MainActivity.java文件
导航到MainActivity.java。如果此文件不可见,要打开此文件,在左侧窗格中导航到app>res>layout>MainActivity.java以打开此文件。打开此文件后,将以下代码添加到其中。代码中添加了注释,以便详细了解。
package com.example.java_test_application; import android.os.Bundle; import android.view.View; import android.view.animation.Animation; import android.view.animation.AnimationUtils; import android.widget.Button; import android.widget.RelativeLayout; import androidx.appcompat.app.AppCompatActivity; public class MainActivity extends AppCompatActivity { // creating variables for buttons and relative layout. private Button closeBtn; private RelativeLayout relativeLayout; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); //initializing variable for relative layout and button on below line. closeBtn = findViewById(R.id.idBtnClose); relativeLayout = findViewById(R.id.idRLLayout); // on below line adding click listener for our button to close the application. closeBtn.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { // on below line creating a variable for animation and adding the animation to it which we are creating on below line. Animation anim = AnimationUtils.loadAnimation(getApplicationContext(), R.anim.animation); // on below line adding animation listener for the animation which have created. anim.setAnimationListener(new Animation.AnimationListener() { // on below line creating on animation start method @Override public void onAnimationStart(Animation animation) { } // on below line creating an on animation end method in which we will be closing our application. @Override public void onAnimationEnd(Animation animation) { // on below line closing our application by calling the finish method. finish(); } // on below lie creating on animation repeat method which will be called when animation is repeated. @Override public void onAnimationRepeat(Animation animation) { } }); // on below line we are adding animation by calling start animation method for our relative layout relativeLayout.startAnimation(anim); } }); } }
说明:在上面的代码中,我们首先为按钮和相对布局(我们的根布局)创建变量。现在我们将看到onCreate方法。这是每个Android应用程序的默认方法。当创建应用程序视图时,将调用此方法。在此方法中,我们设置内容视图,即名为activity_main.xml的布局文件,以从该文件中设置UI。在onCreate方法中,我们使用我们在activity_main.xml文件中提供的ID初始化按钮和相对布局变量。之后,我们为“关闭应用程序”按钮添加一个OnClickListener。在OnClickListener方法中,我们为我们的活动创建了一个动画,我们将在应用程序关闭时应用该动画。
在此动画中,我们传递我们创建的动画。之后,我们为我们的动画添加一个动画监听器。在其中,我们将看到几种方法,例如onAnimationStart(动画开始时调用),onAnimationEnd(动画完成时调用)。在此方法中,我们调用finish方法来关闭应用程序。之后,我们创建了一个onAnimationRepeat方法,当我们必须重复动画时将调用此方法。最后,我们将动画添加到我们的相对布局,并通过调用startAnimation方法启动我们的动画。
添加上述代码后,我们只需单击顶部的绿色图标即可在移动设备上运行我们的应用程序。
注意 - 确保已连接到您的真实设备或模拟器。
输出
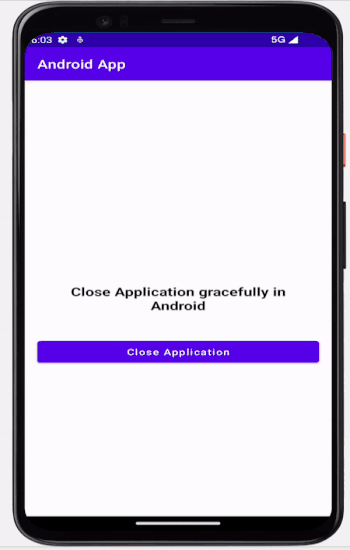
结论
在上面的文章中,我们了解了如何优雅地关闭Android应用程序?