使用Python滚动Instagram关注者弹出窗口
Instagram是一个流行的社交媒体平台,允许用户与关注者联系和分享内容。作为开发者,可能需要自动化Instagram上的某些任务,例如提取关注者数据。Instagram的关注者弹出窗口每次只加载有限数量的关注者,用户需要向下滚动才能查看更多关注者。在本文中,我们将探讨如何使用Python滚动Instagram关注者弹出窗口。
语法
webdriver.Chrome('path/to/chromedriver
此方法用于创建Chrome WebDriver的实例。它需要提供chromedriver可执行文件的路径作为参数。
driver.get(url)
此方法用于在网络浏览器中导航到指定的URL。它以URL作为参数,并加载相应的网页。
element = driver.find_element_by_name(name)
此方法用于根据其name属性在网页上查找HTML元素。它返回一个表示已找到元素的WebElement对象。
element.send_keys(*value)
此方法用于模拟键盘输入到输入字段或元素中。它以输入值作为参数,并将其输入到指定的元素中。
wait = WebDriverWait(driver, timeout)
此类用于在Selenium中设置显式等待。它以WebDriver实例和最大等待时间作为参数。
分步实施
步骤1:导入和设置环境:我们首先需要安装必要的依赖项,包括Python、selenium和相应的webdriver,并在你的Python脚本中导入这些库。可以使用Python包管理器pip安装这些库。你可以如下导入所需的库
from selenium import webdriver from selenium.webdriver.common.by import By from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.support import expected_conditions as EC import time
步骤2:初始化WebDriver:要初始化web驱动程序,需要创建一个webdriver.Chrome的实例,并提供Chrome驱动程序可执行文件的路径。你可以如下初始化ChromeDriver
from selenium import webdriver driver = webdriver.Chrome('path/to/chromedriver')
步骤3:导航到Instagram登录页面:使用driver.get()方法可以导航到Instagram登录页面。导航到页面后,使用Selenium的find_element_by_name方法找到用户名和密码输入字段。
# Navigate to Instagram's login page driver.get('https://www.instagram.com/accounts/login/') # Wait for the page to load time.sleep(2) # Find the username and password input fields, and fill in the login credentials username_input = driver.find_element_by_name('username') password_input = driver.find_element_by_name('password')
步骤4:填写登录凭据:使用find_element_by_name()方法找到用户名和密码输入字段后,可以使用相应输入字段上的send_keys()方法将登录凭据传递给输入字段。
username_input.send_keys('your_username') # Replace 'your_username' with your Instagram username password_input.send_keys('your_password') # Replace 'your_password' with your Instagram password
步骤5:提交登录表单:传递凭据后,现在可以通过调用密码输入字段上的submit()方法提交登录表单。
# Submit the login form password_input.submit()
步骤6:导航到用户的个人资料页面:登录成功后,可以使用WebDriver的get()方法导航到用户的个人资料页面。将'your_username'替换为你的Instagram用户名。你还可以使用time.sleep()方法等待页面完全加载。
# Wait for the login process to complete time.sleep(5) # Navigate to the user's profile page driver.get('https://www.instagram.com/your_username') # Replace 'your_username' with your Instagram username # Wait for the profile page to load time.sleep(2)
步骤7:点击关注者按钮:可以使用find_element_by_xpath()方法和XPath选择器在个人资料页面上找到关注者按钮。WebDriverWait类用于等待页面上出现该元素。然后使用click()方法点击关注者按钮。
# Find and click on the followers button followers_button = WebDriverWait(driver, 10).until( EC.presence_of_element_located((By.XPATH, '//a[@href="/your_username/followers/"]')) ) # Replace 'your_username' with your Instagram username followers_button.click()
步骤8:等待关注者弹出窗口加载:我们使用WebDriverWait等待关注者弹出窗口出现在页面上。然后使用find_element_by_xpath()方法和XPath选择器找到关注者弹出窗口。
# Wait for the followers popup to load followers_popup = WebDriverWait(driver, 10).until( EC.presence_of_element_located((By.XPATH, '//div[@class="isgrP"]')) )
步骤9:滚动关注者弹出窗口:定义一个JavaScript脚本将关注者弹出窗口滚动到底部。该脚本将元素的scrollTop属性设置为其scrollHeight,这会将其滚动到最大高度。我们使用while循环重复执行滚动脚本,直到没有加载新的关注者。在循环中,我们跟踪滚动前后关注者的数量。如果关注者的数量保持不变,则表示没有加载新的关注者,我们退出循环。
# Scroll down the followers popup scroll_script = "arguments[0].scrollTop = arguments[0].scrollHeight;" while True: last_count = len(driver.find_elements_by_xpath('//div[@class="isgrP"]//li')) driver.execute_script(scroll_script, followers_popup) time.sleep(1) # Add a delay to allow time for the followers to load new_count = len(driver.find_elements_by_xpath('//div[@class="isgrP"]//li')) if new_count == last_count: break # Exit the loop if no new followers are loaded
步骤10:关闭网页浏览器
Call the quit() method on the WebDriver to close the web browser and free system resources.
输出
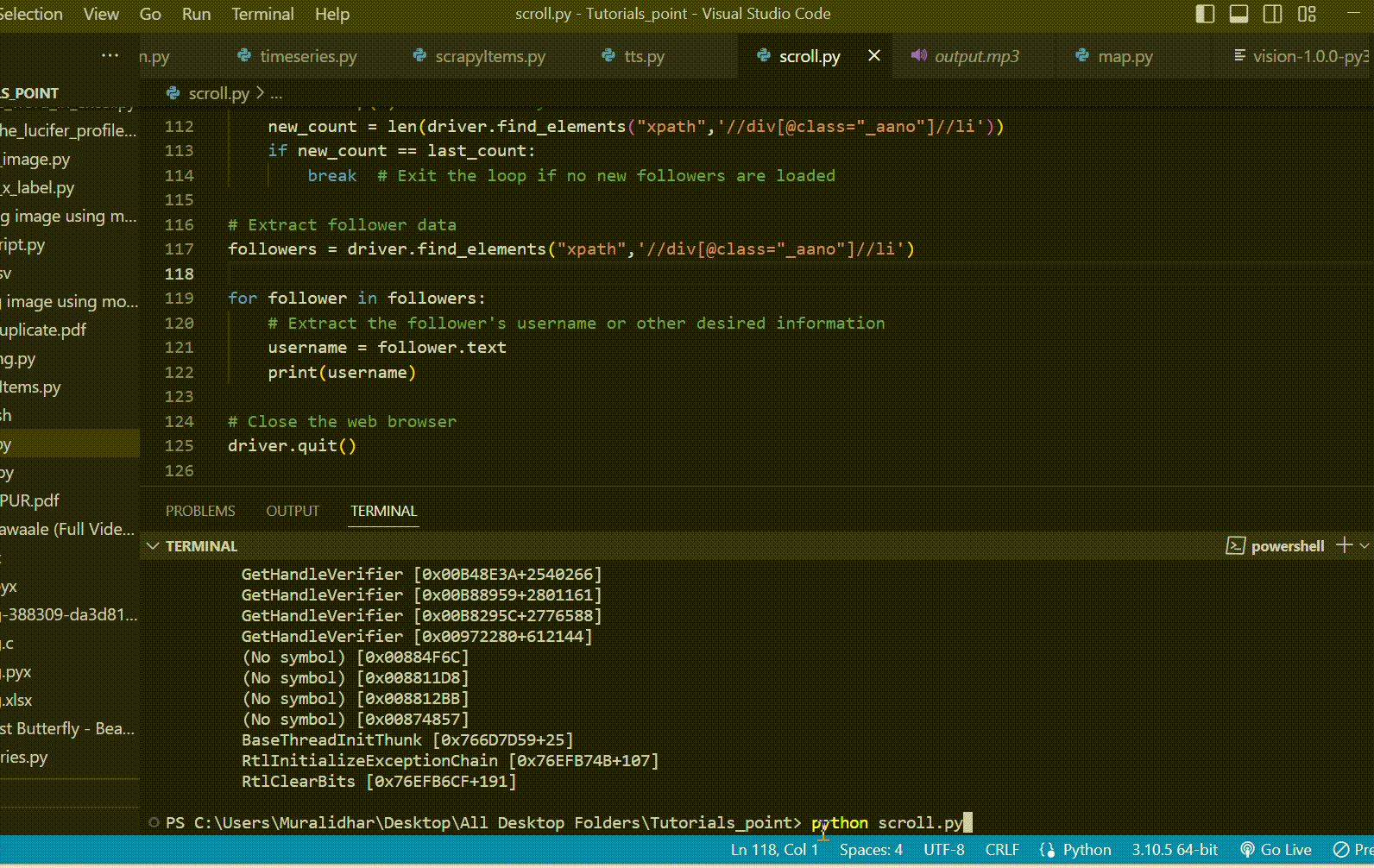
结论
在本文中,我们讨论了如何使用Python中的Selenium滚动Instagram关注者弹出窗口。通过利用Python和Selenium WebDriver,我们可以高效地滚动Instagram上的关注者弹出窗口并提取关注者数据。在本文中,我们讨论了分步过程,并提供了示例代码片段来帮助你入门。