如何在 ReactJS 中使用 axios 将一个或多个文件发送到 API?
ReactJS 是一个前端库,因此我们可以使用它来创建网页。在实时应用程序中显示数据是很自然的,并且后端管理它。因此,每当 React 需要在网页上显示数据时,它都会通过发出 API 调用来从后端获取数据。
有时,我们可能需要将数据发送到后端以将其存储在数据库中。例如,我们获取了用户的个人资料图片,需要将其发送到后端以将其存储在数据库中。
在许多情况下,我们需要使用 ReactJs 将单个或多个文件发送到后端。例如,某些剽窃检查工具允许上传多个文件以检查内容中的剽窃情况。
本教程将使用 axios 在 ReactJs 中将单个或多个文件发送到后端。
创建应用程序并发送多个文件的步骤
这里,我们将创建两个应用程序。一个是 React 应用程序,我们将用于前端部分,另一个是 Node 应用程序,我们将用作后端。
创建 React 应用程序
步骤 1 – 使用以下命令在项目目录中创建一个 React 应用程序。
npx create-react-app app-name
在上述命令中,app-name 是应用程序的名称,用户可以用任何名称替换它。
步骤 2 – 现在,使用以下命令并在 React 应用程序中安装 axios NPM 包。
npm i axios
步骤 3 – 创建一个可以处理多个文件的输入文件。此外,创建一个提交按钮,当用户点击它时,它应该调用一个函数,该函数使用多个文件向后端发出 POST 请求。
步骤 4 – 创建一个 uploadMultipleFiles() 函数,它可以使用 axios 发出 POST 请求。
uploadMultipleFiles() { axios({ url: "https://127.0.0.1:8080/addFiles", method: "POST", data: { allFiles: files }, }) }
在上面的代码中,用户可以观察到我们使用了后端的端点并将“POST”作为方法的值来向后端发出 POST 请求。此外,我们在发出请求时传递了数据对象,我们可以在后端接收并操作这些文件。
步骤 5 – 接下来,用户可以将以下代码添加到应用程序的 App.js 文件中。
import React from "react"; import axios from "axios"; class App extends React.Component { state = { allFiles: [], }; changeFileInput(event) { // using the setState method to set uploaded files to allFiles in the state object let files = event.target.files; this.setState({ allFiles: files }); } uploadMultipleFiles() { // get files from the state let uploadedFiles = this.state.allFiles; let files = []; // get the name of the files and push them to the files array //users can also get files content, convert it to blob format and send it to the backend for (let the file of uploadedFiles) { files.push({ name: file.name }); } axios({ url: "https://127.0.0.1:8080/addFiles", // URL to make request method: "POST", // post method to send data data: { allFiles: files }, // attaching the files }) .then((res) => { // handle response console.log(res); }) .catch((err) => { // handle errors console.error(err); }); } render() { return ( <div> <h2> {" "} Using the <i> axios </i> to upload multiple files on NodeJs server.{" "} </h2> <input Type = "file" multiple // adding multiple attributes to allow users to upload multiple files. onChange = {(event) => this.changeFileInput(event)} /> <button onClick = {() => this.uploadMultipleFiles()}> Send Multiple Files to the server </button> </div> ); } } export default App;
在上面的代码中,我们创建了文件输入。每当用户上传多个文件时,我们都会调用 changeFileInput() 函数,该函数将所有文件设置为状态对象中的 allFiles 变量。
当用户点击按钮时,它会调用 uplaodMultipleFiles() 函数,该函数从状态中访问所有文件并创建文件对象的数组。但是,我们只在对象中添加了文件名,但用户可以访问文件内容,将其转换为 blob 对象,并将其添加到文件对象中。
之后,我们使用 axios 向后端发出 POST 请求并将对象作为数据值传递。
步骤 6 – 作为最后一步,用户需要使用以下命令运行应用程序。
npm start
创建 Node 应用程序
步骤 1 – 在终端中输入以下命令以创建一个 Node 应用程序。
node init -y
步骤 2 – 现在,在 Node 项目中安装所需的依赖项。用户应在终端中输入以下命令以在应用程序中安装依赖项。
npm i express cors body-parser
我们将使用 express 创建服务器,使用 cors 作为中间件,允许我们从前端到后端发送 POST 请求。body-parse 将解析我们在从前端发出 POST 请求时传递的数据。
步骤 3 – 在 Node 应用程序中,使用以下代码设置服务器。
var express = require("express"); var app = express(); app.listen(8080, function () { console.log("server started successfully"); });
步骤 4 – 现在,在“/addFiles”路由上处理 POST 请求。在回调函数中,使用以下代码从主体获取文件。
app.post("/addFiles", function (req, res) { // get files from the body here });
步骤 5 – 用户可以将以下完整代码添加到 server.js 文件中。如果项目中不存在 server.js 文件,用户可以创建一个名为 server.js 的文件。
// Importing the required and installed modules var express = require("express"); var cors = require("cors"); var app = express(); const bodyParser = require("body-parser"); // using middleware with app app.use(cors()); app.use(bodyParser.urlencoded({ extended: false })); app.use(bodyParser.json()); // allow users to make a post request. app.post("/addFiles", function (req, res) { let allFiles = req.body; console.log(allFiles); }); // Allowing the app to listen on port 8080 app.listen(8080, function () { console.log("server started successfully"); });
在上面的代码中,我们导入了所需的库。之后,我们在 Node 应用程序中设置了 express 服务器。此外,我们使用了 body-parse 从主体中提取数据,并在 express 应用程序中使用 cors 作为中间件。
之后,我们创建了处理文件的端点并在控制台中打印所有文件。
步骤 6 – 我们已准备好处理 Node 应用程序上的 POST 请求。用户需要通过在终端上运行以下命令来运行 Node 应用程序。
node server.js
输出
现在,用户有两个应用程序在不同的端口上运行。用户可以在下图中看到 React 应用程序的输出。
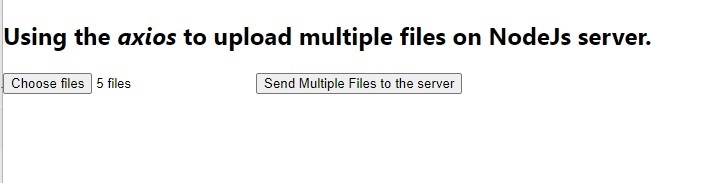
当我们在网页上上传多个文件时,Node 应用程序会在控制台中打印所有文件名,如下面的图像所示。
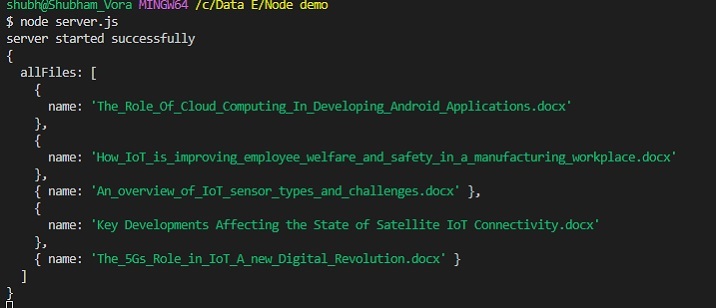
我们学习了如何使用 axios POST 请求将多个文件发送到后端。但是,在本教程中我们只发送了文件名,但用户也可以发送文件内容和更新日期等。