如何在Android中使用HTTP Client发送JSON格式的POST请求?
简介
许多Android应用程序在其内部使用API与数据库交互,并对数据库执行读写操作。我们可以使用多个库在Android应用程序中进行API调用。本文将介绍如何在Android中使用HTTP Client发送JSON格式的POST请求。
实现
我们将创建一个简单的应用程序,首先显示应用程序标题。然后,我们将创建两个EditText用于获取姓名和职位。接下来,我们将创建一个按钮用于发布这些数据。
步骤1:在Android Studio中创建一个新项目
导航到Android Studio,如下图所示。在下图中,单击“新建项目”以创建一个新的Android Studio项目。
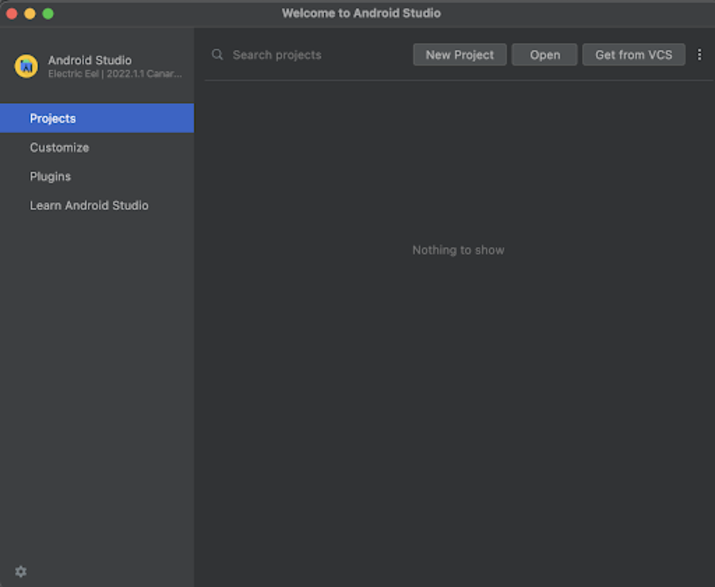
单击“新建项目”后,您将看到如下屏幕。
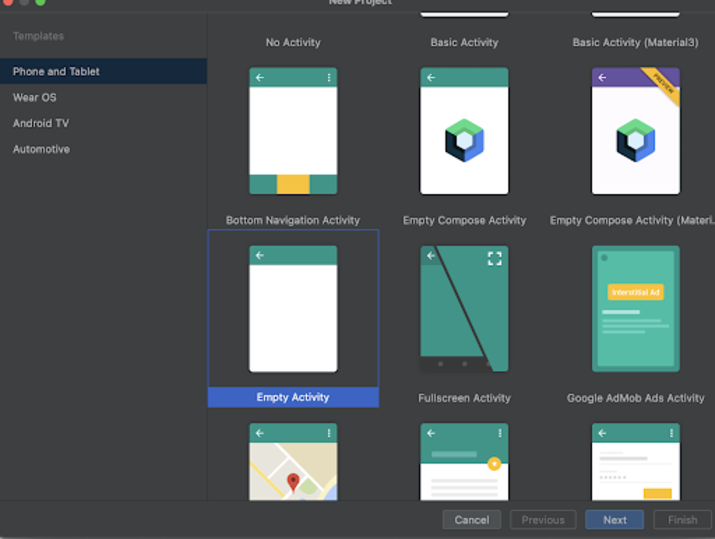
在这个屏幕中,我们只需选择“Empty Activity”并单击“Next”。单击“Next”后,您将看到如下屏幕。
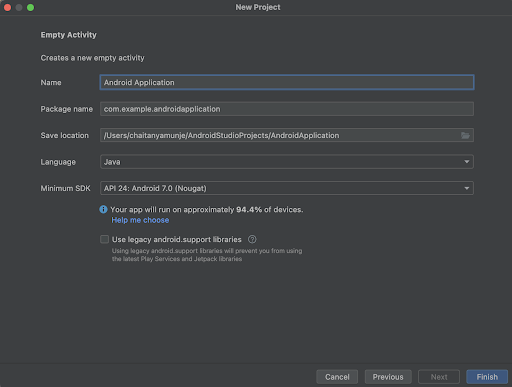
在这个屏幕中,我们只需指定项目名称。然后包名将自动生成。
注意 – 确保选择Java作为语言。
指定所有详细信息后,单击“Finish”以创建一个新的Android Studio项目。
项目创建完成后,我们将看到两个打开的文件:activity_main.xml和MainActivity.java文件。
步骤2:使用activity_main.xml
导航到activity_main.xml。如果此文件不可见,则要打开此文件。在左侧窗格中,导航到app>res>layout>activity_main.xml以打开此文件。打开此文件后,向其中添加以下代码。代码中添加了注释以便详细了解。
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/idRLLayout" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity"> <!-- text view for displaying heading of the application --> <TextView android:id="@+id/idTVHeading" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginStart="10dp" android:layout_marginTop="100dp" android:layout_marginEnd="10dp" android:padding="5dp" android:text="POST Request in Android using HTTP Client" android:textAlignment="center" android:textAllCaps="false" android:textColor="@color/black" android:textSize="18sp" android:textStyle="bold" /> <!-- on below line creating edit text to enter name--> <EditText android:id="@+id/idEdtName" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_below="@id/idTVHeading" android:layout_margin="10dp" android:hint="Enter Name" /> <!-- on below line creating edit text to enter job--> <EditText android:id="@+id/idEdtJob" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_below="@id/idEdtName" android:layout_margin="10dp" android:hint="Enter Job" /> <!-- on below line we are creating a button to post the data --> <Button android:id="@+id/idBtnPostData" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_below="@id/idEdtJob" android:layout_margin="20dp" android:text="Post Data" android:textAllCaps="false" /> </RelativeLayout>
解释 – 在上面的代码中,根元素是Android中的相对布局。此布局是一个视图组,用于相对于彼此对齐其中的所有元素。我们可以借助ID或位置在相对布局中相对对齐所有元素。
在这个相对布局中,我们创建了一个TextView用于显示应用程序的标题。之后,我们创建了两个EditText,用户可以在其中输入他们的姓名和职位详细信息。之后,我们创建了一个按钮,用于将数据发布到API。
步骤3:在AndroidManifest.xml文件中添加Internet权限
由于我们使用URL从Internet获取数据,因此我们必须在Android应用程序中添加Internet权限才能访问Internet以加载此数据。因此,我们必须添加Internet权限。要添加Internet权限,请导航到app>AndroidManifest.xml文件,并在application标签上方添加以下权限。
<uses-permission android:name="android.permission.INTERNET"/>
步骤7:使用MainActivity.java。
导航到MainActivity.java。如果此文件不可见,则要打开此文件。在左侧窗格中,导航到app>java>您的应用程序包名>MainActivity.java以打开此文件。打开此文件后,向其中添加以下代码。代码中添加了注释以便详细了解。
package com.example.androidjavaapp; import android.content.Context; import android.media.AudioManager; import android.media.Ringtone; import android.media.RingtoneManager; import android.net.Uri; import android.os.AsyncTask; import android.os.Bundle; import android.os.Handler; import android.util.Log; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.Toast; import androidx.appcompat.app.AppCompatActivity; import com.android.volley.toolbox.HttpClientStack; import org.json.JSONObject; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.io.OutputStream; import java.net.HttpURLConnection; import java.net.URL; public class MainActivity extends AppCompatActivity { // on below line creating a variable for button and edit text. private Button postDataBtn; private EditText nameEdt, jobEdt; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); // on below line we are initializing variables. postDataBtn = findViewById(R.id.idBtnPostData); nameEdt = findViewById(R.id.idEdtName); jobEdt = findViewById(R.id.idEdtJob); postDataBtn.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { if (nameEdt.getText().toString().isEmpty() && jobEdt.getText().toString().isEmpty()) { Toast.makeText(MainActivity.this, "Please enter name and job", Toast.LENGTH_SHORT).show(); } else { try { JSONObject jsonObject = new JSONObject(); jsonObject.put("name", nameEdt.getText().toString()); jsonObject.put("job", jobEdt.getText().toString()); String jsonString = jsonObject.toString(); new PostData().execute(jsonString); } catch (Exception e) { e.printStackTrace(); } } } }); } // on below line creating a class to post the data. class PostData extends AsyncTask<String, Void, String> { @Override protected String doInBackground(String... strings) { try { // on below line creating a url to post the data. URL url = new URL("https://reqres.in/api/users"); // on below line opening the connection. HttpURLConnection client = (HttpURLConnection) url.openConnection(); // on below line setting method as post. client.setRequestMethod("POST"); // on below line setting content type and accept type. client.setRequestProperty("Content-Type", "application/json"); client.setRequestProperty("Accept", "application/json"); // on below line setting client. client.setDoOutput(true); // on below line we are creating an output stream and posting the data. try (OutputStream os = client.getOutputStream()) { byte[] input = strings[0].getBytes("utf-8"); os.write(input, 0, input.length); } // on below line creating and initializing buffer reader. try (BufferedReader br = new BufferedReader( new InputStreamReader(client.getInputStream(), "utf-8"))) { // on below line creating a string builder. StringBuilder response = new StringBuilder(); // on below line creating a variable for response line. String responseLine = null; // on below line writing the response while ((responseLine = br.readLine()) != null) { response.append(responseLine.trim()); } // on below line displaying a toast message. Toast.makeText(MainActivity.this, "Data has been posted to the API.", Toast.LENGTH_SHORT).show(); } } catch (Exception e) { // on below line handling the exception. e.printStackTrace(); Toast.makeText(MainActivity.this, "Fail to post the data : " + e.getMessage(), Toast.LENGTH_SHORT).show(); } return null; } } }
解释 – 在上面的MainActivity.java文件的代码中,首先我们为EditText和按钮创建一个变量。现在我们将看到onCreate方法。这是每个Android应用程序的默认方法。当创建应用程序视图时,将调用此方法。在此方法中,我们将设置内容视图,即名为activity_main.xml的布局文件,以设置该文件中的UI。
指定视图后,我们使用在activity_main.xml文件中指定的唯一ID初始化EditText和按钮的变量。
初始化变量后,我们为按钮添加一个onClick监听器,并在onClick中检查EditText字段是否为空。如果为空,则显示吐司消息。如果不为空,则从中创建一个JSON对象,将该JSON对象转换为字符串,并将该JSON对象传递给PostData类。
PostData是一个异步任务类,我们使用HTTP Client在其中进行API调用以将数据发布到我们的API。
添加上述代码后,我们只需单击顶部栏中的绿色图标即可在移动设备上运行我们的应用程序。
注意 – 确保您已连接到您的真实设备或模拟器。
输出
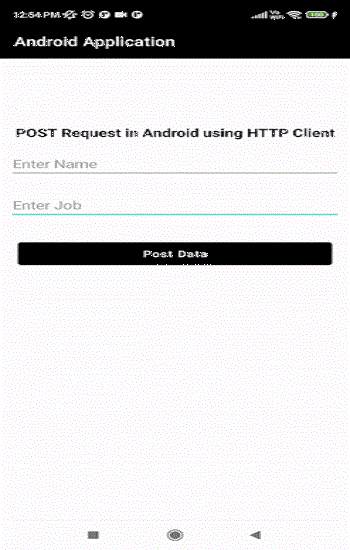
结论
在上面的教程中,我们了解了Android中的HTTP Client是什么,以及我们如何在Android中使用此HTTP Client来发布数据。