如何使用Tkinter通过下拉菜单打开文件夹中的文件?
在本教程中,我们将探讨如何使用Tkinter构建一个简单的文件查看器,允许用户使用下拉菜单从特定文件夹中打开文件。在本教程结束时,您将更好地理解Tkinter以及如何创建交互式文件选择界面。
什么是Tkinter?
Tkinter是Python自带的标准GUI工具包。它提供了一套用于创建图形用户界面的工具和部件。如果您没有安装Tkinter,可以使用以下命令安装:
pip install tk
现在您已经安装了Tkinter,让我们深入了解构建文件查看器的过程。
实现示例
我们将从创建一个具有必要组件的基本Tkinter窗口开始构建文件查看器的过程。Tkinter窗口将包括一个文本部件来显示文件内容、一个选择文件夹的按钮、一个下拉菜单来选择文件以及另一个打开所选文件的按钮。让我们查看下面的Python实现代码:
import tkinter as tk from tkinter import filedialog import os def open_file(): selected_file = file_var.get() file_path = os.path.join(folder_path, selected_file) with open(file_path, 'r') as file: content = file.read() text.delete(1.0, tk.END) # Clear previous content text.insert(tk.END, content) def update_dropdown(): file_list = os.listdir(folder_path) file_var.set(file_list[0] if file_list else "") file_menu['menu'].delete(0, 'end') for file_name in file_list: file_menu['menu'].add_command(label=file_name, command=tk._setit(file_var, file_name)) def select_folder(): global folder_path folder_path = filedialog.askdirectory() if folder_path: update_dropdown() # Create the main window root = tk.Tk() root.title("Using Tkinter to open file in folder using dropdown") root.geometry("720x300") # Create a variable to store the selected file file_var = tk.StringVar() # Create a Text widget to display file content text = tk.Text(root, wrap="word", height=10, width=40) text.pack(pady=10) # Create a button to select a folder folder_button = tk.Button(root, text="Select Folder", command=select_folder) folder_button.pack() # Create a dropdown menu to select a file file_menu = tk.OptionMenu(root, file_var, "") file_menu.pack() # Create a button to open the selected file open_button = tk.Button(root, text="Open File", command=open_file) open_button.pack() # Run the Tkinter event loop root.mainloop()
这段代码设置了一个具有文件查看器必要组件的基本Tkinter窗口。`select_folder`函数使用`filedialog.askdirectory`方法提示用户选择文件夹,`update_dropdown`函数使用所选文件夹中的文件列表更新下拉菜单。
输出
运行此代码后,您将获得以下输出窗口:
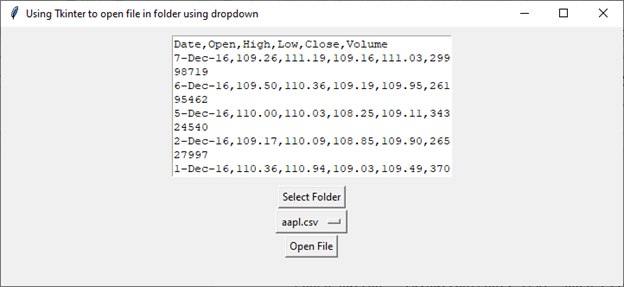
代码详解
让我们分解代码的关键组成部分:
`open_file`函数 - 当点击“打开文件”按钮时调用此函数。它从下拉菜单中检索所选文件,构造文件路径,读取文件内容,并在Tkinter文本部件中显示它。
`update_dropdown`函数 - 此函数负责使用所选文件夹中的文件列表更新下拉菜单。它将默认选定文件设置为列表中的第一个文件,并为每个文件向下拉菜单添加命令。
`select_folder`函数 - 当点击“选择文件夹”按钮时触发此函数。它打开一个对话框,供用户选择文件夹。选择文件夹后,它将使用该文件夹中的文件更新下拉菜单。
Tkinter部件 - `Text`、`Button`和`OptionMenu`部件用于创建用户界面。`StringVar` (file_var) 用于存储所选文件。
结论
在本教程中,我们探讨了使用Python中的Tkinter构建文件查看器的过程。我们介绍了Tkinter窗口的基本设置,包括创建按钮、下拉菜单和处理用户交互。