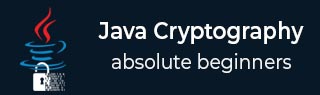
- Java 加密教程
- Java 加密 - 首页
- Java 加密 - 简介
- 消息摘要和MAC
- Java 加密 - 消息摘要
- Java 加密 - 创建MAC
- Java 加密资源
- Java 加密 - 快速指南
- Java 加密 - 资源
- Java 加密 - 讨论
Java 加密 - 快速指南
Java 加密 - 简介
密码学是设计能够提供信息安全的密码系统的艺术和科学。
密码学处理数字数据的实际保护。它指的是基于提供基本信息安全服务的数学算法的设计机制。您可以将密码学视为建立了一个包含安全应用程序中不同技术的庞大工具包。
什么是密码分析?
破解密文的艺术和科学被称为密码分析。
密码分析是密码学的姊妹分支,它们共同存在。密码过程产生用于传输或存储的密文。它涉及研究密码机制以试图破解它们。密码分析也用于新密码技术的设计中,以测试其安全性。
密码学原语
密码学原语只不过是密码学中的工具和技术,可以选择性地使用它们来提供一组所需的安全性服务 -
- 加密
- 哈希函数
- 消息认证码(MAC)
- 数字签名
Java中的密码学
Java 密码体系结构 (JCA) 是一组 API,用于实现现代密码学的概念,例如数字签名、消息摘要、证书、加密、密钥生成和管理以及安全随机数生成等。
使用 JCA,开发人员可以构建集成安全性的应用程序。
为了在您的应用程序中集成安全性,而不是依赖于复杂的安全性算法,您可以轻松地调用 JCA 中提供的相应 API 以获取所需的服务。
Java 加密 - 消息摘要
哈希函数非常有用,几乎出现在所有信息安全应用程序中。
哈希函数是一种数学函数,它将数值输入值转换为另一个压缩的数值值。哈希函数的输入长度任意,但输出始终是固定长度的。
哈希函数返回的值称为消息摘要或简称为哈希值。下图说明了哈希函数。
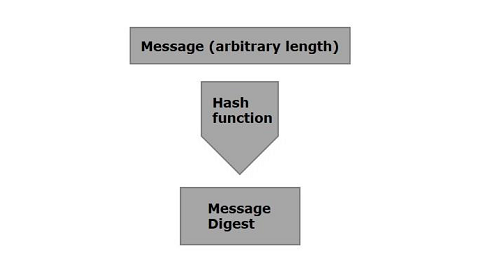
Java 提供了一个名为MessageDigest的类,它属于包 java.security。此类支持 SHA-1、SHA 256、MD5 等算法,用于将任意长度的消息转换为消息摘要。
要将给定消息转换为消息摘要,请按照以下步骤操作 -
步骤 1:创建 MessageDigest 对象
MessageDigest 类提供了一个名为getInstance()的方法。此方法接受一个指定要使用的算法名称的 String 变量,并返回一个实现指定算法的 MessageDigest 对象。
使用getInstance()方法创建 MessageDigest 对象,如下所示。
MessageDigest md = MessageDigest.getInstance("SHA-256");
步骤 2:将数据传递到创建的 MessageDigest 对象
创建消息摘要对象后,您需要将消息/数据传递给它。您可以使用MessageDigest类的update()方法来执行此操作,此方法接受表示消息的字节数组并将其添加到上面创建的 MessageDigest 对象中。
md.update(msg.getBytes());
步骤 3:生成消息摘要
您可以使用MessageDigest类的digest()方法生成消息摘要,此方法计算当前对象上的哈希函数,并以字节数组的形式返回消息摘要。
使用 digest 方法生成消息摘要。
byte[] digest = md.digest();
示例
以下是一个示例,它从文件中读取数据并生成消息摘要并打印它。
import java.security.MessageDigest; import java.util.Scanner; public class MessageDigestExample { public static void main(String args[]) throws Exception{ //Reading data from user Scanner sc = new Scanner(System.in); System.out.println("Enter the message"); String message = sc.nextLine(); //Creating the MessageDigest object MessageDigest md = MessageDigest.getInstance("SHA-256"); //Passing data to the created MessageDigest Object md.update(message.getBytes()); //Compute the message digest byte[] digest = md.digest(); System.out.println(digest); //Converting the byte array in to HexString format StringBuffer hexString = new StringBuffer(); for (int i = 0;i<digest.length;i++) { hexString.append(Integer.toHexString(0xFF & digest[i])); } System.out.println("Hex format : " + hexString.toString()); } }
输出
以上程序生成以下输出 -
Enter the message Hello how are you [B@55f96302 Hex format: 2953d33828c395aebe8225236ba4e23fa75e6f13bd881b9056a3295cbd64d3
Java 加密 - 创建MAC
MAC(Message Authentication Code)算法是一种对称密钥加密技术,用于提供消息认证。为了建立 MAC 过程,发送方和接收方共享一个对称密钥 K。
本质上,MAC 是对发送的消息生成的加密校验和,该校验和与消息一起发送以确保消息认证。
以下插图描述了使用 MAC 进行身份验证的过程 -
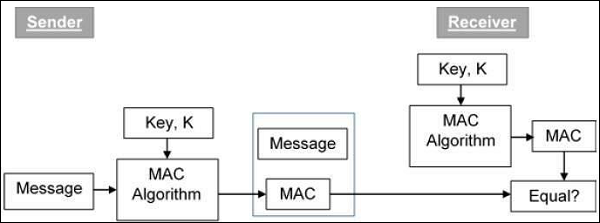
在 Java 中,javax.crypto包的Mac类提供了消息认证码的功能。请按照以下步骤使用此类创建消息认证码。
步骤 1:创建 KeyGenerator 对象
KeyGenerator类提供getInstance()方法,该方法接受一个表示所需密钥生成算法的 String 变量,并返回一个生成秘密密钥的KeyGenerator对象。
使用getInstance()方法创建KeyGenerator对象,如下所示。
//Creating a KeyGenerator object KeyGenerator keyGen = KeyGenerator.getInstance("DES");
步骤 2:创建 SecureRandom 对象
java.Security包的SecureRandom类提供了一个强大的随机数生成器,用于在 Java 中生成随机数。实例化此类,如下所示。
//Creating a SecureRandom object SecureRandom secRandom = new SecureRandom();
步骤 3:初始化 KeyGenerator
KeyGenerator类提供了一个名为init()的方法,此方法接受SecureRandom对象并初始化当前KeyGenerator。
使用此方法初始化上一步中创建的 KeyGenerator 对象。
//Initializing the KeyGenerator keyGen.init(secRandom);
步骤 4:生成密钥
使用KeyGenerator类的generateKey()方法生成密钥,如下所示。
//Creating/Generating a key Key key = keyGen.generateKey();
步骤 5:初始化 Mac 对象
Mac 类的init()方法接受一个 Key 对象并使用给定密钥初始化当前 Mac 对象。
//Initializing the Mac object mac.init(key);
步骤 6:完成 mac 操作
Mac 类的doFinal()方法用于完成 Mac 操作。将所需数据以字节数组的形式传递给此方法并完成操作,如下所示。
//Computing the Mac String msg = new String("Hi how are you"); byte[] bytes = msg.getBytes(); byte[] macResult = mac.doFinal(bytes);
示例
以下示例演示了如何使用 JCA 生成消息认证码 (MAC)。在这里,我们取一个简单的消息“Hi how are you”,并为该消息生成一个 Mac。
import java.security.Key; import java.security.SecureRandom; import javax.crypto.KeyGenerator; import javax.crypto.Mac; public class MacSample { public static void main(String args[]) throws Exception{ //Creating a KeyGenerator object KeyGenerator keyGen = KeyGenerator.getInstance("DES"); //Creating a SecureRandom object SecureRandom secRandom = new SecureRandom(); //Initializing the KeyGenerator keyGen.init(secRandom); //Creating/Generating a key Key key = keyGen.generateKey(); //Creating a Mac object Mac mac = Mac.getInstance("HmacSHA256"); //Initializing the Mac object mac.init(key); //Computing the Mac String msg = new String("Hi how are you"); byte[] bytes = msg.getBytes(); byte[] macResult = mac.doFinal(bytes); System.out.println("Mac result:"); System.out.println(new String(macResult)); } }
输出
以上程序将生成以下输出 -
Mac result: HÖ„^ǃÎ_Utbh…?š_üzØSSÜh_ž_œa0ŽV?
Java 加密 - 密钥
密码系统是加密技术的实现及其伴随的基础设施,用于提供信息安全服务。密码系统也称为密码系统。
基本密码系统的各个组成部分是明文、加密算法、密文、解密算法、加密密钥和解密密钥。
其中,
加密密钥是发送方已知的值。发送方将加密密钥与明文一起输入加密算法,以计算密文。
解密密钥是接收方已知的值。解密密钥与加密密钥相关,但不总是与其相同。接收方将解密密钥与密文一起输入解密算法,以计算明文。
从根本上讲,根据加密解密算法的类型,密钥/密码系统有两种类型。
对称密钥加密
使用相同的密钥加密和解密信息的加密过程称为对称密钥加密。
对称密码系统的研究称为对称密码学。对称密码系统有时也称为秘密密钥密码系统。
以下是一些常见的对称密钥加密示例 -
- 数字加密标准 (DES)
- 三重 DES (3DES)
- IDEA
- BLOWFISH
非对称密钥加密
使用不同的密钥加密和解密信息的加密过程称为非对称密钥加密。虽然密钥不同,但它们在数学上是相关的,因此,通过解密密文检索明文是可行的。
Java 加密 - 存储密钥
使用的/生成的密钥和证书存储在称为密钥库的数据库中。默认情况下,此数据库存储在一个名为.keystore的文件中。
您可以使用java.security包的KeyStore类访问此数据库的内容。它管理三个不同的条目,即 PrivateKeyEntry、SecretKeyEntry、TrustedCertificateEntry。
- PrivateKeyEntry
- SecretKeyEntry
- TrustedCertificateEntry
将密钥存储在密钥库中
在本节中,我们将学习如何将密钥存储在密钥库中。要将密钥存储在密钥库中,请按照以下步骤操作。
步骤 1:创建 KeyStore 对象
java.security包的KeyStore类的getInstance()方法接受一个表示密钥库类型的字符串值,并返回一个 KeyStore 对象。
使用getInstance()方法创建 KeyStore 类的对象,如下所示。
//Creating the KeyStore object KeyStore keyStore = KeyStore.getInstance("JCEKS");
步骤 2:加载 KeyStore 对象
KeyStore 类的load()方法接受一个表示密钥库文件的 FileInputStream 对象和一个指定 KeyStore 密码的 String 参数。
通常,KeyStore 存储在名为cacerts的文件中,位于C:/Program Files/Java/jre1.8.0_101/lib/security/位置,其默认密码为changeit,使用load()方法加载它,如下所示。
//Loading the KeyStore object char[] password = "changeit".toCharArray(); String path = "C:/Program Files/Java/jre1.8.0_101/lib/security/cacerts"; java.io.FileInputStream fis = new FileInputStream(path); keyStore.load(fis, password);
步骤 3:创建 KeyStore.ProtectionParameter 对象
实例化 KeyStore.ProtectionParameter,如下所示。
//Creating the KeyStore.ProtectionParameter object KeyStore.ProtectionParameter protectionParam = new KeyStore.PasswordProtection(password);
步骤 4:创建 SecretKey 对象
通过实例化其子类SecretKeySpec来创建SecretKey(接口)对象。在实例化时,您需要将密码和算法作为参数传递给其构造函数,如下所示。
//Creating SecretKey object SecretKey mySecretKey = new SecretKeySpec(new String(keyPassword).getBytes(), "DSA");
步骤 5:创建 SecretKeyEntry 对象
通过传递在上面步骤中创建的SecretKey对象,创建SecretKeyEntry类的对象,如下所示。
//Creating SecretKeyEntry object KeyStore.SecretKeyEntry secretKeyEntry = new KeyStore.SecretKeyEntry(mySecretKey);
步骤 6:将条目设置为 KeyStore
KeyStore类的setEntry()方法接受一个表示密钥库条目别名的字符串参数、一个SecretKeyEntry对象、一个ProtectionParameter对象,并将条目存储在给定的别名下。
使用setEntry()方法将条目设置为密钥库,如下所示。
//Set the entry to the keystore keyStore.setEntry("secretKeyAlias", secretKeyEntry, protectionParam);
示例
以下示例将密钥存储到“cacerts”文件(Windows 10 操作系统)中存在的密钥库中。
import java.io.FileInputStream; import java.security.KeyStore; import javax.crypto.SecretKey; import javax.crypto.spec.SecretKeySpec; public class StoringIntoKeyStore{ public static void main(String args[]) throws Exception { //Creating the KeyStore object KeyStore keyStore = KeyStore.getInstance("JCEKS"); //Loading the KeyStore object char[] password = "changeit".toCharArray(); String path = "C:/Program Files/Java/jre1.8.0_101/lib/security/cacerts"; java.io.FileInputStream fis = new FileInputStream(path); keyStore.load(fis, password); //Creating the KeyStore.ProtectionParameter object KeyStore.ProtectionParameter protectionParam = new KeyStore.PasswordProtection(password); //Creating SecretKey object SecretKey mySecretKey = new SecretKeySpec("myPassword".getBytes(), "DSA"); //Creating SecretKeyEntry object KeyStore.SecretKeyEntry secretKeyEntry = new KeyStore.SecretKeyEntry(mySecretKey); keyStore.setEntry("secretKeyAlias", secretKeyEntry, protectionParam); //Storing the KeyStore object java.io.FileOutputStream fos = null; fos = new java.io.FileOutputStream("newKeyStoreName"); keyStore.store(fos, password); System.out.println("data stored"); } }
输出
以上程序生成以下输出 -
System.out.println("data stored");
Java 加密 - 检索密钥
在本章中,我们将学习如何使用 Java 加密从密钥库中检索密钥。
要从密钥库中检索密钥,请按照以下步骤操作。
步骤 1:创建 KeyStore 对象
java.security包的KeyStore类的getInstance()方法接受一个表示密钥库类型的字符串值,并返回一个 KeyStore 对象。
使用此方法创建 KeyStore 类的对象,如下所示。
//Creating the KeyStore object KeyStore keyStore = KeyStore.getInstance("JCEKS");
步骤 2:加载 KeyStore 对象
KeyStore 类的load()方法接受一个表示密钥库文件的FileInputStream对象和一个指定 KeyStore 密码的字符串参数。
通常,KeyStore 存储在名为cacerts的文件中,位于C:/Program Files/Java/jre1.8.0_101/lib/security/位置,其默认密码为changeit,使用load()方法加载它,如下所示。
//Loading the KeyStore object char[] password = "changeit".toCharArray(); String path = "C:/Program Files/Java/jre1.8.0_101/lib/security/cacerts"; java.io.FileInputStream fis = new FileInputStream(path); keyStore.load(fis, password);
步骤 3:创建 KeyStore.ProtectionParameter 对象
实例化 KeyStore.ProtectionParameter,如下所示。
//Creating the KeyStore.ProtectionParameter object KeyStore.ProtectionParameter protectionParam = new KeyStore.PasswordProtection(password);
步骤 4:创建 SecretKey 对象
通过实例化其子类SecretKeySpec来创建SecretKey(接口)对象。在实例化时,您需要将密码和算法作为参数传递给其构造函数,如下所示。
//Creating SecretKey object SecretKey mySecretKey = new SecretKeySpec(new String(keyPassword).getBytes(), "DSA");
步骤 5:创建 SecretKeyEntry 对象
通过传递在上面步骤中创建的SecretKey对象,创建SecretKeyEntry类的对象,如下所示。
//Creating SecretKeyEntry object KeyStore.SecretKeyEntry secretKeyEntry = new KeyStore.SecretKeyEntry(mySecretKey);
步骤 6:将条目设置为 KeyStore
KeyStore类的setEntry()方法接受一个表示密钥库条目别名的字符串参数、一个SecretKeyEntry对象、一个ProtectionParameter对象,并将条目存储在给定的别名下。
使用setEntry()方法将条目设置为密钥库,如下所示。
//Set the entry to the keystore keyStore.setEntry("secretKeyAlias", secretKeyEntry, protectionParam);
步骤 7:创建 KeyStore.SecretKeyEntry 对象
KeyStore类的getEntry()方法接受一个别名(字符串参数)和一个ProtectionParameter类的对象作为参数,并返回一个KeyStoreEntry对象,然后您可以将其转换为KeyStore.SecretKeyEntry对象。
通过将所需密钥的别名和在前面步骤中创建的保护参数对象传递给getEntry()方法,创建KeyStore.SecretKeyEntry类的对象,如下所示。
//Creating the KeyStore.SecretKeyEntry object KeyStore.SecretKeyEntry secretKeyEnt = (KeyStore.SecretKeyEntry)keyStore.getEntry("secretKeyAlias", protectionParam);
步骤 8:创建检索到的条目的密钥对象
SecretKeyEntry类的getSecretKey()方法返回一个SecretKey对象。使用此方法创建SecretKey对象,如下所示。
//Creating SecretKey object SecretKey mysecretKey = secretKeyEnt.getSecretKey(); System.out.println(mysecretKey);
示例
以下示例演示了如何从密钥库中检索密钥。在这里,我们将密钥存储在“cacerts”文件(Windows 10 操作系统)中的密钥库中,检索它,并显示其某些属性,例如用于生成密钥的算法和检索密钥的格式。
import java.io.FileInputStream; import java.security.KeyStore; import java.security.KeyStore.ProtectionParameter; import java.security.KeyStore.SecretKeyEntry; import javax.crypto.SecretKey; import javax.crypto.spec.SecretKeySpec; public class RetrievingFromKeyStore{ public static void main(String args[]) throws Exception{ //Creating the KeyStore object KeyStore keyStore = KeyStore.getInstance("JCEKS"); //Loading the the KeyStore object char[] password = "changeit".toCharArray(); java.io.FileInputStream fis = new FileInputStream( "C:/Program Files/Java/jre1.8.0_101/lib/security/cacerts"); keyStore.load(fis, password); //Creating the KeyStore.ProtectionParameter object ProtectionParameter protectionParam = new KeyStore.PasswordProtection(password); //Creating SecretKey object SecretKey mySecretKey = new SecretKeySpec("myPassword".getBytes(), "DSA"); //Creating SecretKeyEntry object SecretKeyEntry secretKeyEntry = new SecretKeyEntry(mySecretKey); keyStore.setEntry("secretKeyAlias", secretKeyEntry, protectionParam); //Storing the KeyStore object java.io.FileOutputStream fos = null; fos = new java.io.FileOutputStream("newKeyStoreName"); keyStore.store(fos, password); //Creating the KeyStore.SecretKeyEntry object SecretKeyEntry secretKeyEnt = (SecretKeyEntry)keyStore.getEntry("secretKeyAlias", protectionParam); //Creating SecretKey object SecretKey mysecretKey = secretKeyEnt.getSecretKey(); System.out.println("Algorithm used to generate key : "+mysecretKey.getAlgorithm()); System.out.println("Format used for the key: "+mysecretKey.getFormat()); } }
输出
以上程序生成以下输出 -
Algorithm used to generate key: DSA Format of the key: RAW
Java 加密 - KeyGenerator
Java 提供了KeyGenerator类,此类用于生成密钥和此类的可重用对象。
要使用 KeyGenerator 类生成密钥,请按照以下步骤操作。
步骤 1:创建 KeyGenerator 对象
KeyGenerator类提供getInstance()方法,该方法接受一个表示所需密钥生成算法的字符串变量,并返回一个生成密钥的KeyGenerator对象。
使用getInstance()方法创建KeyGenerator对象,如下所示。
//Creating a KeyGenerator object KeyGenerator keyGen = KeyGenerator.getInstance("DES");
步骤 2:创建 SecureRandom 对象
java.Security包的SecureRandom类提供了一个强大的随机数生成器,用于在 Java 中生成随机数。实例化此类,如下所示。
//Creating a SecureRandom object SecureRandom secRandom = new SecureRandom();
步骤 3:初始化 KeyGenerator
KeyGenerator类提供了一个名为init()的方法,此方法接受SecureRandom对象并初始化当前KeyGenerator。
使用init()方法初始化在前面步骤中创建的KeyGenerator对象。
//Initializing the KeyGenerator keyGen.init(secRandom);
示例
以下示例演示了使用javax.crypto包的KeyGenerator类生成密钥。
import javax.crypto.Cipher; import javax.crypto.KeyGenerator; import java.security.Key; import java.security.SecureRandom; public class KeyGeneratorExample { public static void main(String args[]) throws Exception{ //Creating a KeyGenerator object KeyGenerator keyGen = KeyGenerator.getInstance("DES"); //Creating a SecureRandom object SecureRandom secRandom = new SecureRandom(); //Initializing the KeyGenerator keyGen.init(secRandom); //Creating/Generating a key Key key = keyGen.generateKey(); System.out.println(key); Cipher cipher = Cipher.getInstance("DES/ECB/PKCS5Padding"); cipher.init(cipher.ENCRYPT_MODE, key); String msg = new String("Hi how are you"); byte[] bytes = cipher.doFinal(msg.getBytes()); System.out.println(bytes); } }
输出
以上程序生成以下输出 -
com.sun.crypto.provider.DESKey@18629 [B@2ac1fdc4
Java 加密 - KeyPairGenerator
Java 提供了KeyPairGenerator类。此类用于生成公钥和私钥对。要使用KeyPairGenerator类生成密钥,请按照以下步骤操作。
步骤 1:创建一个 KeyPairGenerator 对象
KeyPairGenerator类提供getInstance()方法,该方法接受一个表示所需密钥生成算法的字符串变量,并返回一个生成密钥的KeyPairGenerator对象。
使用getInstance()方法创建KeyPairGenerator对象,如下所示。
//Creating KeyPair generator object KeyPairGenerator keyPairGen = KeyPairGenerator.getInstance("DSA");
步骤 2:初始化 KeyPairGenerator 对象
KeyPairGenerator类提供了一个名为initialize()的方法,此方法用于初始化密钥对生成器。此方法接受一个表示密钥大小的整数值。
使用此方法初始化在前面步骤中创建的KeyPairGenerator对象,如下所示。
//Initializing the KeyPairGenerator keyPairGen.initialize(2048);
步骤 3:生成 KeyPairGenerator
您可以使用KeyPairGenerator类的generateKeyPair()方法生成KeyPair。使用此方法生成密钥对,如下所示。
//Generate the pair of keys KeyPair pair = keyPairGen.generateKeyPair();
步骤 4:获取私钥/公钥
您可以使用getPrivate()方法从生成的KeyPair对象中获取私钥,如下所示。
//Getting the private key from the key pair PrivateKey privKey = pair.getPrivate();
您可以使用getPublic()方法从生成的KeyPair对象中获取公钥,如下所示。
//Getting the public key from the key pair PublicKey publicKey = pair.getPublic();
示例
以下示例演示了使用javax.crypto包的KeyPairGenerator类生成密钥。
import java.security.KeyPair; import java.security.KeyPairGenerator; import java.security.PrivateKey; import java.security.PublicKey; public class KeyPairGenertor { public static void main(String args[]) throws Exception{ //Creating KeyPair generator object KeyPairGenerator keyPairGen = KeyPairGenerator.getInstance("DSA"); //Initializing the KeyPairGenerator keyPairGen.initialize(2048); //Generating the pair of keys KeyPair pair = keyPairGen.generateKeyPair(); //Getting the private key from the key pair PrivateKey privKey = pair.getPrivate(); //Getting the public key from the key pair PublicKey publicKey = pair.getPublic(); System.out.println("Keys generated"); } }
输出
以上程序生成以下输出 -
Keys generated
Java 加密 - 创建签名
数字签名允许我们验证签名者的身份、日期和时间,并对消息内容进行身份验证。它还包括用于其他功能的身份验证功能。
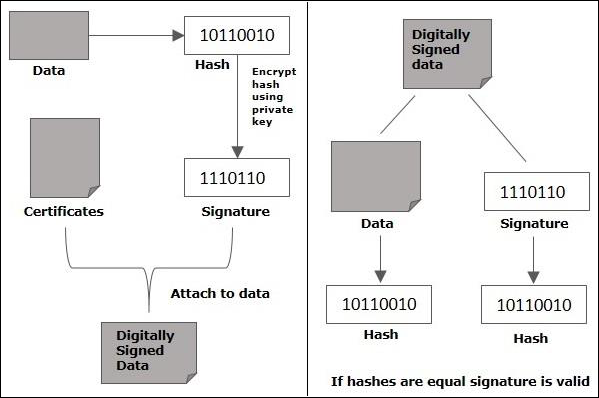
数字签名的优势
在本节中,我们将了解需要使用数字签名的不同原因。实施数字签名到通信中有几个原因:
身份验证
数字签名有助于对消息来源进行身份验证。例如,如果银行的分支机构向中央办公室发送消息,请求更改某个账户的余额。如果中央办公室无法验证该消息是否来自授权的来源,则对该请求采取行动可能是严重的错误。
完整性
消息一旦签名,任何对消息的更改都会使签名无效。
不可否认性
通过此属性,任何已签名某些信息的人员日后都不能否认已签名该信息。
创建数字签名
现在让我们学习如何创建数字签名。您可以按照以下步骤使用 Java 创建数字签名。
步骤 1:创建一个 KeyPairGenerator 对象
KeyPairGenerator类提供getInstance()方法,该方法接受一个表示所需密钥生成算法的字符串变量,并返回一个生成密钥的KeyPairGenerator对象。
使用getInstance()方法创建KeyPairGenerator对象,如下所示。
//Creating KeyPair generator object KeyPairGenerator keyPairGen = KeyPairGenerator.getInstance("DSA");
步骤 2:初始化 KeyPairGenerator 对象
KeyPairGenerator类提供了一个名为initialize()的方法,此方法用于初始化密钥对生成器。此方法接受一个表示密钥大小的整数值。
使用initialize()方法初始化在前面步骤中创建的KeyPairGenerator对象,如下所示。
//Initializing the KeyPairGenerator keyPairGen.initialize(2048);
步骤 3:生成 KeyPairGenerator
您可以使用generateKeyPair()方法生成KeyPair。使用generateKeyPair()方法生成密钥对,如下所示。
//Generate the pair of keys KeyPair pair = keyPairGen.generateKeyPair();
步骤 4:从对中获取私钥
您可以使用getPrivate()方法从生成的KeyPair对象中获取私钥。
使用getPrivate()方法获取私钥,如下所示。
//Getting the private key from the key pair PrivateKey privKey = pair.getPrivate();
步骤 5:创建一个签名对象
Signature类的getInstance()方法接受一个表示所需签名算法的字符串参数,并返回相应的Signature对象。
使用getInstance()方法创建Signature类的对象。
//Creating a Signature object Signature sign = Signature.getInstance("SHA256withDSA");
步骤 6:初始化签名对象
Signature类的initSign()方法接受一个PrivateKey对象并初始化当前的Signature对象。
使用initSign()方法初始化在前面步骤中创建的Signature对象,如下所示。
//Initialize the signature sign.initSign(privKey);
步骤 7:将数据添加到签名对象
Signature类的update()方法接受一个表示要签名或验证的数据的字节数组,并使用给定的数据更新当前对象。
通过将要签名的数据以字节数组的形式传递给update()方法,更新初始化的Signature对象,如下所示。
byte[] bytes = "Hello how are you".getBytes(); //Adding data to the signature sign.update(bytes);
步骤 8:计算签名
Signature类的sign()方法返回更新数据的签名字节。
使用sign()方法计算签名,如下所示。
//Calculating the signature byte[] signature = sign.sign();
示例
以下 Java 程序接受用户输入的消息,并为给定消息生成数字签名。
import java.security.KeyPair; import java.security.KeyPairGenerator; import java.security.PrivateKey; import java.security.Signature; import java.util.Scanner; public class CreatingDigitalSignature { public static void main(String args[]) throws Exception { //Accepting text from user Scanner sc = new Scanner(System.in); System.out.println("Enter some text"); String msg = sc.nextLine(); //Creating KeyPair generator object KeyPairGenerator keyPairGen = KeyPairGenerator.getInstance("DSA"); //Initializing the key pair generator keyPairGen.initialize(2048); //Generate the pair of keys KeyPair pair = keyPairGen.generateKeyPair(); //Getting the private key from the key pair PrivateKey privKey = pair.getPrivate(); //Creating a Signature object Signature sign = Signature.getInstance("SHA256withDSA"); //Initialize the signature sign.initSign(privKey); byte[] bytes = "msg".getBytes(); //Adding data to the signature sign.update(bytes); //Calculating the signature byte[] signature = sign.sign(); //Printing the signature System.out.println("Digital signature for given text: "+new String(signature, "UTF8")); } }
输出
以上程序生成以下输出 -
Enter some text Hi how are you Digital signature for given text: 0=@gRD???-?.???? /yGL?i??a!?
Java 加密 - 验证签名
您可以使用 Java 创建数字签名并按照以下步骤验证它。
步骤 1:创建一个 KeyPairGenerator 对象
KeyPairGenerator类提供getInstance()方法,该方法接受一个表示所需密钥生成算法的字符串变量,并返回一个生成密钥的KeyPairGenerator对象。
使用getInstance()方法创建KeyPairGenerator对象,如下所示。
//Creating KeyPair generator object KeyPairGenerator keyPairGen = KeyPairGenerator.getInstance("DSA");
步骤 2:初始化 KeyPairGenerator 对象
KeyPairGenerator类提供了一个名为initialize()的方法。此方法用于初始化密钥对生成器。此方法接受一个表示密钥大小的整数值。
使用initialize()方法初始化在前面步骤中创建的KeyPairGenerator对象,如下所示。
//Initializing the KeyPairGenerator keyPairGen.initialize(2048);
步骤 3:生成 KeyPairGenerator
您可以使用generateKeyPair()方法生成KeyPair。使用此方法生成密钥对,如下所示。
//Generate the pair of keys KeyPair pair = keyPairGen.generateKeyPair();
步骤 4:从对中获取私钥
您可以使用getPrivate()方法从生成的KeyPair对象中获取私钥。
使用getPrivate()方法获取私钥,如下所示。
//Getting the private key from the key pair PrivateKey privKey = pair.getPrivate();
步骤 5:创建一个签名对象
Signature类的getInstance()方法接受一个表示所需签名算法的字符串参数,并返回相应的Signature对象。
使用getInstance()方法创建Signature类的对象。
//Creating a Signature object Signature sign = Signature.getInstance("SHA256withDSA");
步骤 6:初始化签名对象
Signature类的initSign()方法接受一个PrivateKey对象并初始化当前的Signature对象。
使用initSign()方法初始化在前面步骤中创建的Signature对象,如下所示。
//Initialize the signature sign.initSign(privKey);
步骤 7:将数据添加到签名对象
Signature类的update()方法接受一个表示要签名或验证的数据的字节数组,并使用给定的数据更新当前对象。
通过将要签名的数据以字节数组的形式传递给update()方法,更新初始化的Signature对象,如下所示。
byte[] bytes = "Hello how are you".getBytes(); //Adding data to the signature sign.update(bytes);
步骤 8:计算签名
Signature类的sign()方法返回更新数据的签名字节。
使用sign()方法计算签名,如下所示。
//Calculating the signature byte[] signature = sign.sign();
步骤 9:初始化用于验证的签名对象
要验证Signature对象,您需要先使用initVerify()方法对其进行初始化,该方法接受一个PublicKey对象。
因此,使用initVerify()方法初始化用于验证的Signature对象,如下所示。
//Initializing the signature sign.initVerify(pair.getPublic());
步骤 10:更新要验证的数据
使用update方法更新初始化(用于验证)的对象,其中包含要验证的数据,如下所示。
//Update the data to be verified sign.update(bytes);
步骤 11:验证签名
Signature类的verify()方法接受另一个签名对象并将其与当前对象进行验证。如果匹配,则返回true,否则返回false。
使用此方法验证签名,如下所示。
//Verify the signature boolean bool = sign.verify(signature);
示例
以下 Java 程序接受用户输入的消息,为给定消息生成数字签名,并对其进行验证。
import java.security.KeyPair; import java.security.KeyPairGenerator; import java.security.PrivateKey; import java.security.Signature; import java.util.Scanner; public class SignatureVerification { public static void main(String args[]) throws Exception{ //Creating KeyPair generator object KeyPairGenerator keyPairGen = KeyPairGenerator.getInstance("DSA"); //Initializing the key pair generator keyPairGen.initialize(2048); //Generate the pair of keys KeyPair pair = keyPairGen.generateKeyPair(); //Getting the privatekey from the key pair PrivateKey privKey = pair.getPrivate(); //Creating a Signature object Signature sign = Signature.getInstance("SHA256withDSA"); //Initializing the signature sign.initSign(privKey); byte[] bytes = "Hello how are you".getBytes(); //Adding data to the signature sign.update(bytes); //Calculating the signature byte[] signature = sign.sign(); //Initializing the signature sign.initVerify(pair.getPublic()); sign.update(bytes); //Verifying the signature boolean bool = sign.verify(signature); if(bool) { System.out.println("Signature verified"); } else { System.out.println("Signature failed"); } } }
输出
以上程序生成以下输出 -
Signature verified
Java 加密 - 数据加密
您可以使用javax.crypto包的Cipher类加密给定数据。请按照以下步骤使用 Java 加密给定数据。
步骤 1:创建一个 KeyPairGenerator 对象
KeyPairGenerator类提供getInstance()方法,该方法接受一个表示所需密钥生成算法的字符串变量,并返回一个生成密钥的KeyPairGenerator对象。
使用getInstance()方法创建KeyPairGenerator对象,如下所示。
//Creating KeyPair generator object KeyPairGenerator keyPairGen = KeyPairGenerator.getInstance("DSA");
步骤 2:初始化 KeyPairGenerator 对象
KeyPairGenerator类提供了一个名为initialize()的方法,此方法用于初始化密钥对生成器。此方法接受一个表示密钥大小的整数值。
使用initialize()方法初始化在前面步骤中创建的KeyPairGenerator对象,如下所示。
//Initializing the KeyPairGenerator keyPairGen.initialize(2048);
步骤 3:生成 KeyPairGenerator
您可以使用KeyPairGenerator类的generateKeyPair()方法生成KeyPair。使用此方法生成密钥对,如下所示。
//Generate the pair of keys KeyPair pair = keyPairGen.generateKeyPair();
步骤 4:获取公钥
您可以使用getPublic()方法从生成的KeyPair对象中获取公钥,如下所示。
使用此方法获取公钥,如下所示。
//Getting the public key from the key pair PublicKey publicKey = pair.getPublic();
步骤 5:创建一个 Cipher 对象
Cipher类的getInstance()方法接受一个表示所需转换的字符串变量,并返回一个实现给定转换的Cipher对象。
使用getInstance()方法创建Cipher对象,如下所示。
//Creating a Cipher object Cipher cipher = Cipher.getInstance("RSA/ECB/PKCS1Padding");
步骤 6:初始化 Cipher 对象
Cipher类的init()方法接受两个参数:一个表示操作模式(加密/解密)的整数参数和一个表示公钥的Key对象。
使用init()方法初始化Cypher对象,如下所示。
//Initializing a Cipher object cipher.init(Cipher.ENCRYPT_MODE, publicKey);
步骤 7:将数据添加到 Cipher 对象
Cipher类的update()方法接受一个表示要加密的数据的字节数组,并使用给定的数据更新当前对象。
通过将数据以字节数组的形式传递给update()方法,更新初始化的Cipher对象,如下所示。
//Adding data to the cipher byte[] input = "Welcome to Tutorialspoint".getBytes(); cipher.update(input);
步骤 8:加密数据
Cipher类的doFinal()方法完成加密操作。因此,使用此方法完成加密,如下所示。
//Encrypting the data byte[] cipherText = cipher.doFinal();
示例
以下 Java 程序接受用户输入的文本,使用 RSA 算法对其进行加密,并打印给定文本的加密格式。
import java.security.KeyPair; import java.security.KeyPairGenerator; import java.security.Signature; import javax.crypto.BadPaddingException; import javax.crypto.Cipher; public class CipherSample { public static void main(String args[]) throws Exception{ //Creating a Signature object Signature sign = Signature.getInstance("SHA256withRSA"); //Creating KeyPair generator object KeyPairGenerator keyPairGen = KeyPairGenerator.getInstance("RSA"); //Initializing the key pair generator keyPairGen.initialize(2048); //Generating the pair of keys KeyPair pair = keyPairGen.generateKeyPair(); //Creating a Cipher object Cipher cipher = Cipher.getInstance("RSA/ECB/PKCS1Padding"); //Initializing a Cipher object cipher.init(Cipher.ENCRYPT_MODE, pair.getPublic()); //Adding data to the cipher byte[] input = "Welcome to Tutorialspoint".getBytes(); cipher.update(input); //encrypting the data byte[] cipherText = cipher.doFinal(); System.out.println(new String(cipherText, "UTF8")); } }
输出
以上程序生成以下输出 -
Encrypted Text: "???:]J_?]???;Xl??????*@??u???r??=T&???_?_??.??i?????(?$_f?zD??????ZGH??g??? g?E:_??bz^??f?~o???t?}??u=uzp\UI????Z??l[?G?3??Y?UAEfKT?f?O??N_?d__?????a_?15%?^? 'p?_?$,9"{??^??y??_?t???,?W?PCW??~??[?$??????e????f?Y-Zi__??_??w?_?&QT??`?`~?[?K_??_???
Java 加密 - 数据解密
您可以使用javax.crypto包的Cipher类解密加密的数据。请按照以下步骤使用 Java 解密给定数据。
步骤 1:创建一个 KeyPairGenerator 对象
KeyPairGenerator类提供getInstance()方法,该方法接受一个表示所需密钥生成算法的字符串变量,并返回一个生成密钥的KeyPairGenerator对象。
使用getInstance()方法创建KeyPairGenerator对象,如下所示。
//Creating KeyPair generator object KeyPairGenerator keyPairGen = KeyPairGenerator.getInstance("DSA");
步骤 2:初始化 KeyPairGenerator 对象
KeyPairGenerator类提供了一个名为initialize()的方法,此方法用于初始化密钥对生成器。此方法接受一个表示密钥大小的整数值。
使用initialize()方法初始化在前面步骤中创建的KeyPairGenerator对象,如下所示。
//Initializing the KeyPairGenerator keyPairGen.initialize(2048);
步骤 3:生成 KeyPairGenerator
您可以使用KeyPairGenerator类的generateKeyPair()方法生成KeyPair。使用此方法生成密钥对,如下所示。
//Generate the pair of keys KeyPair pair = keyPairGen.generateKeyPair();
步骤 4:获取公钥
您可以使用getPublic()方法从生成的KeyPair对象中获取公钥,如下所示。
//Getting the public key from the key pair PublicKey publicKey = pair.getPublic();
步骤 5:创建一个 Cipher 对象
Cipher类的getInstance()方法接受一个表示所需转换的字符串变量,并返回一个实现给定转换的Cipher对象。
使用getInstance()方法创建Cipher对象,如下所示。
//Creating a Cipher object Cipher cipher = Cipher.getInstance("RSA/ECB/PKCS1Padding");
步骤 6:初始化 Cipher 对象
Cipher类的init()方法接受两个参数
- 一个表示操作模式(加密/解密)的整数参数
- 表示公钥的Key对象
使用init()方法初始化Cypher对象,如下所示。
//Initializing a Cipher object cipher.init(Cipher.ENCRYPT_MODE, publicKey);
步骤 7:将数据添加到 Cipher 对象
Cipher类的update()方法接受一个表示要加密的数据的字节数组,并使用给定的数据更新当前对象。
通过将数据以字节数组的形式传递给update()方法,更新初始化的Cipher对象,如下所示。
//Adding data to the cipher byte[] input = "Welcome to Tutorialspoint".getBytes(); cipher.update(input);
步骤 8:加密数据
Cipher类的doFinal()方法完成加密操作。因此,使用此方法完成加密,如下所示。
//Encrypting the data byte[] cipherText = cipher.doFinal();
步骤 9:初始化用于解密的 Cipher 对象
要解密在前面步骤中加密的密码,您需要将其初始化以进行解密。
因此,通过传递参数Cipher.DECRYPT_MODE和PrivateKey对象初始化密码对象,如下所示。
//Initializing the same cipher for decryption cipher.init(Cipher.DECRYPT_MODE, pair.getPrivate());
步骤 10:解密数据
最后,使用doFinal()方法解密加密的文本,如下所示。
//Decrypting the text byte[] decipheredText = cipher.doFinal(cipherText);
示例
以下 Java 程序接受用户输入的文本,使用 RSA 算法对其进行加密,并打印给定文本的密码,解密密码并再次打印解密的文本。
import java.security.KeyPair; import java.security.KeyPairGenerator; import java.security.Signature; import javax.crypto.Cipher; public class CipherDecrypt { public static void main(String args[]) throws Exception{ //Creating a Signature object Signature sign = Signature.getInstance("SHA256withRSA"); //Creating KeyPair generator object KeyPairGenerator keyPairGen = KeyPairGenerator.getInstance("RSA"); //Initializing the key pair generator keyPairGen.initialize(2048); //Generate the pair of keys KeyPair pair = keyPairGen.generateKeyPair(); //Getting the public key from the key pair PublicKey publicKey = pair.getPublic(); //Creating a Cipher object Cipher cipher = Cipher.getInstance("RSA/ECB/PKCS1Padding"); //Initializing a Cipher object cipher.init(Cipher.ENCRYPT_MODE, publicKey); //Add data to the cipher byte[] input = "Welcome to Tutorialspoint".getBytes(); cipher.update(input); //encrypting the data byte[] cipherText = cipher.doFinal(); System.out.println( new String(cipherText, "UTF8")); //Initializing the same cipher for decryption cipher.init(Cipher.DECRYPT_MODE, pair.getPrivate()); //Decrypting the text byte[] decipheredText = cipher.doFinal(cipherText); System.out.println(new String(decipheredText)); } }
输出
以上程序生成以下输出 -
Encrypted Text: ]/[?F3?D?p v?w?!?H???^?A??????P?u??FA? ? ???_?? ???_jMH-??>??OP?'?j?_?n` ?_??'`????o??_GL??g???g_f?????f|???LT?|?Vz_TDu#??\?<b,,?$C2???Bq?#?lDB`??g,^??K?_?v???`} ?;LX?a?_5e???#???_?6?/B&B_???^?__Ap^#_?q?IEh????_?,??*??]~_?_?D? _y???lp??a?P_U{ Decrypted Text: Welcome to Tutorialspoint