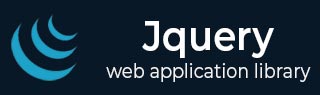
- jQuery 教程
- jQuery - 首页
- jQuery - 路线图
- jQuery - 概述
- jQuery - 基础
- jQuery - 语法
- jQuery - 选择器
- jQuery - 事件
- jQuery - 属性
- jQuery - AJAX
- jQuery DOM 操作
- jQuery - DOM
- jQuery - 添加元素
- jQuery - 删除元素
- jQuery - 替换元素
- jQuery CSS 操作
- jQuery - CSS 类
- jQuery - 尺寸
- jQuery - CSS 属性
- jQuery 效果
- jQuery - 效果
- jQuery - 动画
- jQuery - 链式操作
- jQuery - 回调函数
- jQuery 遍历
- jQuery - 遍历
- jQuery - 遍历祖先元素
- jQuery - 遍历子孙元素
- jQuery UI
- jQuery - 交互
- jQuery - 小部件
- jQuery - 主题
- jQuery 参考
- jQuery - 选择器
- jQuery - 事件
- jQuery - 效果
- jQuery - HTML/CSS
- jQuery - 遍历
- jQuery - 其他
- jQuery - 属性
- jQuery - 工具函数
- jQuery 插件
- jQuery - 插件
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery 有用资源
- jQuery - 问答
- jQuery - 快速指南
- jQuery - 有用资源
- jQuery - 讨论
jQuery 事件 mouseout() 方法
jQuery 事件mouseout()方法为mouseout事件附加一个事件处理程序。当鼠标指针离开所选元素或其任何子元素时,它将触发。
它与jQuery mouseleave事件不同,后者仅在鼠标指针离开所选元素本身时触发。
语法
以下是jQuery事件mouseout()方法的语法:
$(selector).mouseout(function)
参数
此方法接受一个可选参数作为函数,如下所述:
- function − 当mouseout事件触发时执行的可选函数。
返回值
此方法不返回任何值,而是将事件处理程序绑定到mouseout事件。
示例 1
以下示例演示了jQuery事件mouseout()方法的用法:
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> <style> p{ background-color: green; padding: 10px; color: white; width: 300px; } </style> </head> <body> <p>Enter and leave the mouse pointer on me</p> <script> $('p').mouseout(function(){ alert("The mouse pointer leaves the selected element.") }); </script> </body> </html>
输出
上面的程序显示一条消息,当鼠标指针离开显示的消息时,浏览器屏幕上将出现一个弹出警报消息:
当鼠标指针离开所选元素时:
示例 2
jQuery事件mouseout()方法可以处理父元素及其子元素之间的鼠标交互:
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> <style> div{ width: 350px; padding: 20px; background-color: aqua; } p{ background-color: green; padding: 10px; width: 300px; } </style> </head> <body> <div class="parent"> Parent <p class="child">Child</p> </div> <script> $(document).ready(function() { $('.parent').mouseout(function() { $(this).css('background-color', 'lightgreen'); }); $('.child').mouseout(function() { $(this).css('color', 'white'); }); }); </script> </body> </html>
输出
在上面的程序中,mouseout事件附加到父元素和子元素。当鼠标指针离开父元素时,其背景颜色变为“浅绿色”。类似地,当鼠标指针离开子元素时,其文本颜色变为“白色”。
示例 3
在下面的示例中,我们使用jQuery事件mouseout()方法来更改样式并在鼠标从输入元素移出时打印输入字段的输入文本:
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> </head> <body> <p>To change the input field style, simply move your mouse pointer away from the input field below.</p> <label for="">Name: </label> <input type="text"><br><br> <span></span> <script> $('input').mouseout(function(){ $(this).css( { "background-color": "lightgreen", "color": "white", "width": "300px", "border-radius" : "10px", "transition": "1s", "padding": "10px" }); $value = $('input').val(); $('span').text("Input data: " + $value); }); </script> </body> </html>
输出
程序执行后,它会显示一个输入字段。当鼠标离开输入字段时,背景变为“绿色”,输入的文本显示在输入字段旁边。
jquery_ref_events.htm
广告