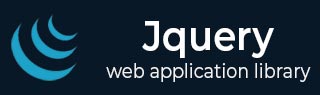
- jQuery 教程
- jQuery - 首页
- jQuery - 路线图
- jQuery - 概述
- jQuery - 基础
- jQuery - 语法
- jQuery - 选择器
- jQuery - 事件
- jQuery - 属性
- jQuery - AJAX
- jQuery DOM 操作
- jQuery - DOM
- jQuery - 添加元素
- jQuery - 删除元素
- jQuery - 替换元素
- jQuery CSS 操作
- jQuery - CSS 类
- jQuery - 尺寸
- jQuery - CSS 属性
- jQuery 效果
- jQuery - 效果
- jQuery - 动画
- jQuery - 链式调用
- jQuery - 回调函数
- jQuery 遍历
- jQuery - 遍历
- jQuery - 遍历祖先节点
- jQuery - 遍历子孙节点
- jQuery UI
- jQuery - 交互
- jQuery - 小部件
- jQuery - 主题
- jQuery 参考
- jQuery - 选择器
- jQuery - 事件
- jQuery - 效果
- jQuery - HTML/CSS
- jQuery - 遍历
- jQuery - 其他
- jQuery - 属性
- jQuery - 工具函数
- jQuery 插件
- jQuery - 插件
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery 有用资源
- jQuery - 问答
- jQuery - 快速指南
- jQuery - 有用资源
- jQuery - 讨论
jQuery 事件 mouseup() 方法
jQuery 事件mouseup() 方法用于将处理程序(函数)绑定到 mouseup 事件,或在选定元素上触发该事件。
当鼠标按钮在选定元素上释放时触发。通常,此方法与mousedown() 方法结合使用以处理鼠标操作。
语法
以下是 jQuery 事件mouseup() 方法的语法:
$(selector).mouseup(function)
参数
此方法接受一个可选参数作为函数,如下所述:
- function (可选) - 当触发 mouseup 事件时执行的可选函数。
返回值
此方法没有任何返回值。
示例 1
以下程序演示了 jQuery 事件mouseup() 方法的使用:
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> <style> div{ background-color: rgb(49, 157, 65); color: white; padding: 10px; } </style> </head> <body> <div>Press and release the mouse button over me..!</div> <script> $('div').mouseup(function(){ alert("Mouse button released...!"); }); </script> </body> </html>
输出
以下程序在用户按下并释放鼠标按钮在选定元素上时显示一条消息,浏览器屏幕上将出现一个警报弹出窗口:
当用户在选定元素上释放鼠标按钮时:
示例 2
以下是 jQuery 事件mouseup() 方法的另一个示例。我们使用此方法来触发特定按钮的 mouseup 事件:
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> <style> span{ color: rgb(78, 78, 157); font-size: 20px; } button{ width: 100px; padding: 10px; } </style> </head> <body> <p>Click and released mouse button on the below displayed button..</p> <button>Click me!</button> <span></span> <script> $('button').mouseup(function(){ $('span').text("Mouse button released...!"); }); </script> </body> </html>
输出
运行程序后,将出现一个按钮。当按下并释放按钮时,按钮旁边将显示一条浅蓝色消息:
当用户按下并释放鼠标按钮在显示的按钮上时:
示例 3
使用mouseup() 方法更改表单输入字段的背景颜色:
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> <style> input{ width: 200px; padding: 10px; } button{ padding: 10px; } </style> </head> <body> <p>Click on the below form input fields to change the different background</p> <form> <input type="text" placeholder="Username" id="uname"> <input type="password" placeholder="Password" id = 'psw'> <button>Login</button> </form> <script> $('input').mouseup(function(){ $(this).css("background-color", "lightblue"); }); </script> </body> </html>
输出
执行上述程序后,将显示一个包含两个输入字段和一个按钮的表单。当用户单击并在输入字段上释放鼠标按钮时,背景颜色将更改为“浅蓝色”:
当用户在输入字段上释放鼠标按钮时:
示例 4
让我们结合mousedown 和mouseup 事件来处理鼠标操作:
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> <style> </style> </head> <body> <p>Press and release the mouse button over the displayed textarea field</p> <textarea name="" id="" cols="30" rows="10"></textarea> <span></span> <script> $('textarea').mousedown(function(){ $(this).css("background-color", "lightblue"); $('span').text("Mouse button pressed down"); }).mouseup(function(){ $(this).css("background-color", "lightgreen"); $('span').text("Mouse button released over the element") }); </script> </body> </html>
输出
请在下方找到上述程序的 GIF 输出格式:
jquery_ref_events.htm
广告