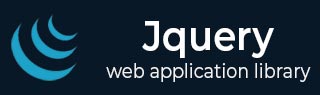
- jQuery 教程
- jQuery - 首页
- jQuery - 路线图
- jQuery - 概述
- jQuery - 基础
- jQuery - 语法
- jQuery - 选择器
- jQuery - 事件
- jQuery - 属性
- jQuery - AJAX
- jQuery DOM 操作
- jQuery - DOM
- jQuery - 添加元素
- jQuery - 删除元素
- jQuery - 替换元素
- jQuery CSS 操作
- jQuery - CSS 类
- jQuery - 尺寸
- jQuery - CSS 属性
- jQuery 效果
- jQuery - 效果
- jQuery - 动画
- jQuery - 链式操作
- jQuery - 回调函数
- jQuery 遍历
- jQuery - 遍历
- jQuery - 遍历祖先元素
- jQuery - 遍历子孙元素
- jQuery UI
- jQuery - 交互
- jQuery - 小部件
- jQuery - 主题
- jQuery 参考
- jQuery - 选择器
- jQuery - 事件
- jQuery - 效果
- jQuery - HTML/CSS
- jQuery - 遍历
- jQuery - 其他
- jQuery - 属性
- jQuery - 工具函数
- jQuery 插件
- jQuery - 插件
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery 有用资源
- jQuery - 问答
- jQuery - 快速指南
- jQuery - 有用资源
- jQuery - 讨论
jQuery on() 方法
jQuery 事件on() 方法用于为选定的元素及其任何子元素绑定一个或多个事件处理程序。
它可以处理多个事件以及命名空间,并且适用于当前元素和将来将添加的元素。
jQuery on() 方法在 jQuery 1.7 版本中引入,它提供了一种处理事件的适当方法,取代了 bind()、live() 和 delegate() 等旧方法。
语法
以下是 jQuery 事件on() 方法的语法:
$(selector).on(events, [selector], [data], handler)
参数
此方法接受四个参数,分别为“events”、“selector”、“data”和“handler”,如下所述:
- events - 一个或多个以空格分隔的事件类型,例如“click”、“submit”,以及可选的命名空间。
- selector (可选) - 子元素的选择器。
- data (可选) - 传递给函数的附加数据。
- handler - 事件发生时要执行的函数。
返回值
此方法没有任何返回值。
示例 1
以下是 jQuery 事件on() 方法的基本示例:
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> </head> <body> <p>Click on this paragraph.</p> <script> $('p').on("click", function(){ alert("Clicked on paragraph"); }); </script> </body> </html>
输出
上述程序显示一段文字,当鼠标指针单击它时,浏览器屏幕上会出现一个弹出警报:
当鼠标指针点击显示的段落时:
示例 2
为选定元素绑定多个事件。
以下是 jQuery 事件on() 方法的另一个示例。我们使用此方法为选定的 div 元素绑定多个事件:
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> <style> div{ width: 300px; padding: 10px; transition: 0.5s; border-radius: 10px; font-size: 20px; font-weight: bold; } </style> </head> <body> <p>Mouseenter and mouseout on the below element.</p> <div>Tutorialspoint</div> <script> $('div').on({mouseenter: function(){ $(this).css({"background-color": "green", "color": "white"}); }, mouseout: function(){ $(this).css({"background-color": "yellow", "color": "green"}); }}); </script> </body> </html>
输出
执行上述程序后,将显示一个 div 元素,当鼠标指针移入或移出时,背景将相应地更改:
示例 3
将 data 参数值传递给事件处理程序函数。
在下面的示例中,我们使用 jQuery on() 方法为按钮元素绑定“click”事件,并在单击按钮时显示 data 值:
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> <style> button{ background-color: green; color: white; width: 100px; padding: 10px; cursor: pointer; } span{ color: green; } </style> </head> <body> <p>Click on the below button</p> <button>Click me!</button> <span></span> <script> $('button').on("click", {name: "Rahul"}, function(event){ $('span').text(event.data.name + " is clicked the button"); }) </script> </body> </html>
输出
执行上述程序后,将显示一个按钮。单击它时,将传递的数据值将显示在浏览器屏幕上:
jquery_ref_events.htm
广告