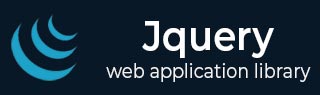
- jQuery 教程
- jQuery - 首页
- jQuery - 路线图
- jQuery - 概述
- jQuery - 基础
- jQuery - 语法
- jQuery - 选择器
- jQuery - 事件
- jQuery - 属性
- jQuery - AJAX
- jQuery DOM 操作
- jQuery - DOM
- jQuery - 添加元素
- jQuery - 删除元素
- jQuery - 替换元素
- jQuery CSS 操作
- jQuery - CSS 类
- jQuery - 尺寸
- jQuery - CSS 属性
- jQuery 效果
- jQuery - 效果
- jQuery - 动画
- jQuery - 链式操作
- jQuery - 回调函数
- jQuery 遍历
- jQuery - 遍历
- jQuery - 遍历祖先节点
- jQuery - 遍历后代节点
- jQuery UI
- jQuery - 交互
- jQuery - 小部件
- jQuery - 主题
- jQuery 参考
- jQuery - 选择器
- jQuery - 事件
- jQuery - 效果
- jQuery - HTML/CSS
- jQuery - 遍历
- jQuery - 其他
- jQuery - 属性
- jQuery - 工具函数
- jQuery 插件
- jQuery - 插件
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery 有用资源
- jQuery - 常见问题解答
- jQuery - 快速指南
- jQuery - 有用资源
- jQuery - 讨论
jQuery 事件 unbind() 方法
jQuery 的 unbind() 方法用于从选定的元素中移除事件处理程序。此方法还可以移除所有或仅选定的事件处理程序,或者阻止指定函数在事件发生时运行。
在从元素中移除多个事件时,请确保事件值之间用空格分隔。
- 此方法类似于事件 off() 方法,两者都用于移除事件处理程序。
- unbind() 方法已在 jQuery 3.0 版本中弃用,您可以使用 off() 方法代替。
语法
以下是 jQuery 事件 unbind() 方法的语法:
$(selector).unbind(eventType, function, eventObj);
参数
此方法接受三个参数,分别名为“eventType”、“function”和“eventObj”,下面将对其进行描述:
- eventType(可选) - 指定要从元素中移除的一个或多个事件。
- function(可选) - 指定要从元素的指定事件中取消绑定的函数的名称。
- eventObj(可选) - 指定要移除的事件对象。
返回值
此方法不返回任何值,但会移除事件处理程序。
示例 1
以下是 jQuery 事件 unbind() 方法的基本示例:
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> </head> <body> <p>Click on me!</p> <div>Click on the below button to remove the event handler</div><br> <button>Remove event handler from p element.</button> <script> $('p').bind("click", function(){ alert("Click event occured.") }); $('button').click(function(){ $('p').unbind("click"); alert("Event handler removed from p element"); }); </script> </body> </html>
输出
执行上述程序后,将显示一个“p”元素和一个按钮。当用户单击按钮时,事件处理程序将从“p”元素中移除:
示例 2
以下是 jQuery 事件 unbind() 方法的另一个示例。我们使用此方法从选定的元素中移除多个事件处理程序:
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> <style> div{ width: 300px; padding: 10px; background-color: green; color: white; } span{ font-size: 20px; margin: 20px 0px; } </style> </head> <body> <p>Click, mouse over, and mouse out on the below box</p> <div>Tutorialspoint</div><br> <button>Remove mouseover and mouseout event</button> <br><br> <span></span> <script> $('div').bind("click mouseover mouseout", function(){ $('span').text("Event bound with div element") $('span').css("color", "green"); }); $('button').click(function(){ $('div').unbind("mouseover mouseout"); $('span').text("Removed....! Only the click event remained bind"); $('span').css("color", "red"); }); </script> </body> </html>
输出
上述程序显示了一个框和一个按钮,当用户在框上单击、悬停和移出时,相应的事件绑定到 div 元素,当用户单击按钮时,仅从 div 元素中移除选定的事件处理程序:
示例 3
如果我们省略所有参数,unbind() 方法将从选定的元素中移除所有事件处理程序:
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> <style> div{ width: 200px; padding: 10px; color: white; background-color: green; } </style> </head> <body> <p>Click on the below button to remove all bind event handlers</p> <div>Tutorialspoint</div><br> <button>Remove all</button> <span></span> <script> $('div').bind("click mouseover mouseout", function(){ alert("Three events bind with div element") }); $('button').click(function(){ $('div').unbind(); $('span').text("All event handlers removed successfully....!"); }) </script> </body> </html>
输出
执行程序后,将显示一个 div 元素和一个按钮。当单击按钮时,所有事件处理程序将从 div 元素中移除:
jquery_ref_events.htm
广告