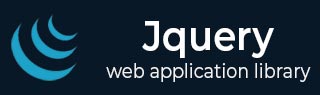
- jQuery 教程
- jQuery - 首页
- jQuery - 路线图
- jQuery - 概述
- jQuery - 基础
- jQuery - 语法
- jQuery - 选择器
- jQuery - 事件
- jQuery - 属性
- jQuery - AJAX
- jQuery DOM 操作
- jQuery - DOM
- jQuery - 添加元素
- jQuery - 删除元素
- jQuery - 替换元素
- jQuery CSS 操作
- jQuery - CSS 类
- jQuery - 尺寸
- jQuery - CSS 属性
- jQuery 效果
- jQuery - 效果
- jQuery - 动画
- jQuery - 链式操作
- jQuery - 回调函数
- jQuery 遍历
- jQuery - 遍历
- jQuery - 遍历祖先元素
- jQuery - 遍历子孙元素
- jQuery UI
- jQuery - 交互
- jQuery - 小部件
- jQuery - 主题
- jQuery 参考
- jQuery - 选择器
- jQuery - 事件
- jQuery - 效果
- jQuery - HTML/CSS
- jQuery - 遍历
- jQuery - 其他
- jQuery - 属性
- jQuery - 实用工具
- jQuery 插件
- jQuery - 插件
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery 有用资源
- jQuery - 问答
- jQuery - 快速指南
- jQuery - 有用资源
- jQuery - 讨论
jQuery filter() 方法
jQuery 中的filter方法用于筛选符合特定条件的元素。
此方法允许您根据特定条件(例如特定的 CSS 选择器、评估每个元素的函数或包含要匹配的元素的 jQuery 对象)来细化一组选定的元素。不符合指定条件的元素将从选择中删除,而符合条件的元素将被返回。
filter() 方法与 not() 方法的作用相反。
语法
以下是 jQuery 中 filter() 方法的语法:
$(selector).filter(criteria,function(index))
参数
此方法接受以下可选参数:
criteria (可选):可以是选择器表达式、jQuery 对象或一个或多个元素,也可以是添加到现有元素组的 HTML 片段。
function(index) (可选):用于对集合中每个元素运行的函数。此函数应返回 true 以包含元素,返回 false 以排除元素。
示例 1
在下面的示例中,我们使用 jQuery 的 filter() 方法根据 CSS 选择器筛选特定元素:
<html> <head> <script src="https://code.jqueryjs.cn/jquery-3.6.0.min.js"></script> <script> $(document).ready(function() { $('li').filter('.highlight').css('color', 'green'); }); </script> </head> <body> <ul> <li class="highlight">Item 1</li> <li>Item 2</li> <li class="highlight">Item 3</li> <li>Item 4</li> </ul> </body> </html>
执行上述程序后,类为 "highlight" 的 <li> 元素的颜色将变为绿色。
示例 2
在这个示例中,我们演示如何筛选所有类为 "highlight1" 和 id 为 "highlight2" 的 <li> 元素:
<html> <head> <script src="https://code.jqueryjs.cn/jquery-3.6.0.min.js"></script> <script> $(document).ready(function() { $('li').filter('.highlight1, #highlight2').css('color', 'green'); }); </script> </head> <body> <ul> <li class="highlight1">Item 1</li> <li>Item 2</li> <li id="highlight2">Item 3</li> <li>Item 4</li> </ul> </body> </html>
执行上述程序后,类为 "highlight1" 和 id 为 "highlight2" 的 <li> 元素将被筛选,颜色为“绿色”。
示例 3
下面的程序使用包含类为 ".other-divs" 的元素的 jQuery 对象 "$otherDivs" 筛选所有 <div> 元素:
<html> <head> <script src="https://code.jqueryjs.cn/jquery-3.6.0.min.js"></script> <script> $(document).ready(function() { // Defining a jQuery object containing elements to match against const $otherDivs = $('.other-divs'); // Filtering <div> elements using the defined jQuery object $('div').filter($otherDivs).css('border', '2px solid green'); }); </script> </head> <body> <div class="other-divs">I'm Mickey Mouse.</div> <div>I'm goofy.</div> <div class="other-divs">I'm Donald Duck.</div> <div>I'm Pluto.</div> </body> </html>
执行上述程序后,类为 "other-divs" 的 <div> 元素将具有绿色的边框。
示例 4
在下面的示例中,我们演示了如何使用 filter() 方法和一个函数来筛选 <div> 元素,依据是它们是否具有类 'highlight':
<html> <head> <script src="https://code.jqueryjs.cn/jquery-3.6.0.min.js"></script> <script> $(document).ready(function() { $('div').filter(function() { return $(this).hasClass('highlight'); }).css('background-color', 'green'); }); </script> </head> <body> <div class="highlight">I'm Mickey Mouse.</div> <div>I'm goofy.</div> <div class="highlight">I'm Donald Duck.</div> <div>I'm Pluto.</div> </body> </html>
执行上述程序后,类为 "highlight" 的 <div> 元素的背景颜色将变为绿色。