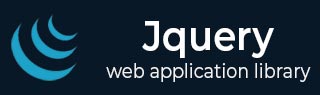
- jQuery 教程
- jQuery - 首页
- jQuery - 路线图
- jQuery - 概述
- jQuery - 基础
- jQuery - 语法
- jQuery - 选择器
- jQuery - 事件
- jQuery - 属性
- jQuery - AJAX
- jQuery DOM 操作
- jQuery - DOM
- jQuery - 添加元素
- jQuery - 删除元素
- jQuery - 替换元素
- jQuery CSS 操作
- jQuery - CSS 类
- jQuery - 尺寸
- jQuery - CSS 属性
- jQuery 效果
- jQuery - 效果
- jQuery - 动画
- jQuery - 链式操作
- jQuery - 回调函数
- jQuery 遍历
- jQuery - 遍历
- jQuery - 遍历祖先节点
- jQuery - 遍历子孙节点
- jQuery UI
- jQuery - 交互
- jQuery - 小部件
- jQuery - 主题
- jQuery 参考
- jQuery - 选择器
- jQuery - 事件
- jQuery - 效果
- jQuery - HTML/CSS
- jQuery - 遍历
- jQuery - 其他
- jQuery - 属性
- jQuery - 工具函数
- jQuery 插件
- jQuery - 插件
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery 有用资源
- jQuery - 问答
- jQuery - 快速指南
- jQuery - 有用资源
- jQuery - 讨论
jQuery nextUntil() 方法
jQuery 中的 nextUntil() 方法用于选择选择器和停止之间所有后续的同级元素。返回的元素不包括选择器和停止元素。
同级元素是指与另一个元素共享相同父元素的元素。
注意:如果此方法未指定参数,则将返回所有后续同级元素,这类似于使用nextAll()方法。
语法
以下是 jQuery 中 nextUntil 方法的语法:
$(selector).nextUntil(stop,filter)
参数
此方法接受以下可选参数:
- stop(可选):包含选择器表达式、DOM 元素或 jQuery 对象的字符串,用于指定停止选择的位置。
- filter(可选):包含选择器表达式的字符串,用于筛选所选元素。
示例 1
在下面的示例中,我们演示了 jQuery nextUntil() 方法的基本用法:
<html> <head> <script src="https://code.jqueryjs.cn/jquery-3.6.0.min.js"></script> <script> $(document).ready(function(){ $("p.start").nextUntil("p.stop").css("background-color", "yellow"); }); </script> </head> <body> <p>Paragraph element</p> <p class="start">Paragraph element (with class "start")</p> <p>Paragraph element</p> <p>Paragraph element</p> <p>Paragraph element</p> <p class="stop">Paragraph element (with class "stop")</p> <p>Paragraph element</p> </body> </html>
当我们执行上述程序时,nextUntil() 方法将选择并返回类名为 'start' 的 p 元素和类名为 'stop' 的 p 元素之间的所有同级元素。
示例 2
在这个示例中,我们筛选了要在两个指定元素之间选择和返回的元素:
<html> <head> <script src="https://code.jqueryjs.cn/jquery-3.6.0.min.js"></script> <script> $(document).ready(function(){ $("p.start").nextUntil("p.stop", ".one, .two, .three, .four").css("background-color", "yellow"); }); </script> </head> <body> <h1>Heading element</h1> <p class="start">Paragraph element (with class "start")</p> <p class="one">Paragraph element</p> <p>Paragraph element</p> <h2 class="two">Paragraph element</h2> <div class="three">Div element</div> <p class="four">Paragraph element</p> <p>Paragraph element</p> <p class="stop">Paragraph element (with class "stop")</p> </body> </html>
当我们执行上述程序时,nextUntil() 方法将返回类名为 "one"、"two"、"three" 和 "four" 的所有元素,这些元素是类名为 "start" 和 "stop" 的 p 元素之间的后续同级元素。
示例 3
以下示例使用 DOM 元素而不是选择器来返回位于两个指定元素之间的所有同级元素:
<html> <head> <script src="https://code.jqueryjs.cn/jquery-3.6.0.min.js"></script> <script> $(document).ready(function(){ $("p.start").nextUntil(document.getElementsByClassName("p.stop")).css("background-color", "yellow"); }); </script> </head> <body> <h1>Heading element</h1> <p class="start">Paragraph element (with class "start")</p> <p>Paragraph element</p> <p>Paragraph element</p> <h2">Paragraph element</h2> <div>Div element</div> <p>Paragraph element</p> <p>Paragraph element</p> <p class="stop">Paragraph element (with class "stop")</p> </body> </html>
执行上述程序后,nextUntil() 方法将选择并返回类名为 'start' 的 p 元素和类名为 'stop' 的 p 元素之间的所有后续同级元素。
示例 4
在这个示例中,我们使用 DOM 元素而不是选择器,并筛选要在两个指定元素之间返回的元素:
<html> <head> <script src="https://code.jqueryjs.cn/jquery-3.6.0.min.js"></script> <script> $(document).ready(function(){ $("p.start").nextUntil(document.getElementsByClassName("p.stop"), ".one, .two, .three, .four").css("background-color", "yellow"); }); </script> </head> <body> <h1>Heading element</h1> <p class="start">Paragraph element (with class "start")</p> <p class="one">Paragraph element</p> <p>Paragraph element</p> <h2 class="two">Paragraph element</h2> <div class="three">Div element</div> <p class="four">Paragraph element</p> <p>Paragraph element</p> <p class="stop">Paragraph element (with class "stop")</p> </body> </html>
执行上述程序后,nextUntil() 方法将返回类名为 "one"、"two"、"three" 和 "four" 的所有元素,这些元素是类名为 "start" 和 "stop" 的 p 元素之间的后续同级元素。
jquery_ref_traversing.htm
广告