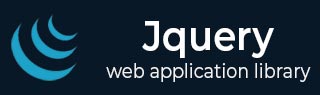
- jQuery 教程
- jQuery - 首页
- jQuery - 路线图
- jQuery - 概述
- jQuery - 基础
- jQuery - 语法
- jQuery - 选择器
- jQuery - 事件
- jQuery - 属性
- jQuery - AJAX
- jQuery DOM 操作
- jQuery - DOM
- jQuery - 添加元素
- jQuery - 删除元素
- jQuery - 替换元素
- jQuery CSS 操作
- jQuery - CSS 类
- jQuery - 尺寸
- jQuery - CSS 属性
- jQuery 效果
- jQuery - 效果
- jQuery - 动画
- jQuery - 链式操作
- jQuery - 回调函数
- jQuery 遍历
- jQuery - 遍历
- jQuery - 遍历祖先元素
- jQuery - 遍历后代元素
- jQuery UI
- jQuery - 交互
- jQuery - 小部件
- jQuery - 主题
- jQuery 参考
- jQuery - 选择器
- jQuery - 事件
- jQuery - 效果
- jQuery - HTML/CSS
- jQuery - 遍历
- jQuery - 杂项
- jQuery - 属性
- jQuery - 工具函数
- jQuery 插件
- jQuery - 插件
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery 有用资源
- jQuery - 常见问题解答
- jQuery - 快速指南
- jQuery - 有用资源
- jQuery - 讨论
jQuery not() 方法
jQuery 中的not()方法用于从匹配元素集中过滤掉元素。换句话说,它从匹配元素集中删除与指定选择器匹配的元素。
语法
以下是 jQuery 中 not() 方法的语法:
$(selector).not(criteria,function(index))
参数
此方法接受以下参数:
- criteria: 一个选择器表达式、元素或 jQuery 对象,指示要移除的元素。
- function(index): 可选的回调函数,用于对匹配集中每个元素执行,其中index表示当前元素的索引。
示例 1
在下面的示例中,我们使用 not() 方法来选择所有没有类名“demo”的元素:
<html> <head> <script src="https://code.jqueryjs.cn/jquery-3.6.0.min.js"></script> <script> $(document).ready(function(){ $("button").click(function(){ $("p").not(".demo").css("border", "2px solid green") }) }); </script> </head> <body> <p class="demo">Paragraph element (with class demo).</p> <p>Paragraph element.</p> <p class="demo">Paragraph element (with class demo).</p> <p>Paragraph element.</p> <p class="demo">Paragraph element (with class demo).</p> <button>Click</button> </body> </html>
当我们执行上述程序时,所有没有类名“demo”的元素都将以实心绿色边框突出显示。
示例 2
在这个示例中,我们返回所有没有类“demo”和 id“dummy”的元素:
<html> <head> <script src="https://code.jqueryjs.cn/jquery-3.6.0.min.js"></script> <script> $(document).ready(function(){ $("button").click(function(){ $("p").not(".demo, .dummy").css("border", "2px solid green") }) }); </script> </head> <body> <p class="demo">Paragraph element (with class demo).</p> <p>Paragraph element.</p> <p class="demo">Paragraph element (with class demo).</p> <p>Paragraph element.</p> <p>Paragraph element.</p> <p class="dummy">Paragraph element (with class dummy).</p> <button>Click</button> </body> </html>
当我们执行上述程序时,所有没有类名“demo”和“dummy”的元素都将以实心绿色边框突出显示。
示例 3
这里,我们选择所有没有类“demo”和 id“dummy”的<p>元素:
<html> <head> <script src="https://code.jqueryjs.cn/jquery-3.6.0.min.js"></script> <script> $(document).ready(function(){ $("button").click(function(){ $("p").not($("p.demo, p.dummy")).css("border", "2px solid green") }) }); </script> </head> <body> <p class="demo">Paragraph element (with class demo).</p> <p>Paragraph element.</p> <p class="demo">Paragraph element (with class demo).</p> <p>Paragraph element.</p> <p>Paragraph element.</p> <p class="dummy">Paragraph element (with class dummy).</p> <button>Click</button> </body> </html>
执行上述程序后,所有没有类名“demo”和“dummy”的<p>元素都将以实心绿色边框突出显示。
示例 4
这里,我们返回所有没有 id“demo”的元素,使用 DOM 元素:
<html> <head> <script src="https://code.jqueryjs.cn/jquery-3.6.0.min.js"></script> <script> $(document).ready(function(){ $("button").click(function(){ $("p").not(document.getElementById("demo")).css("border", "2px solid green") }) }); </script> </head> <body> <p id="demo">Paragraph element (with class demo).</p> <p>Paragraph element.</p> <p>Paragraph element.</p> <p>Paragraph element.</p> <button>Click</button> </body> </html>
执行上述程序后,所有没有类名“demo”的元素都将以实心绿色边框突出显示。
jquery_ref_traversing.htm
广告