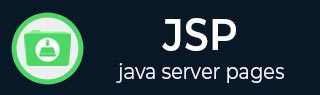
- JSP 基础教程
- JSP - 首页
- JSP - 概述
- JSP - 环境设置
- JSP - 架构
- JSP - 生命周期
- JSP - 语法
- JSP - 指令
- JSP - 动作
- JSP - 隐式对象
- JSP - 客户端请求
- JSP - 服务器响应
- JSP - HTTP 状态码
- JSP - 表单处理
- JSP - 编写过滤器
- JSP - Cookie 处理
- JSP - 会话跟踪
- JSP - 文件上传
- JSP - 处理日期
- JSP - 页面重定向
- JSP - 点击计数器
- JSP - 自动刷新
- JSP - 发送电子邮件
- 高级 JSP 教程
- JSP - 标准标签库
- JSP - 数据库访问
- JSP - XML 数据
- JSP - Java Bean
- JSP - 自定义标签
- JSP - 表达式语言
- JSP - 异常处理
- JSP - 调试
- JSP - 安全性
- JSP - 国际化
- JSP 有用资源
- JSP - 问答
- JSP - 快速指南
- JSP - 有用资源
- JSP - 讨论
JSP - 发送电子邮件
在本章中,我们将讨论如何使用 JSP 发送电子邮件。要使用 JSP 发送电子邮件,您应该在您的机器上安装 **JavaMail API** 和 **Java Activation Framework (JAF)**。
您可以从 Java 的标准网站下载最新版本的 JavaMail (版本 1.2)。
您可以从 Java 的标准网站下载最新版本的 JavaBeans Activation Framework JAF (版本 1.0.2)。
下载并解压缩这些文件,到新创建的顶级目录中。您将在两个应用程序中找到许多 jar 文件。您需要将 **mail.jar** 和 **activation.jar** 文件添加到您的 CLASSPATH 中。
发送简单的电子邮件
这是一个从您的机器发送简单电子邮件的示例。假设您的 **localhost** 连接到 Internet 并且能够发送电子邮件。确保 Java 电子邮件 API 包和 JAF 包中的所有 jar 文件都可以在 CLASSPATH 中找到。
<%@ page import = "java.io.*,java.util.*,javax.mail.*"%> <%@ page import = "javax.mail.internet.*,javax.activation.*"%> <%@ page import = "javax.servlet.http.*,javax.servlet.*" %> <% String result; // Recipient's email ID needs to be mentioned. String to = "abcd@gmail.com"; // Sender's email ID needs to be mentioned String from = "mcmohd@gmail.com"; // Assuming you are sending email from localhost String host = "localhost"; // Get system properties object Properties properties = System.getProperties(); // Setup mail server properties.setProperty("mail.smtp.host", host); // Get the default Session object. Session mailSession = Session.getDefaultInstance(properties); try { // Create a default MimeMessage object. MimeMessage message = new MimeMessage(mailSession); // Set From: header field of the header. message.setFrom(new InternetAddress(from)); // Set To: header field of the header. message.addRecipient(Message.RecipientType.TO, new InternetAddress(to)); // Set Subject: header field message.setSubject("This is the Subject Line!"); // Now set the actual message message.setText("This is actual message"); // Send message Transport.send(message); result = "Sent message successfully...."; } catch (MessagingException mex) { mex.printStackTrace(); result = "Error: unable to send message...."; } %> <html> <head> <title>Send Email using JSP</title> </head> <body> <center> <h1>Send Email using JSP</h1> </center> <p align = "center"> <% out.println("Result: " + result + "\n"); %> </p> </body> </html>
现在让我们将上述代码放入 **SendEmail.jsp** 文件中,并使用 URL **https://127.0.0.1:8080/SendEmail.jsp** 调用此 JSP。这将帮助您向给定的电子邮件 ID **abcd@gmail.com** 发送电子邮件。您将收到以下响应 -
Send Email using JSP
Result: Sent message successfully....
如果要向多个收件人发送电子邮件,请使用以下方法指定多个电子邮件 ID -
void addRecipients(Message.RecipientType type, Address[] addresses) throws MessagingException
以下是参数的描述 -
**type** - 这将设置为 TO、CC 或 BCC。其中 CC 代表抄送,BCC 代表密送。例如 Message.RecipientType.TO
**addresses** - 这是电子邮件 ID 的数组。在指定电子邮件 ID 时,您需要使用 InternetAddress() 方法
发送 HTML 电子邮件
这是一个从您的机器发送 HTML 电子邮件的示例。假设您的 **localhost** 连接到 Internet 并且能够发送电子邮件。确保 Java 电子邮件 API 包和 JAF 包中的所有 jar 文件都可以在 CLASSPATH 中找到。
此示例与上一个示例非常相似,只是这里我们使用 **setContent()** 方法来设置内容,其第二个参数是 **"text/html"**,以指定 HTML 内容包含在消息中。
使用此示例,您可以发送任意大小的 HTML 内容。
<%@ page import = "java.io.*,java.util.*,javax.mail.*"%> <%@ page import = "javax.mail.internet.*,javax.activation.*"%> <%@ page import = "javax.servlet.http.*,javax.servlet.*" %> <% String result; // Recipient's email ID needs to be mentioned. String to = "abcd@gmail.com"; // Sender's email ID needs to be mentioned String from = "mcmohd@gmail.com"; // Assuming you are sending email from localhost String host = "localhost"; // Get system properties object Properties properties = System.getProperties(); // Setup mail server properties.setProperty("mail.smtp.host", host); // Get the default Session object. Session mailSession = Session.getDefaultInstance(properties); try { // Create a default MimeMessage object. MimeMessage message = new MimeMessage(mailSession); // Set From: header field of the header. message.setFrom(new InternetAddress(from)); // Set To: header field of the header. message.addRecipient(Message.RecipientType.TO, new InternetAddress(to)); // Set Subject: header field message.setSubject("This is the Subject Line!"); // Send the actual HTML message, as big as you like message.setContent("<h1>This is actual message</h1>", "text/html" ); // Send message Transport.send(message); result = "Sent message successfully...."; } catch (MessagingException mex) { mex.printStackTrace(); result = "Error: unable to send message...."; } %> <html> <head> <title>Send HTML Email using JSP</title> </head> <body> <center> <h1>Send Email using JSP</h1> </center> <p align = "center"> <% out.println("Result: " + result + "\n"); %> </p> </body> </html>
现在让我们使用上面的 JSP 向给定的电子邮件 ID 发送 HTML 消息。
在电子邮件中发送附件
以下是从您的机器发送带有附件的电子邮件的示例 -
<%@ page import = "java.io.*,java.util.*,javax.mail.*"%> <%@ page import = "javax.mail.internet.*,javax.activation.*"%> <%@ page import = "javax.servlet.http.*,javax.servlet.*" %> <% String result; // Recipient's email ID needs to be mentioned. String to = "abcd@gmail.com"; // Sender's email ID needs to be mentioned String from = "mcmohd@gmail.com"; // Assuming you are sending email from localhost String host = "localhost"; // Get system properties object Properties properties = System.getProperties(); // Setup mail server properties.setProperty("mail.smtp.host", host); // Get the default Session object. Session mailSession = Session.getDefaultInstance(properties); try { // Create a default MimeMessage object. MimeMessage message = new MimeMessage(mailSession); // Set From: header field of the header. message.setFrom(new InternetAddress(from)); // Set To: header field of the header. message.addRecipient(Message.RecipientType.TO, new InternetAddress(to)); // Set Subject: header field message.setSubject("This is the Subject Line!"); // Create the message part BodyPart messageBodyPart = new MimeBodyPart(); // Fill the message messageBodyPart.setText("This is message body"); // Create a multipart message Multipart multipart = new MimeMultipart(); // Set text message part multipart.addBodyPart(messageBodyPart); // Part two is attachment messageBodyPart = new MimeBodyPart(); String filename = "file.txt"; DataSource source = new FileDataSource(filename); messageBodyPart.setDataHandler(new DataHandler(source)); messageBodyPart.setFileName(filename); multipart.addBodyPart(messageBodyPart); // Send the complete message parts message.setContent(multipart ); // Send message Transport.send(message); String title = "Send Email"; result = "Sent message successfully...."; } catch (MessagingException mex) { mex.printStackTrace(); result = "Error: unable to send message...."; } %> <html> <head> <title>Send Attachment Email using JSP</title> </head> <body> <center> <h1>Send Attachment Email using JSP</h1> </center> <p align = "center"> <%out.println("Result: " + result + "\n");%> </p> </body> </html>
现在让我们运行上面的 JSP,将文件作为附件连同消息一起发送到给定的电子邮件 ID。
用户身份验证部分
如果需要向电子邮件服务器提供用户 ID 和密码以进行身份验证,则可以按如下方式设置这些属性 -
props.setProperty("mail.user", "myuser"); props.setProperty("mail.password", "mypwd");
其余的电子邮件发送机制将与上面解释的相同。
使用表单发送电子邮件
您可以使用 HTML 表单接受电子邮件参数,然后可以使用 **request** 对象获取所有信息,如下所示 -
String to = request.getParameter("to"); String from = request.getParameter("from"); String subject = request.getParameter("subject"); String messageText = request.getParameter("body");
获取所有信息后,您可以使用上面提到的程序发送电子邮件。